How to Create a Pandas Dataframe From a List of Dictionary
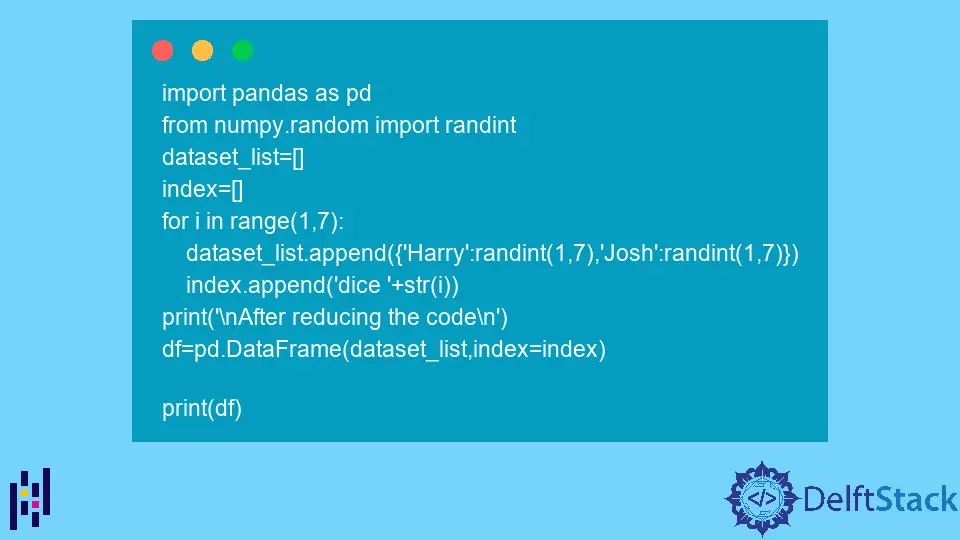
The dictionary is a compact and flexible Python container that stores separate key-value maps. Dictionaries are written in curly brackets ({}
), which include pairs of keywords separated by commas (,)
and :
separate each key from its value.
Three dictionaries are shown below, containing an example of a dice game.
Let’s take an example from a dice game. Two players roll their six dices and store dices with corresponding players in this case.
import pandas as pd
from numpy.random import randint
# create datset from multiple dictionaries
dataset_list = [
{"Harry": 1, "Josh": 3, "dices": "first dice"},
{"Harry": 5, "Josh": 1, "dices": "second dice"},
{"Harry": 6, "Josh": 2, "dices": "third dice"},
{"Harry": 2, "Josh": 3, "dices": "fourth dice"},
{"Harry": 6, "Josh": 6, "dices": "fifth dice"},
{"Harry": 4, "Josh": 3, "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
print()
harry = []
josh = []
for i in range(6):
harry.append(randint(1, 7))
josh.append(randint(1, 7))
We created a dataset from a list of items that contain a dictionary as we know that the DataFrame
takes key-value pair. That’s why this is appropriate with a dictionary.
Output:
Harry Josh dices
0 1 3 first dice
1 5 1 second dice
2 6 2 third dice
3 2 3 fourth dice
4 6 6 fifth dice
5 4 3 sixth dice
We manually set dice in the last example; now, we will use a randint
method defined in the numpy
library. We created two blank lists named harry
and josh
in the following line. Next, we created a for
loop, this range is defined 0-6, appending random numbers in two defined lists as an element using append()
method, look at below.
import pandas as pd
from numpy.random import randint
print()
harry = []
josh = []
for i in range(6):
harry.append(randint(1, 7))
josh.append(randint(1, 7))
# create datset from multiple dictionaries
dataset_list = [
{"Harry": harry[0], "Josh": josh[0], "dices": "first dice"},
{"Harry": harry[1], "Josh": josh[1], "dices": "second dice"},
{"Harry": harry[2], "Josh": josh[2], "dices": "third dice"},
{"Harry": harry[3], "Josh": josh[3], "dices": "fourth dice"},
{"Harry": harry[4], "Josh": josh[4], "dices": "fifth dice"},
{"Harry": harry[5], "Josh": josh[5], "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
Remember, randint()
takes range from given one to n-1
or by default zero to n-1
that’s why we defined range from 1-7
.
output
Harry Josh dices
0 4 1 first dice
1 4 2 second dice
2 3 4 third dice
3 1 1 fourth dice
4 4 5 fifth dice
5 4 4 sixth dice
Now we reduced lines of code with the help of the for
loop and appended the whole dictionary in a list, further append indexes in the list named index
correspond with the player turns and set as an index in DataFrame
.
import pandas as pd
from numpy.random import randint
dataset_list = []
index = []
for i in range(1, 7):
dataset_list.append({"Harry": randint(1, 7), "Josh": randint(1, 7)})
index.append("dice " + str(i))
print("\nAfter reducing the code\n")
df = pd.DataFrame(dataset_list, index=index)
print(df)
output:
Harry Josh
dice 1 2 4
dice 2 2 3
dice 3 6 5
dice 4 5 2
dice 5 4 2
dice 6 1 1
All examples:
import pandas as pd
from numpy.random import randint
# create datset from multiple dictionaries
dataset_list = [
{"Harry": 1, "Josh": 3, "dices": "first dice"},
{"Harry": 5, "Josh": 1, "dices": "second dice"},
{"Harry": 6, "Josh": 2, "dices": "third dice"},
{"Harry": 2, "Josh": 3, "dices": "fourth dice"},
{"Harry": 6, "Josh": 6, "dices": "fifth dice"},
{"Harry": 4, "Josh": 3, "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
print()
harry = []
josh = []
for i in range(6):
harry.append(randint(1, 7))
josh.append(randint(1, 7))
# create datset from multiple dictionaries
dataset_list = [
{"Harry": harry[0], "Josh": josh[0], "dices": "first dice"},
{"Harry": harry[1], "Josh": josh[1], "dices": "second dice"},
{"Harry": harry[2], "Josh": josh[2], "dices": "third dice"},
{"Harry": harry[3], "Josh": josh[3], "dices": "fourth dice"},
{"Harry": harry[4], "Josh": josh[4], "dices": "fifth dice"},
{"Harry": harry[5], "Josh": josh[5], "dices": "sixth dice"},
]
df = pd.DataFrame(dataset_list)
print(df)
dataset_list = []
index = []
for i in range(1, 7):
dataset_list.append({"Harry": randint(1, 7), "Josh": randint(1, 7)})
index.append("dice " + str(i))
print("\nAfter reducing the code\n")
df = pd.DataFrame(dataset_list, index=index)
print(df)
output:
Harry Josh dices
0 1 3 first dice
1 5 1 second dice
2 6 2 third dice
3 2 3 fourth dice
4 6 6 fifth dice
5 4 3 sixth dice
Harry Josh dices
0 4 1 first dice
1 4 2 second dice
2 3 4 third dice
3 1 1 fourth dice
4 4 5 fifth dice
5 4 4 sixth dice
After reducing the code
Harry Josh
dice 1 2 4
dice 2 2 3
dice 3 6 5
dice 4 5 2
dice 5 4 2
dice 6 1 1