How to Convert Pandas DataFrame Column to List
-
Use the
tolist()
Method to Convert a Dataframe Column to a List -
Use the
list()
Function to Convert a Dataframe Column to a List -
Use the
.values
Property to Convert a Dataframe Column to a List - Use List Comprehension to Convert a Dataframe Column to a List
- Conclusion
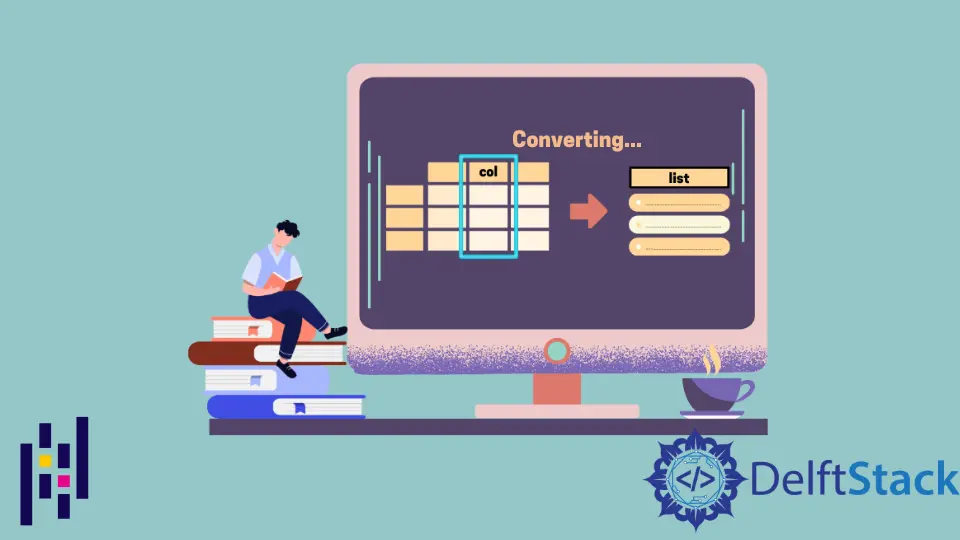
When working with Pandas DataFrames in Python, you might often need to convert a column of your DataFrame into a Python list. This process can be crucial for various data manipulation and analysis tasks. Fortunately, Pandas provides several methods to achieve this, making it easy to extract the data you need in list form.
In this article, we’ll explore different methods to convert a Pandas DataFrame column into a Python list. We’ll demonstrate these methods using a sample DataFrame that contains information about names, dates of birth (DOB), and salaries.
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": ["1000", "12000", "36000", "15000", "12000"],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
We’ll explore four different methods to perform this conversion: using the tolist()
method, the list()
function, the .values
property, and list comprehension. Each method has its advantages, so let’s dive in.
Use the tolist()
Method to Convert a Dataframe Column to a List
A column in the Pandas dataframe is a Pandas Series
. So if we need to convert a column to a list, we can use tolist()
method in the Series
. tolist()
converts the Series
of pandas data-frame to a list.
In the code below, df['DOB']
returns the Series
, or the column, with the name as DOB
from the DataFrame.
The tolist()
method converts the Series
to a list.
import pandas as pd
df = pd.DataFrame(
[
["James", "1/1/2014", "1000"],
["Michelina", "2/1/2014", "12000"],
["Marc", "3/1/2014", "36000"],
["Bob", "4/1/2014", "15000"],
["Halena", "4/1/2014", "12000"],
],
columns=["Name", "DOB", "Salary"],
)
print("Pandas DataFrame:\n\n", df, "\n")
list_of_single_column = df["DOB"].tolist()
print(
"the list of a single column from the dataframe\n",
list_of_single_column,
"\n",
type(list_of_single_column),
)
Output:
Pandas DataFrame:
Name DOB Salary
0 James 1/1/2014 1000
1 Michelina 2/1/2014 12000
2 Marc 3/1/2014 36000
3 Bob 4/1/2014 15000
4 Halena 4/1/2014 12000
the list of a single column from the dataframe
['1/1/2014', '2/1/2014', '3/1/2014', '4/1/2014', '4/1/2014']
<class 'list'>
Use the list()
Function to Convert a Dataframe Column to a List
We can also use the list()
function to convert a DataFrame column to a list, by passing the DataFrame to the list()
function.
We will use the same data as above to demonstrate this approach.
import pandas as pd
df = pd.DataFrame(
[
["James", "1/1/2014", "1000"],
["Michelina", "2/1/2014", "12000"],
["Marc", "3/1/2014", "36000"],
["Bob", "4/1/2014", "15000"],
["Halena", "4/1/2014", "12000"],
],
columns=["Name", "DOB", "Salary"],
)
print("Pandas DataFrame:\n\n", df, "\n")
list_of_single_column = list(df["DOB"])
print(
"the list of a single column from the dataframe\n",
list_of_single_column,
"\n",
type(list_of_single_column),
)
Output:
Pandas DataFrame:
Name DOB Salary
0 James 1/1/2014 1000
1 Michelina 2/1/2014 12000
2 Marc 3/1/2014 36000
3 Bob 4/1/2014 15000
4 Halena 4/1/2014 12000
the list of a single column from the dataframe
['1/1/2014', '2/1/2014', '3/1/2014', '4/1/2014', '4/1/2014']
<class 'list'>
Use the .values
Property to Convert a Dataframe Column to a List
Another way to achieve this is by using the .values
property.
Let’s go through the steps of converting the ‘Salary’ column of this DataFrame into a Python list using the .values
property.
The .values
property of a Pandas Series returns a NumPy array representation of the data. To convert it into a Python list, you can use the .tolist()
method. Here’s how you do it:
salary_list = df["Salary"].values.tolist()
Here’s the complete code:
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": ["1000", "12000", "36000", "15000", "12000"],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
# Convert the 'Salary' column to a list using .values
salary_list = df["Salary"].values.tolist()
print(salary_list)
Output:
['1000', '12000', '36000', '15000', '12000']
After executing this code, the Salary
column from the DataFrame will be converted into a Python list, which is stored in the salary_list
variable.
Use List Comprehension to Convert a Dataframe Column to a List
List comprehension is a concise and efficient way to create lists in Python. To convert a column of a Pandas DataFrame into a Python list using list comprehension, you can follow the below code.
# Using list comprehension to convert the 'Salary' column to a list
salary_list = [salary for salary in df["Salary"]]
Here’s the complete code:
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": [1000, 12000, 36000, 15000, 12000],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
# Using list comprehension to convert the 'Salary' column to a list
salary_list = [salary for salary in df["Salary"]]
print(salary_list)
Output:
[1000, 12000, 36000, 15000, 12000]
Conclusion
In this article, we explored four different methods to convert a Pandas DataFrame column into a Python list: using the tolist()
method, the list()
function, the .values
property, and list comprehension. Each method has its advantages and can be chosen based on your specific use case and coding style.
Whether you prefer the simplicity of the tolist()
method, the standard Pythonic approach of the list()
function, the efficiency of the .values
property, or the readability of list comprehension, Pandas offers multiple options to help you seamlessly convert your DataFrame columns into Python lists.
Related Article - Pandas DataFrame Column
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Get the Sum of Pandas Column
- How to Change the Order of Pandas DataFrame Columns
- How to Convert DataFrame Column to String in Pandas