How to Count Unique Values in Pandas
Suraj Joshi
Feb 02, 2024
-
Count Unique Values in a DataFrame Using
Series.value_counts()
-
Count Unique Values in a DataFrame Using
DataFrame.nunique()
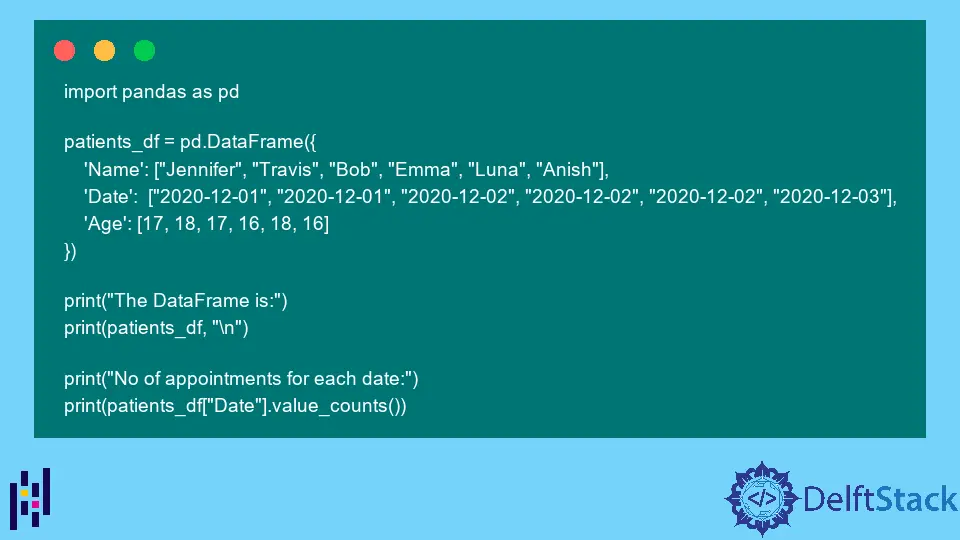
This tutorial explains how we can get count of all the unique values in a DataFrame using Series.value_counts()
and DataFrame.nunique()
methods.
import pandas as pd
patients_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Date": [
"2020-12-01",
"2020-12-01",
"2020-12-02",
"2020-12-02",
"2020-12-02",
"2020-12-03",
],
"Age": [17, 18, 17, 16, 18, 16],
}
)
print(patients_df)
Output:
Name Date Age
0 Jennifer 2020-12-01 17
1 Travis 2020-12-01 18
2 Bob 2020-12-02 17
3 Emma 2020-12-02 16
4 Luna 2020-12-02 18
5 Anish 2020-12-03 16
We will use the DataFrame patients_df
, which contains patients’ names, their appointment date, and age, to explain how we can get the count of all the unique values in a DataFrame.
Count Unique Values in a DataFrame Using Series.value_counts()
import pandas as pd
patients_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Date": [
"2020-12-01",
"2020-12-01",
"2020-12-02",
"2020-12-02",
"2020-12-02",
"2020-12-03",
],
"Age": [17, 18, 17, 16, 18, 16],
}
)
print("The DataFrame is:")
print(patients_df, "\n")
print("No of appointments for each date:")
print(patients_df["Date"].value_counts())
Output:
The DataFrame is:
Name Date Age
0 Jennifer 2020-12-01 17
1 Travis 2020-12-01 18
2 Bob 2020-12-02 17
3 Emma 2020-12-02 16
4 Luna 2020-12-02 18
5 Anish 2020-12-03 16
No of appointments for each date:
2020-12-02 3
2020-12-01 2
2020-12-03 1
Name: Date, dtype: int64
It displays the count of each unique value of the Date
column in the DataFrame.
Count Unique Values in a DataFrame Using DataFrame.nunique()
import pandas as pd
patients_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Date": [
"2020-12-01",
"2020-12-01",
"2020-12-02",
"2020-12-02",
"2020-12-02",
"2020-12-03",
],
"Age": [17, 18, 17, 16, 18, 16],
}
)
print(patients_df, "\n")
print(patients_df.groupby("Date").Name.nunique())
Output:
Name Date Age
0 Jennifer 2020-12-01 17
1 Travis 2020-12-01 18
2 Bob 2020-12-02 17
3 Emma 2020-12-02 16
4 Luna 2020-12-02 18
5 Anish 2020-12-03 16
Date
2020-12-01 2
2020-12-02 3
2020-12-03 1
Name: Name, dtype: int64
It splits the DataFrame based on the value of the Date
column i.e., rows with the same value of Date
are placed in the same group and then counts the occurrence of each name in a particular group to know the count of each unique value of Date
column in the DataFrame.
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn