How to Convert Timedelta to Int in Pandas
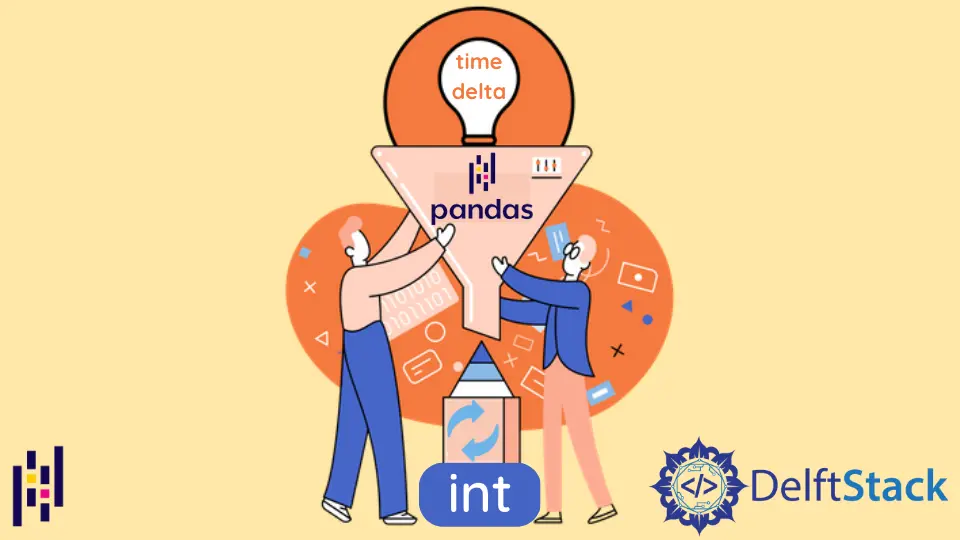
This tutorial will discuss converting the timedelta
to int
using the dt
attribute in Pandas.
Convert the timedelta
to int
Using the dt
Attribute in Pandas
To convert the timedelta
to an integer value, we can use the pandas
library’s dt
attribute. The dt
attribute allows us to extract components of the timedelta
. For example, we can extract the year, month, day, minutes, or seconds using the dt
attribute. To do that, we need to write the component’s name after the dt
attribute. To show all the components of the timedelta
variable, we can use the components
attribute. For example, let’s create a time series using the pandas Series
attribute and show its components using the components
attribute.
import pandas as pd
time_series = pd.Series(pd.timedelta_range(start="1 days", end="10 days", freq="1500T"))
time_series.dt.components
Output:
As you can see, the components
attribute showed all the components of the time series. The timedelta_range()
attribute is used in the above code to create the time series. We can define the starting and ending point and the frequency at which the time will change inside the timedelta_range()
attribute. We can extract any of these components using the name of that component. For example, let’s extract the days component from the above time series. See the code below.
import pandas as pd
time_series = pd.Series(pd.timedelta_range(start="1 days", end="10 days", freq="1500T"))
time_series.dt.days
Output:
0 1
1 2
2 3
3 4
4 5
5 6
6 7
7 8
8 9
dtype: int64
You can extract any component you want from the above time series. We can also convert the timedelta
to an integer by dividing it by the timedelta
of one day or by extracting its integer part using the astype()
attribute. For example, let’s create a timedelta
object and convert it into an integer to get the value of the day using NumPy
. See the code below.
import numpy as np
x = np.timedelta64(2058311000000000, "ns")
day = x.astype("timedelta64[D]")
days.astype(int)
Output:
23.0
The timedelta
is actually in the int64
data type, and we can extract our desired component by converting it into an int
using the astype()
attribute. We can also convert the timedelta
to hours or seconds or any other component using the same method. To do that, we need to change D
in the third line of code to h
for hours and s
for seconds, and so on.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn