How to Create Pandas Dataframe From a List
- Use Single List to Create Pandas DataFrame
- Use Multiple Lists to Create Pandas DataFrame
- Use Multi-Dimensional List to Create Pandas DataFrame
- Create DataFrame from a Dictionary of Lists
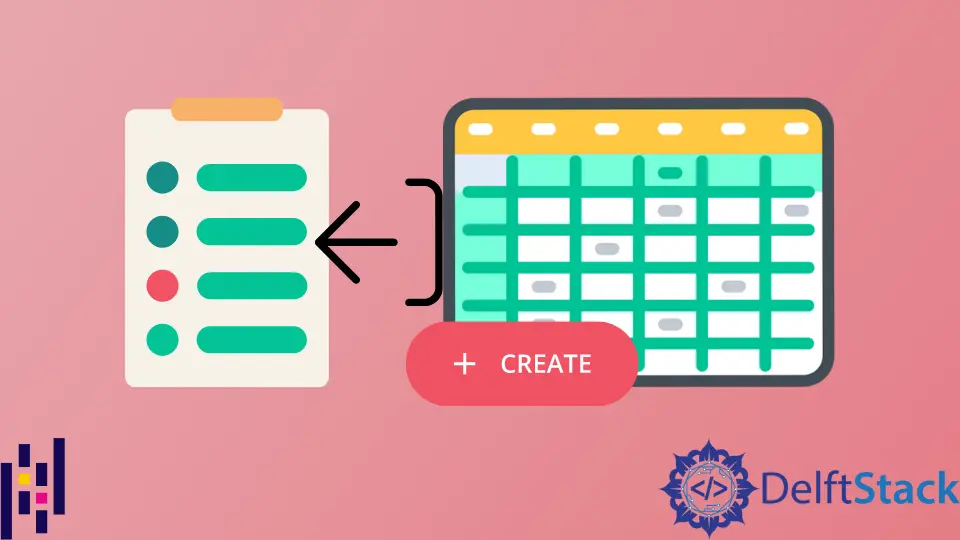
Pandas allow us to create Pandas DataFrame from a list using the pd.DataFrame()
method. We can achieve this using a single list, multiple lists, and multi-dimensional lists.
Use Single List to Create Pandas DataFrame
It is the most basic case which creates a Dataframe out of a single list. We simply pass the list to pd.DataFrame()
and the result is a single column Dataframe.
Example:
import pandas as pd
import numpy as np
lst = ["Jay", "Raj", "Jack"]
df = pd.DataFrame(lst, columns=["Name"])
print(df)
Output:
Name
0 Jay
1 Raj
2 Jack
Use Multiple Lists to Create Pandas DataFrame
For creating a Pandas DataFrame from more than one list, we have to use the zip()
function. The zip()
function returns an object of zip
type which pairs the elements at first position together, at second position together, and so on. Here each list acts as a different column.
Example:
import pandas as pd
import numpy as np
lst1 = ["Jay", "Raj", "Jack"]
lst2 = [12, 15, 14]
df = pd.DataFrame(list(zip(lst1, lst2)), columns=["Name", "Age"])
print(df)
Output:
Name Age
0 Jay 12
1 Raj 15
2 Jack 14
Use Multi-Dimensional List to Create Pandas DataFrame
A list that contains another list is called a multi-dimensional list. In this case, each list, which is nested inside the main list, acts as a row for the dataframe. The following example will show how:
import pandas as pd
import numpy as np
lst = [["Jay", 12, "BBA"], ["Jack", 15, "BSc"]]
df = pd.DataFrame(lst, columns=["Name", "Age", "Course"])
print(df)
Output:
Name Age Course
0 Jay 12 BBA
1 Jack 15 BSc
Create DataFrame from a Dictionary of Lists
import pandas as pd
track_no = [1, 2, 3, 4, 5]
songs_list = ["Circles", "Rockstar", "SunFlower", "Better Now", "Congratulations"]
songs_df = pd.DataFrame({"Track No": track_no, "Song Name": songs_list})
print(songs_df)
Output:
Track No Song Name
0 1 Circles
1 2 Rockstar
2 3 SunFlower
3 4 Better Now
4 5 Congratulations
It creates a DataFrame from the dictionary of lists whose key represents the column name and values represent the column’s values with column name specified by the key.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn