How to Find the Product of Columns in a Pandas DataFrame
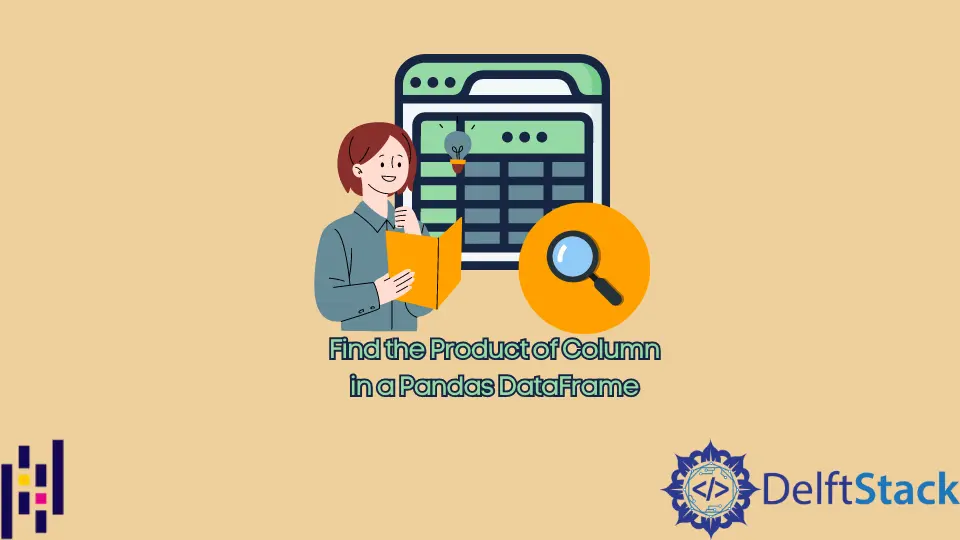
This tutorial demonstrates how to find the product of several columns in a Pandas DataFrame in Python.
The DataFrame is a data structure that is somewhat similar to a table with the rows and columns labeled and can be accessed, created, and manipulated with the Pandas module.
Use the product()
Function to Find the Product of Several Columns in a Pandas DataFrame in Python
The product()
function straightforwardly returns the product of the specified columns ordered by the axis that the programmer requires.
The syntax for the product()
function is shown below for ease of understanding.
DataFrame.product(
axis=None, skipna=None, level=None, numeric_only=None, min_count=0, **kwargs
)
All the parameters of the product()
function have been thoroughly explained below.
axis
: As the name suggests, it defines the axis, 0 being the one for the index while 1 for a column.skipna
: It takes in a Boolean value. By default, the value is taken to beNone
. All NA/null values are excluded while calculating the result if it is seen to beTrue
.level
: It defaults toNone
. It simply represents the hierarchy of the index.numeric_only
: It takes in a Boolean value. By default, the value is taken to beNone
. If seen asTrue
, only int, float, and boolean columns are included in this parameter.min_count
: It is usually an int value that defaults to 0. It specifies the number of necessary and valid values to perform a given operation.**kwargs
: Any additional keywords that need to be passed are through this.
The following code uses the product()
function to find the product of several columns in a Pandas DataFrame in Python.
Example:
import pandas as pd
df1 = pd.DataFrame({"A": [8, 4], "B": [6, 2], "C": [1, 9]})
print(df1)
print(df1[["A", "B"]].product(axis=1))
Output:
A B C
0 8 6 1
1 4 2 9
0 48
1 8
dtype: int64
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn