How to Get the Length of List in Python Pandas Dataframe
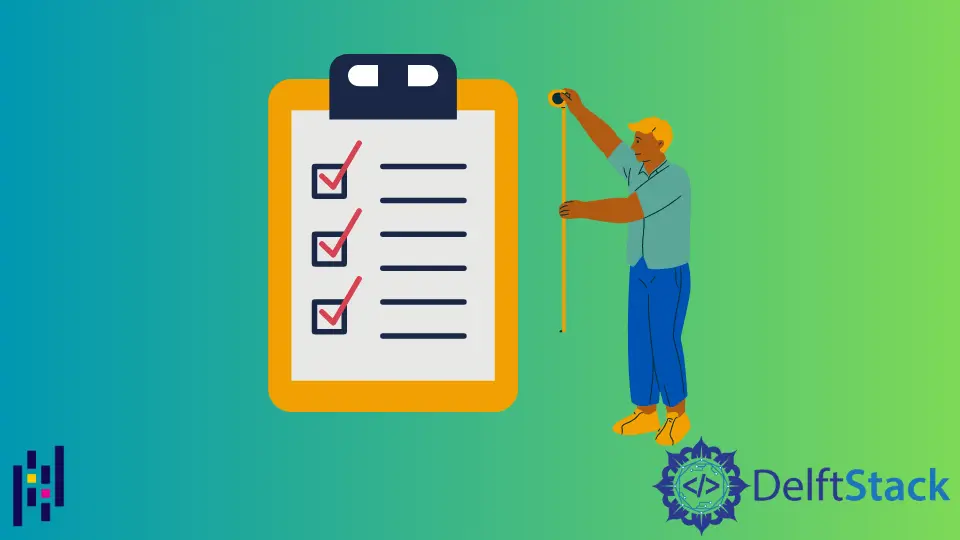
Because lists are an essential component of day-to-day Python programming, all Python users should learn to utilize them. Understanding their utility and operations is fundamental.
This article will look at one method for determining the number of elements in a list.
Determine the Length of the List in Pandas Dataframe Using the len()
Method
The len()
method is the most popular and straightforward way to determine the length of any list. This is the most often used approach among programmers nowadays.
Example:
import pandas as pd
data = {
"os": [
["ubuntu", "mac-osx", "syslinux"],
["ubuntu", "mod-rewrite", "laconica", "apache-2.2"],
["ubuntu", "nat", "squid", "mikrotik"],
]
}
index = ["2013-12-22 15:25:02", "2009-12-14 14:29:32", "2013-12-22 15:42:00"]
df = pd.DataFrame(data, index)
As you can see, we imported the Python Pandas module. Then, we create a two-variable data and index. Create an os
column with its values in the data column.
The index
variable stores the date and time.
Output:
| index | os |
| ------------------- | -------------------------------------- |
| 2013-12-22 15:25:02 | ubuntu,mac-osx,syslinux |
| 2009-12-14 14:29:32 | ubuntu,mod-rewrite,laconica,apache-2.2 |
| 2013-12-22 15:42:00 | ubuntu,nat,squid,mikrotik |
Now, we create a 3rd column named Length
and use the os.map(len)
function that tells the length of the list column in the dataframe.
df["Length"] = df.os.map(len)
df
Output:
| index | os | Length |
| ------------------- | -------------------------------------- | ------ |
| 2013-12-22 15:25:02 | ubuntu,mac-osx,syslinux | 3 |
| 2009-12-14 14:29:32 | ubuntu,mod-rewrite,laconica,apache-2.2 | 4 |
| 2013-12-22 15:42:00 | ubuntu,nat,squid,mikrotik | 4 |
The above table shows that in the os
column, the list length is 3.
Conclusion
In this article, we have discussed how to determine the length of the dataframe list.
You can quickly determine the list’s length using a dataframe example containing a column with list values. Then, the len()
function finds the column’s length with list values.