How to Set Port in Flask
- Use Code Prompt of Visual Studio Code to Set Port in Flask
- Use Command Line Interface (CLI) to Set Port in Flask
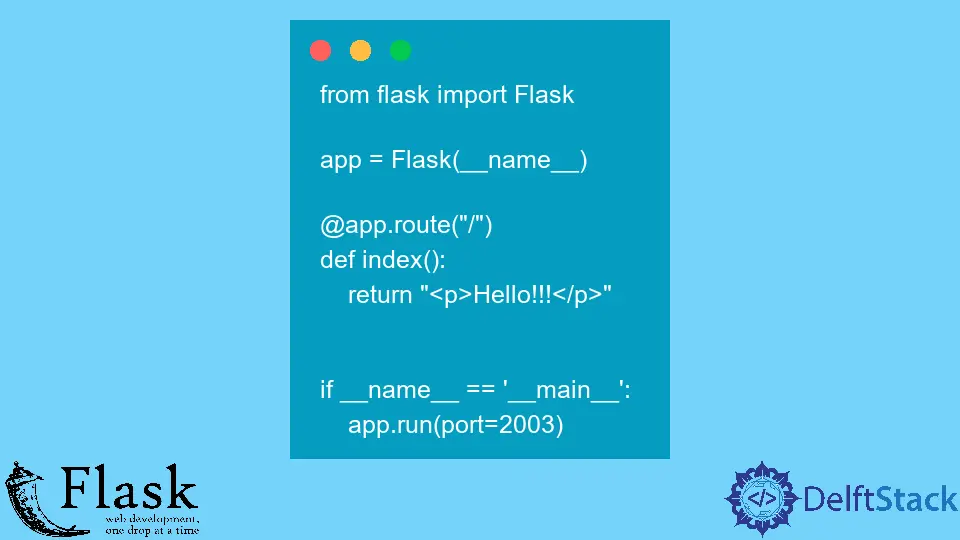
Here, in this guide, we will examine the question: How do we set up a port in Flask? So let us not wait any further and start with this guide.
Use Code Prompt of Visual Studio Code to Set Port in Flask
We will look into two ways to set up a port. One uses only a command prompt, and the other uses software; in this case, Visual Studio Code is used.
This method requires the installation of Visual Studio Code; the link is provided here. Visual Studio Code prompts us to set up a port using a simple function.
the app.run()
Function
Let us look into this with some simple explanation to further understand how to set up a port. The app.run()
function allows one to run an application.
It can run with or without any arguments. It will consider the arguments its default state if they contain no arguments.
When setting up a port, we only have to type in our desired port, and the system will run it successfully.
app.run(port=2003)
Here is an example code that shows the way to use this argument properly.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def index():
return "<p>Hello!!!</p>"
if __name__ == "__main__":
app.run(port=2003)
The application’s port number is now changed from 5000
to 2003
, as seen in the output below.
Use Command Line Interface (CLI) to Set Port in Flask
Using the systems command prompt, we can easily change our applications port without needing to type any code. We can use any code and make these changes while executing.
flask --app <app_name> run --port=2003
flask --app f run --port=2003
Suppose we are using a file name f.py
. By typing this command, the system will recognize the user’s need to change the port number from its default, 5000
, to the desired, 2003
.
By using these methods, one can easily change the port number.
* Serving Flask app 'f'
* Debug mode: off
WARNING: This is a development server. Do not use it in a production deployment. Use a production WSGI server instead.
* Running on http://127.0.0.1:2003
Press CTRL+C to quit
As we can see from this output, using either of these methods does not have any changes in the output.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn