How to Add Image to Pandas Dataframe
- Understanding Pandas DataFrame
- Method 1: Adding Images as File Paths
- Method 2: Embedding Images Directly
- Method 3: Using Image URLs
- Conclusion
- FAQ
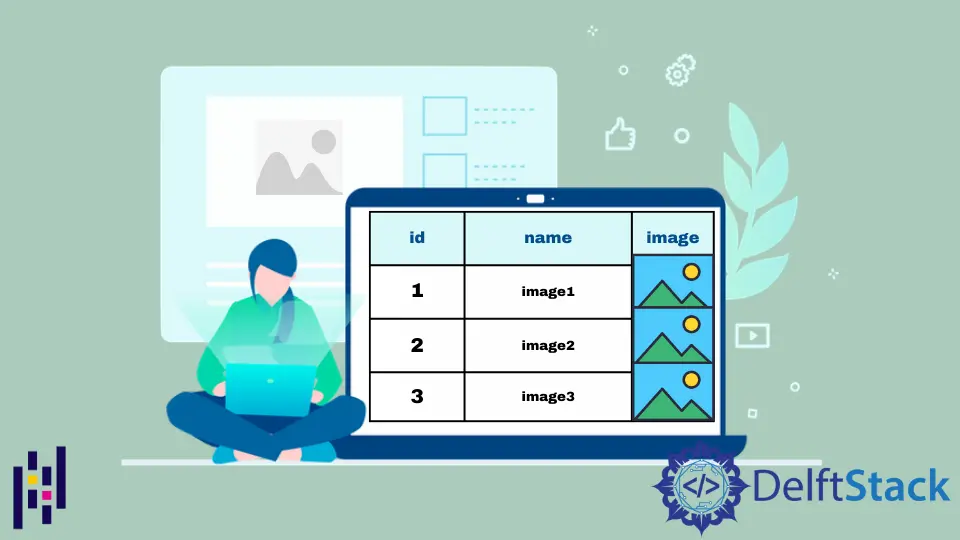
In the world of data analysis, visual representation plays a crucial role. Whether you’re working on a machine learning project, data visualization, or simply organizing your data, adding images to a Pandas DataFrame can enhance your analysis and make your data more engaging.
This tutorial demonstrates how to add images to a Pandas DataFrame using Python. We will explore different methods to achieve this, ensuring that you have a complete understanding of the process. By the end of this article, you will be equipped with the knowledge to integrate images seamlessly into your data structures, making your analyses not only more informative but visually appealing as well.
Understanding Pandas DataFrame
Before diving into how to add images to a Pandas DataFrame, let’s quickly recap what a DataFrame is. In Python, a DataFrame is a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). Pandas, a powerful data manipulation library, allows users to work with DataFrames easily. You can think of it as an Excel spreadsheet in Python, where you can store various data types, including integers, floats, strings, and even images.
Method 1: Adding Images as File Paths
One of the simplest ways to include images in a Pandas DataFrame is to store the file paths of the images. This method involves creating a DataFrame with a column that contains the paths to the images. Here’s how to do it:
import pandas as pd
data = {
'Name': ['Image1', 'Image2'],
'ImagePath': ['path/to/image1.png', 'path/to/image2.png']
}
df = pd.DataFrame(data)
print(df)
Output:
Name ImagePath
0 Image1 path/to/image1.png
1 Image2 path/to/image2.png
In this example, we create a DataFrame with two columns: “Name” and “ImagePath.” The “ImagePath” column contains the paths to the images. While this method does not display the images directly in the DataFrame, it provides a straightforward way to reference images stored on your local system or a remote server. You can later use libraries like Matplotlib or PIL to visualize these images as needed.
Method 2: Embedding Images Directly
If you want to display images directly within a Jupyter Notebook or any environment that supports HTML rendering, you can embed images in a DataFrame using the HTML representation. This method is particularly useful for creating visually appealing reports. Here’s how you can do it:
from IPython.display import HTML
import pandas as pd
data = {
'Name': ['Image1', 'Image2'],
'Image': ['<img src="path/to/image1.png" width="100" />',
'<img src="path/to/image2.png" width="100" />']
}
df = pd.DataFrame(data)
HTML(df.to_html(escape=False))
In this snippet, we create a DataFrame with an “Image” column that contains HTML <img>
tags. The images are displayed as part of the DataFrame when rendered in a Jupyter Notebook. The escape=False
argument in to_html()
ensures that the HTML tags are rendered correctly instead of being escaped. This method allows you to visualize images alongside your data, making it easier to analyze and present your findings.
Method 3: Using Image URLs
Another effective way to add images to a Pandas DataFrame is by using URLs. If your images are hosted online, you can directly link to them. This method is particularly useful when sharing your DataFrame with others, as they can access the images without needing local files. Here’s how to implement this:
import pandas as pd
data = {
'Name': ['Image1', 'Image2'],
'ImageURL': ['https://example.com/image1.png',
'https://example.com/image2.png']
}
df = pd.DataFrame(data)
print(df)
Output:
Name ImageURL
0 Image1 https://example.com/image1.png
1 Image2 https://example.com/image2.png
In this example, we create a DataFrame with an “ImageURL” column containing links to images hosted online. This approach is beneficial for collaborative projects or when working in cloud environments. Users can easily access the images via the provided URLs. You can also use libraries like Matplotlib to fetch and display these images directly in your analysis.
Conclusion
Adding images to a Pandas DataFrame can significantly enhance your data analysis and visualization capabilities. Whether you choose to store file paths, embed images directly, or use URLs, each method has its advantages and can be tailored to your specific needs. By incorporating images, you can create more engaging reports, presentations, and analyses that communicate your findings effectively. Now that you’re equipped with these techniques, you can experiment with different methods to see which best suits your projects.
FAQ
-
Can I add images from a local directory to a Pandas DataFrame?
Yes, you can add images by specifying their file paths in a DataFrame column. -
How can I display images in a Jupyter Notebook?
You can embed images using HTML<img>
tags within a DataFrame and render it usingHTML()
. -
Is it possible to use online images in a Pandas DataFrame?
Absolutely! You can link to images hosted online using their URLs. -
Can I manipulate images stored in a Pandas DataFrame?
Yes, you can use libraries like PIL or OpenCV to manipulate images after retrieving them from the DataFrame. -
What is the best method to add images for data sharing?
Using image URLs is the most efficient way to share images in a DataFrame, as it does not require local files.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn