How to Append a Column in Pandas DataFrame
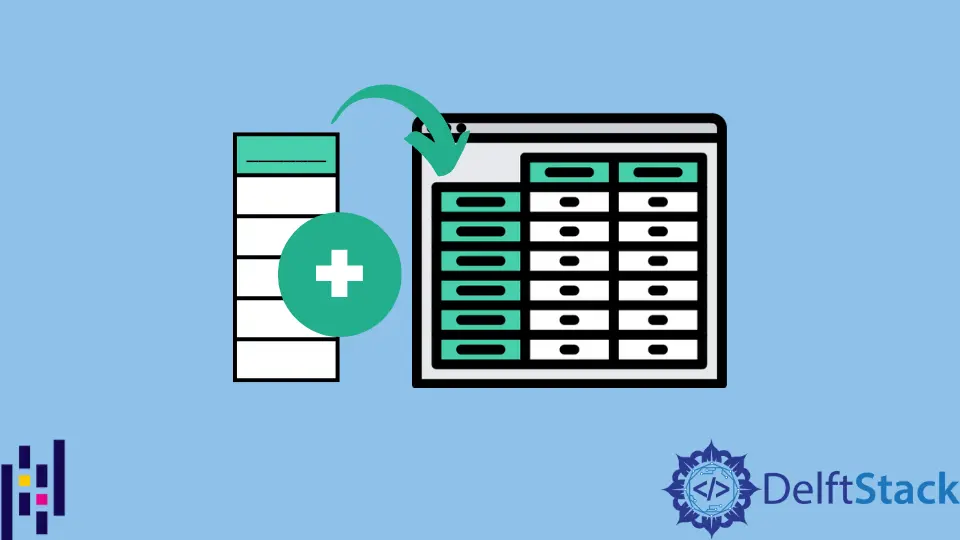
In this tutorial, you will learn to add a particular column to a Pandas data frame.
Before we begin, we create a dummy data frame to work with. Here we make two data frames, namely, dat1
and dat2
, along with a few entries.
import pandas as pd
dat1 = pd.DataFrame({"dat1": [9, 5]})
print(dat1)
Output:
dat1
0 9
1 5
Now, let us create another data frame named dat2
. We can do this using the following code.
dat2 = pd.DataFrame({"dat2": [7, 6]})
print(dat2)
Output:
dat2
0 7
1 6
As we can see for both dat1
and dat2
, we have 2 columns and 2 rows where one indicates the index and the second shows the values in our data frame.
Use concat()
to Append a Column in Pandas
We can use the concat
function in Pandas to merge or concatenate multiple data frames into one with the help of a single argument that is passed as an array with all the data frames to be combined.
We need to assign the axis of the addition of the data frame to alter the data frame in terms of columns or rows.
Now, let us try to merge dat2
to dat1
data frame. We use the following code:
dat1 = pd.concat([dat1, dat2], axis=1)
Output:
dat1 dat2
0 9 7
1 5 6
As evident from the code, we use the axis parameter with a value of 1. The axis parameter indicates that we want to add a column to the array’s data frame assigned in the first argument.
In the output, dat1
has been altered such that an additional column has been added in the first axis.
Use join()
to Append a Column in Pandas
Pandas assists us with another function called the join
function. This function helps join two different data frames, thereby helping us to add a particular column to a specific data frame.
We can merge dat1
and dat2
with the help of this function.
val = dat1.join(dat2)
print(val)
Output:
dat1 dat2
0 9 7
1 5 6
As we can see, we have the expected result. Notably, we have added a new column to the dat1
data frame with the help of the join
function in Pandas.
With the help of the join
function and concat
function in Pandas, we can efficiently filter data based on our requirement as and when needed and add a particular column or a group of columns to a specific data set.