How to Delete a Row Based on Column Value in Pandas DataFrame
-
.drop
Method to Delete Row on Column Value in PandasDataFrame
-
boolean masking
Method to Delete Row in PandasDataFrame
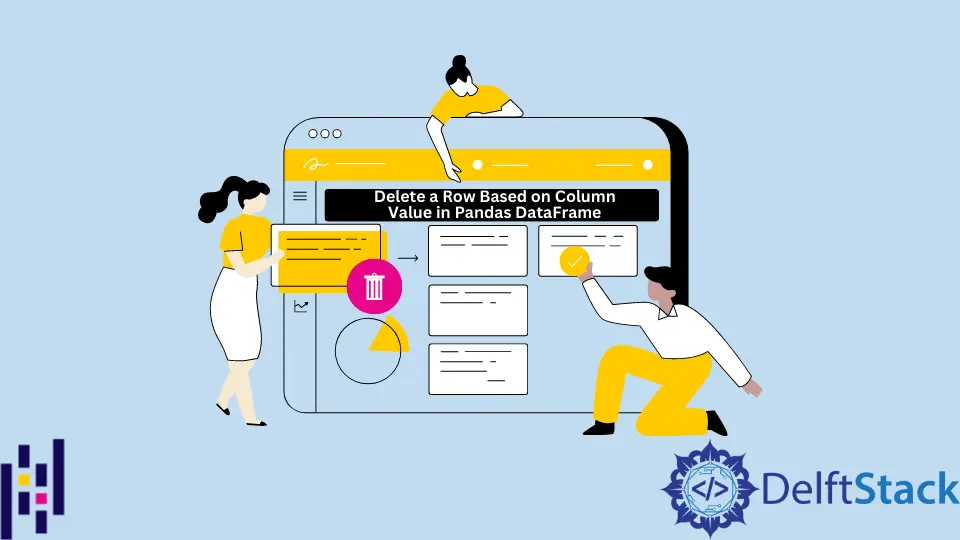
We will introduce methods to delete Pandas DataFrame
rows based on the conditions on column values, by using .drop
(with and without loc
) and boolean masking
.
.drop
Method to Delete Row on Column Value in Pandas DataFrame
.drop
method accepts a single or list of columns’ names and deletes the rows or columns. For rows we set parameter axis=0
and for column we set axis=1
(by default axis
is 0
). We can also get the series of True
and False
based on condition applying on column value in Pandas DataFrame
.
Example Codes:
# python 3.x
import pandas as pd
fruit_list = [
("Orange", 34, "Yes"),
("Mango", 24, "No"),
("banana", 14, "No"),
("Apple", 44, "Yes"),
("Pineapple", 64, "No"),
("Kiwi", 84, "Yes"),
]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
# Get names of indexes for which column Stock has value No
indexNames = df[df["Stock"] == "No"].index
# Delete these row indexes from dataFrame
df.drop(indexNames, inplace=True)
print(df)
Output:
Name Price Stock
0 Orange 34 Yes
3 Apple 44 Yes
5 Kiwi 84 Yes
We can also get a similar result by using .loc
inside df.drop
method.
df.drop(df.loc[df["Stock"] == "Yes"].index, inplace=True)
We can also drop the rows based on multiple column values. In the above example, we can delete rows that have price >= 30 and price <=70.
Example Code:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [
("Orange", 34, "Yes"),
("Mango", 24, "No"),
("banana", 14, "No"),
("Apple", 44, "Yes"),
("Pineapple", 64, "No"),
("Kiwi", 84, "Yes"),
]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
indexNames = df[(df["Price"] >= 30) & (df["Price"] <= 70)].index
df.drop(indexNames, inplace=True)
print(df)
Output:
Name Price Stock
1 Mango 24 No
2 banana 14 No
5 Kiwi 84 Yes
Rows with price > 30 and less < 70 have been deleted.
boolean masking
Method to Delete Row in Pandas DataFrame
boolean masking
is the best and simplest way to delete row in Pandas DataFrame
based on column value.
Example codes:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [
("Orange", 34, "Yes"),
("Mango", 24, "No"),
("banana", 14, "No"),
("Apple", 44, "Yes"),
("Pineapple", 64, "No"),
("Kiwi", 84, "Yes"),
]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
print(df[df.Price > 40])
print("............................")
print(df[(df.Price > 40) & (df.Stock == "Yes")])
Output:
Name Price Stock
3 Apple 44 Yes
4 Pineapple 64 No
5 Kiwi 84 Yes
............................
Name Price Stock
3 Apple 44 Yes
5 Kiwi 84 Yes