How to Convert Index of a Pandas Dataframe Into a Column
Asad Riaz
Feb 02, 2024
-
df.index
to Add Index as a New Column -
reset_index
Withrename_axis
to Rename the Current Index Column Name -
Use the
set_index
Method to Convert Column to Index -
MultiIndex
to Set Multiple Layers ofindexes
oncolumn
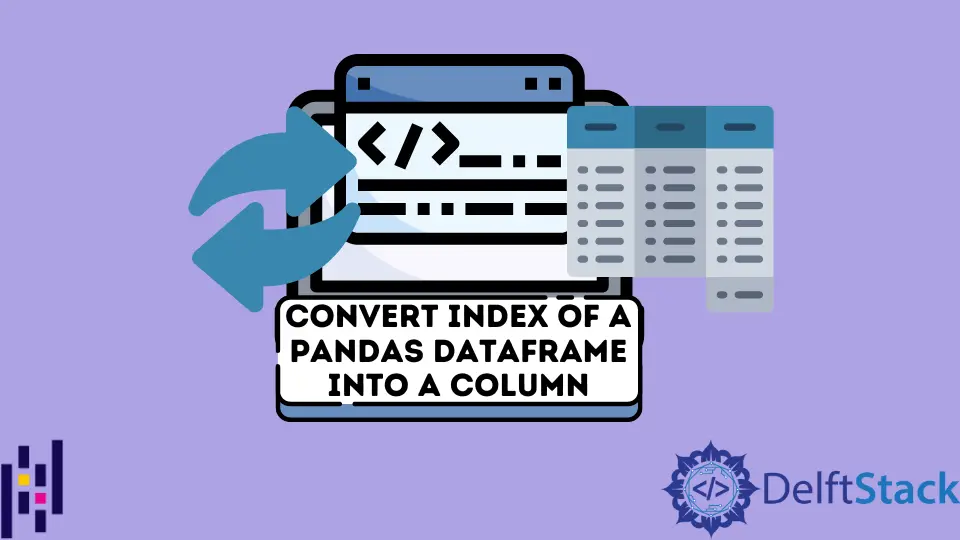
We will introduce various methods to convert the index
of a Pandas DataFrame
into a column, like df.index
, set_index
, and reset_index
with rename_axis
to rename the index
.
We will also introduce how we can apply Multi-Index
to a given DataFrame
with multiple layers of indexes.
df.index
to Add Index as a New Column
The simplest way to add index as the column is by adding df.index
as a new column to DataFrame
.
Example Codes:
# python 3.x
import pandas as pd
df = pd.DataFrame(
[(1, 2, None), (None, 4, None), (5, None, 7), (5, None, None)],
columns=["a", "b", "d"],
)
df["index"] = df.index
print(df)
Output:
a b d index1
0 1.0 2.0 NaN 0
1 NaN 4.0 NaN 1
2 5.0 NaN 7.0 2
3 5.0 NaN NaN 3
reset_index
With rename_axis
to Rename the Current Index Column Name
We can change the name of our index
, then use reset_index
to a series:
# python 3.x
import pandas as pd
df = pd.DataFrame(
[(1, 2, None), (None, 4, None), (5, None, 7), (5, None, None)],
columns=["a", "b", "d"],
)
df = df.rename_axis("index").reset_index()
print(df)
Output:
index a b d
0 0 1.0 2.0 NaN
1 1 NaN 4.0 NaN
2 2 5.0 NaN 7.0
3 3 5.0 NaN NaN
Use the set_index
Method to Convert Column to Index
We can convert any column to index
using the set_index
method.
# python 3.x
import pandas as pd
df = pd.DataFrame(
[(1, 2, None), (None, 4, None), (5, 4, 7), (5, 5, None)], columns=["a", "b", "d"]
)
df.set_index("b", inplace=True)
print(df)
Output:
a d
b
2 1.0 NaN
4 NaN NaN
4 5.0 7.0
5 5.0 NaN
Or if we want to remove the index
name, as in the original, we can do df.index.name = None
:
# python 3.x
import pandas as pd
df = pd.DataFrame(
[(1, 2, None), (None, 4, None), (5, 4, 7), (5, 5, None)], columns=["a", "b", "d"]
)
df.set_index("b", inplace=True)
df.index.name = None
print(df)
Output:
a d
2 1.0 NaN
4 NaN NaN
4 5.0 7.0
5 5.0 NaN
MultiIndex
to Set Multiple Layers of indexes
on column
We can use MultiIndex.from_product()
function to make a MultiIndex as follow:
# python 3.x
import pandas as pd
import numpy as np
index = pd.MultiIndex.from_product(
[["Burger", "Steak", "Sandwich"], ["Half", "Full"]], names=["Item", "Type"]
)
df = pd.DataFrame(
index=index, data=np.random.randint(0, 10, (6, 4)), columns=list("abcd")
)
print(df)
Output:
a b c d
Item Type
Burger Half 0 3 9 1
Full 2 2 0 5
Steak Half 8 4 5 5
Full 5 8 0 7
Sandwich Half 2 8 9 5
Full 4 4 5 9