How to Fix Error - Module Pandas Has No Attribute Dataframe Error
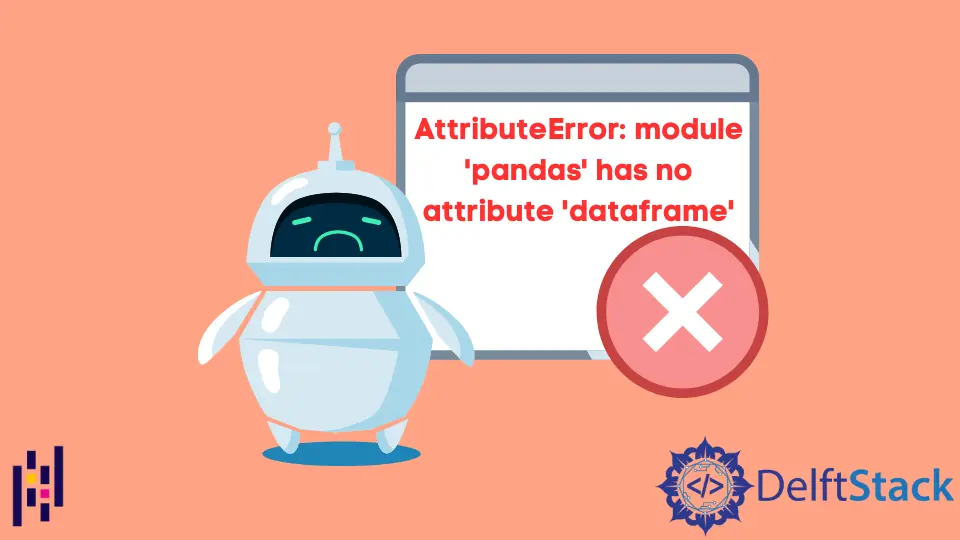
This tutorial will teach us how to fix the module 'pandas' has no attribute 'dataframe'
error.
Fix module 'pandas' has no attribute 'dataframe'
Error in Pandas
We must use DataFrame()
to generate a dataframe. Because there is no dataframe attribute in Pandas, using the dataframe will result in an error.
DataFrame()
is the way. Any dictionary can be used as an argument.
We can pass the dictionary as an argument because it has a key and value pair.
Keys are treated as attributes in Dataframe, and pairs are treated as tuples. Let’s look at why we receive issues when making a dataframe.
Using the function dataframe()
, let us create a data frame.
But before that, we need to import Pandas to get started.
import pandas as pd
Now that we have imported Pandas let us create the data frame.
data = {"id": [1, 2, 3], "name": ["shivesh", "sanay", "rutwik"]}
df = pd.dataframe(data)
Now let us see if or not we get an error while creating the data frame using dataframe()
and if we get what exactly it is.
File "c:/Users/Shivesh/Debugging/new_debug.py", line 45, in <module>
df = pd.dataframe(data)
File "C:\Users\Shivesh\AppData\Local\Programs\Python\Python38\lib\site-packages\pandas\__init__.py", line 261, in __getattr__
raise AttributeError(f"module 'pandas' has no attribute '{name}'")
AttributeError: module 'pandas' has no attribute 'dataframe'
We get the above error while creation which mentions that module 'pandas' has no attribute 'dataframe'
. So now, we will try creating using the DataFrame()
method.
df = pd.DataFrame(data)
print(df)
Let us see the output to ensure that the data frame was successfully created.
id name
0 1 shivesh
1 2 sanay
2 3 rutwik
We can see above that our data frame was successfully created when we created it using the DataFrame()
method.
Hence, we can fix the error module 'pandas' has no attribute 'dataframe'
in Pandas.