How to Load Data From Text File in Pandas
-
read_csv()
Method to Load Data From Text File -
read_fwf()
Method to Load Width-Formated Text File to PandasDataFrame
-
read_table()
Method to Load Text File to PandasDataFrame
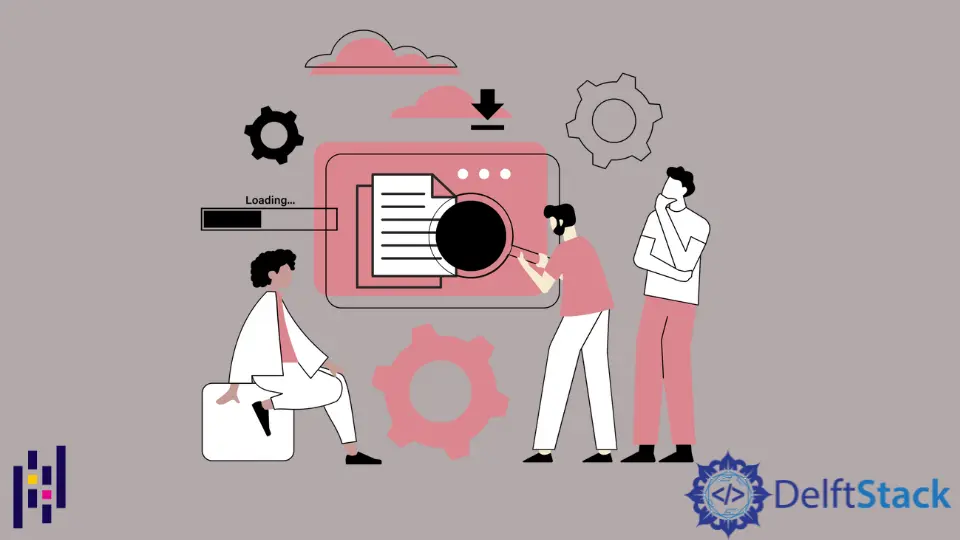
We will introduce the methods to load the data from a txt
file with Pandas DataFrame
. We will also go through the available options.
First, we will create a simple text file called sample.txt
and add the following lines to the file:
45 apple orange banana mango
12 orange kiwi onion tomato
We need to save it to the same directory from where Python script will be running.
read_csv()
Method to Load Data From Text File
read_csv()
is the best way to convert the text
file into Pandas DataFrame
. We need to set header=None
as we don’t have any header
in the above-created file. We can also set keep_default_na=False
inside the method if we wish to replace empty values with NaN
.
Example Codes:
# python 3.x
import pandas as pd
df = pd.read_csv("sample.txt", sep=" ", header=None)
print(df)
Output:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi onion tomato
We set sep=" "
because a single white space separates values. Similarly, we can set sep=","
if we read data from a comma-separated file. Replace the white spaces inside sample.txt
with ,
and then run the code after replacing sep=" "
with sep=","
.
Sample.txt
45,apple,orange,banana,mango
12,orange,kiwi,,tomato
Code:
# python 3.x
import pandas as pd
df = pd.read_csv("sample.txt", sep=",", header=None)
print(df)
Output:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi NaN tomato
read_fwf()
Method to Load Width-Formated Text File to Pandas DataFrame
read_fwf()
is very helpful to load a width-formatted text file. We can’t use sep
because different values may have different delimiters. Consider the following text file:
Sample.txt
45 apple orange banana mango
12 orange kiwi onion tomato
In Sample.text
, delimiter
is not the same for all values. So read_fwf()
will do the job here.
Code:
# python 3.x
import pandas as pd
df = pd.read_fwf("sample.txt", header=None)
print(df)
Output:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi onion tomato
read_table()
Method to Load Text File to Pandas DataFrame
read_table()
is another approach to load data from text
file to Pandas DataFrame
.
Sample.txt:
45 apple orange banana mango
12 orange kiwi onion tomato
Code:
# python 3.x
import pandas as pd
df = pd.read_table("sample.txt", header=None, sep=" ")
print(df)
Output:
0 1 2 3 4
0 45 apple orange banana mango
1 12 orange kiwi onion tomato