How to Add One Row to Pandas DataFrame
-
.loc[index]
Method to Add the Row to Pandas Dataframe With Lists - Append Dictionary as the Row to Add It to Pandas Dataframe
-
Dataframe
append()
Method to Add a Row
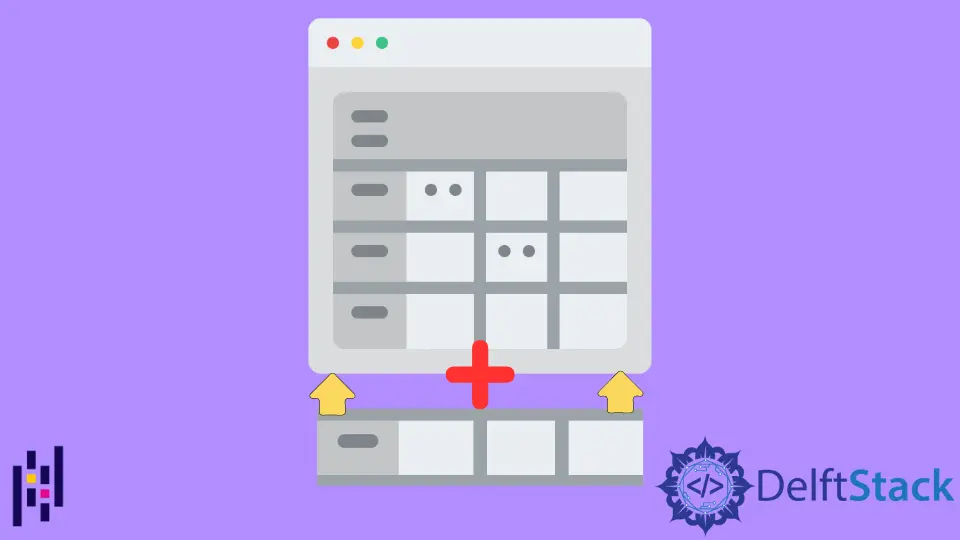
Pandas is designed to load a fully populated DataFrame
. We can add row one by one to pandas.Dataframe
by using various approaches like .loc
, dictionaries
, pandas.concat()
or DataFrame.append()
.
.loc[index]
Method to Add the Row to Pandas Dataframe With Lists
loc[index]
takes the new list as a new row and add it to the given index
of pandas.Dataframe
.
Example Codes:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [("Orange", 34, "Yes")]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
# Add new ROW
df.loc[1] = ["Mango", 4, "No"]
df.loc[2] = ["Apple", 14, "Yes"]
print(df)
Output:
Name Price Stock
0 Orange 34 Yes
1 Mango 4 No
2 Apple 14 Yes
ignore_index
shall be set to be True
when we pass a dictionary to the append()
function. Otherwise, it will raise errors.Append Dictionary as the Row to Add It to Pandas Dataframe
The append()
method can take the dictionary’s value directly as a row and add it to pandas DataFrame
.
Example Codes:
# python 3.x
import pandas as pd
# List of Tuples
fruit_list = [("Orange", 34, "Yes")]
# Create a DataFrame object
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
# Add new ROW
df = df.append({"Name": "Apple", "Price": 23, "Stock": "No"}, ignore_index=True)
df = df.append({"Name": "Mango", "Price": 13, "Stock": "Yes"}, ignore_index=True)
print(df)
Output:
Name Price Stock
0 Orange 34 Yes
1 Apple 23 No
2 Mango 13 Yes
Dataframe append()
Method to Add a Row
append()
method could append rows of other DataFrame
to the end of the original DataFrame
, and return a new DataFrame
. Columns of the new DataFrame
which are not in the original datafarme
are also added to the existing DataFrame
and the new cells’ values are filled with NaN
.
Example Codes:
import pandas as pd
fruit_list = [("Orange", 34, "Yes")]
df = pd.DataFrame(fruit_list, columns=["Name", "Price", "Stock"])
print("Original DataFrame:")
print(df)
print(".............................")
print(".............................")
new_fruit_list = [("Apple", 34, "Yes", "small")]
dfNew = pd.DataFrame(new_fruit_list, columns=["Name", "Price", "Stock", "Type"])
print("Newly Created DataFrame:")
print(dfNew)
print(".............................")
print(".............................")
# append one dataframe to othher
df = df.append(dfNew, ignore_index=True)
print("Copying DataFrame to orignal...")
print(df)
ignore_index=True
will ignore the index
of the new DataFrame
and assign them a new index in the original DataFrame
.
Output:
Original DataFrame:
Name Price Stock
0 Orange 34 Yes
.............................
.............................
Newly Created DataFrame:
Name Price Stock Type
0 Apple 34 Yes small
.............................
.............................
Copying DataFrame to original..:
Name Price Stock Type
0 Orange 34 Yes NaN
1 Apple 34 Yes small