How to Get the Row Count of a Pandas DataFrame
- Using the shape Attribute
-
Using the
len()
Function - Counting Rows That Satisfy a Condition
- Conclusion
- FAQ
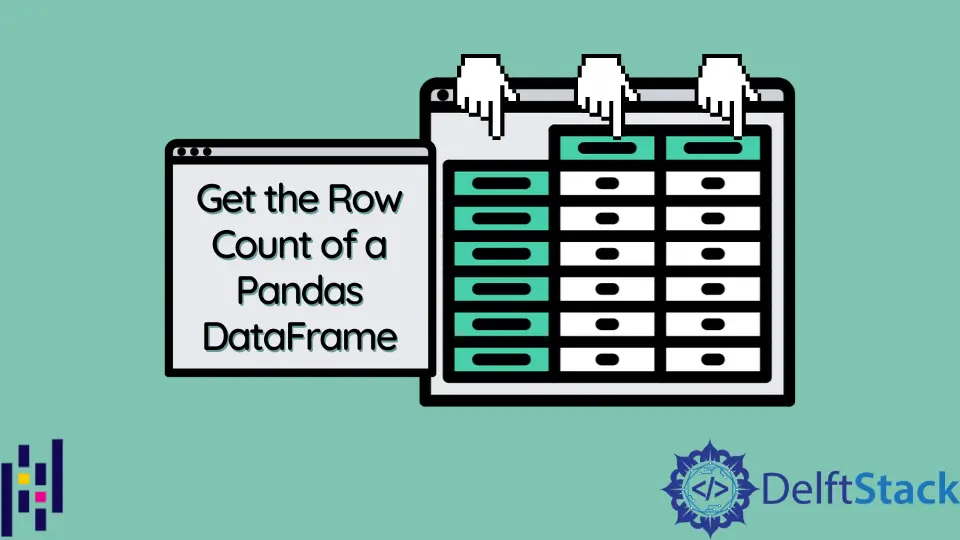
When working with data in Python, particularly using the Pandas library, knowing how to get the row count of a DataFrame is fundamental. Whether you’re performing data analysis, cleaning datasets, or preparing data for machine learning, understanding the size of your DataFrame can provide valuable insights.
In this tutorial, we’ll explore different methods to retrieve the row count of a Pandas DataFrame, including using the shape
attribute, the len()
function, and filtering to count rows that meet specific conditions. By the end of this guide, you’ll have a solid grasp of how to efficiently determine the number of rows in your DataFrame.
Using the shape Attribute
One of the simplest and most commonly used methods to get the row count of a Pandas DataFrame is by utilizing the shape
attribute. This attribute returns a tuple representing the dimensions of the DataFrame, where the first element corresponds to the number of rows and the second element corresponds to the number of columns.
Here’s how you can use the shape
attribute:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
row_count = df.shape[0]
print(row_count)
Output:
3
The shape
attribute is particularly useful because it provides both row and column counts in a single call. By accessing the first element of the tuple (df.shape[0]
), you can quickly retrieve the number of rows. This method is efficient and straightforward, making it a favorite among data analysts and scientists. Additionally, since shape
is an attribute, it doesn’t require function calls, which can slightly enhance performance in scenarios where speed is critical.
Using the len()
Function
Another straightforward approach to get the row count of a Pandas DataFrame is by using the built-in len()
function. This function returns the total number of items in an object. When applied to a DataFrame, it returns the number of rows.
Here’s how you can use len()
to find the row count:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
row_count = len(df)
print(row_count)
Output:
3
Using len()
is another intuitive method for counting rows. It’s simple and effective, especially for those who are familiar with Python’s built-in functions. This approach is often preferred for its readability; it clearly conveys the intention of counting the number of rows without delving into attributes. However, it’s worth mentioning that while len()
is easy to use, it only provides the row count and does not give any information about the columns, unlike the shape
attribute.
Counting Rows That Satisfy a Condition
Sometimes, you may want to count only the rows that satisfy a specific condition. For instance, if you want to know how many individuals in a DataFrame are above a certain age, you can use boolean indexing combined with the sum()
function. This method allows for more granular control over your data analysis.
Here’s an example of how to count rows based on a condition:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
condition = df['Age'] > 28
row_count = condition.sum()
print(row_count)
Output:
2
In this code snippet, we first create a boolean Series by checking which rows meet the condition (df['Age'] > 28
). The resulting Series contains True
for rows where the condition is met and False
otherwise. By applying the sum()
function to this Series, we effectively count the number of True
values, which correspond to the rows that satisfy the condition. This method is powerful for data filtering and analysis, allowing you to derive meaningful insights from your datasets.
Conclusion
In conclusion, knowing how to get the row count of a Pandas DataFrame is a crucial skill for anyone working with data in Python. Whether you choose to use the shape
attribute, the len()
function, or boolean indexing to count rows that meet specific conditions, each method offers its own advantages. By mastering these techniques, you can enhance your data analysis capabilities and make more informed decisions based on your datasets. So, the next time you’re working with a DataFrame, you’ll be well-equipped to determine its size and understand your data better.
FAQ
-
How can I get both row and column counts in a Pandas DataFrame?
You can use the shape attribute, which returns a tuple of (rows, columns). For example, df.shape will give you the dimensions. -
Is there a way to count rows based on multiple conditions?
Yes, you can combine conditions using logical operators (like & for AND, | for OR) and then apply the sum() function on the resulting boolean Series. -
Can I count rows in a DataFrame that contains missing values?
Yes, you can use the dropna() method before counting to exclude rows with missing values or use conditions that account for NaNs. -
What is the difference between using shape and len()?
The shape attribute provides both row and column counts, while len() only returns the number of rows. -
How can I count unique rows in a DataFrame?
You can use the drop_duplicates() method followed by len() to count unique rows, like this: len(df.drop_duplicates()).
Related Article - Pandas DataFrame Row
- How to Randomly Shuffle DataFrame Rows in Pandas
- How to Filter Dataframe Rows Based on Column Values in Pandas
- How to Iterate Through Rows of a DataFrame in Pandas
- How to Get Index of All Rows Whose Particular Column Satisfies Given Condition in Pandas
- How to Find Duplicate Rows in a DataFrame Using Pandas