How to Delete Pandas DataFrame Column
-
del
Method to Delete DataFrame Column -
df.drop
Method to Delete DataFrame Columns -
df.pop
Method to Delete DataFrame Column in Pandas
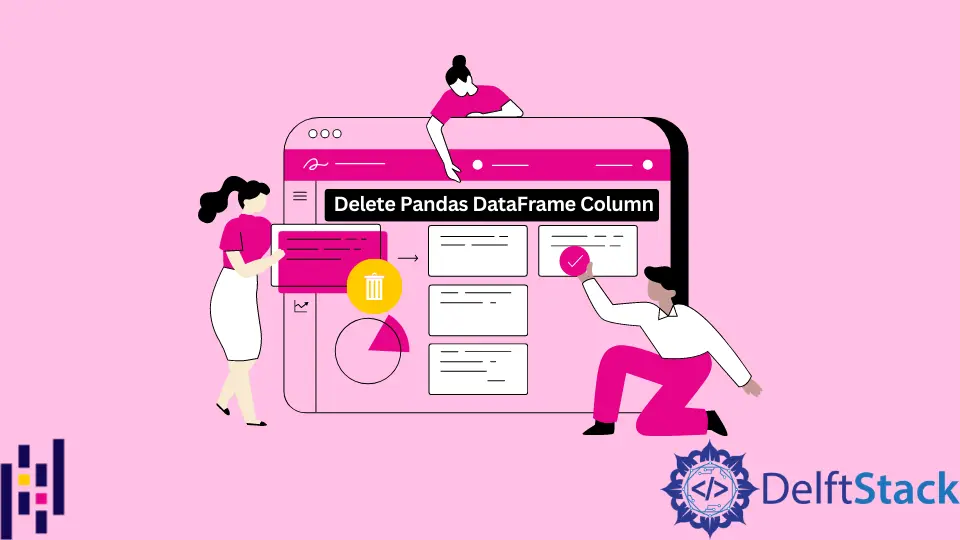
This article introduces different methods to delete DataFrame
columns in Pandas,
del
methoddf.drop
methoddf.pop
method
We will use the same DataFrame
to demonstrate different methods in this article.
>>> from pandas import DataFrame
>>> df = DataFrame.from_items(
[('Alfa', [1, 2, 3]),
('Bravo', [4, 5, 6]),
('Charlie', [7,8, 9])],
orient='index',
columns=['A', 'B', 'C'])
>>> df
A B C
Alfa 1 2 3
Bravo 4 5 6
Charlie 7 8 9
del
Method to Delete DataFrame Column
>>> df
A B C
Alfa 1 2 3
Bravo 4 5 6
Charlie 7 8 9
>>> del df['A']
>>> df
B C
Alfa 2 3
Bravo 5 6
Charlie 8 9
del df['col_name']
deletes the DataFrame column that has the names as col_name
.
The limitation of this del
method is that it could only delete one column at one time.
df.drop
Method to Delete DataFrame Columns
drop(
self,
labels=None,
axis=0,
index=None,
columns=None,
level=None,
inplace=False,
errors="raise",
)
drop
method drops, or in other words removes/deletes the specified labels
from rows or columns.
labels
could be a single label or list-like index or column labels to drop.
axis
specifies whether the labels are dropped from the index/row (0
or index
) or column (1
or columns
).
index
, columns
is the alternative to specifying axis. drop(labels, axis=0)
is equal to drop(index=labels)
, meanwhile drop(labels, axis=1)
is equal to drop(column=labels)
.
inplace
specifies the DataFrame is modified in place if inplace = True
, otherwise, it returns the new DataFrame with the original DataFrame unmodified.
>>> df
A B C
Alfa 1 2 3
Bravo 4 5 6
Charlie 7 8 9
>>> df.drop(["B", "C"], axis=1)
A
Alfa 1
Bravo 4
Charlie 7
## or equally
>>> df.drop(columns=["B", "C"])
A
Alfa 1
Bravo 4
Charlie 7
## or drop the columns in place
>>> df.drop(columns=["B", "C"],inplace=True)
>>> df
A
Alfa 1
Bravo 4
Charlie 7
df.pop
Method to Delete DataFrame Column in Pandas
df.pop(item)
DataFrame pop
method returns the item and drops it from the DataFrame.
>>> df
A B C
Alfa 1 2 3
Bravo 4 5 6
Charlie 7 8 9
>>> df.pop("A")
Alfa 1
Bravo 4
Charlie 7
Name: A, dtype: int64
>>> df
B C
Alfa 2 3
Bravo 5 6
Charlie 8 9
The DataFrame data is modified in place as shown above.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum