Flask g Object
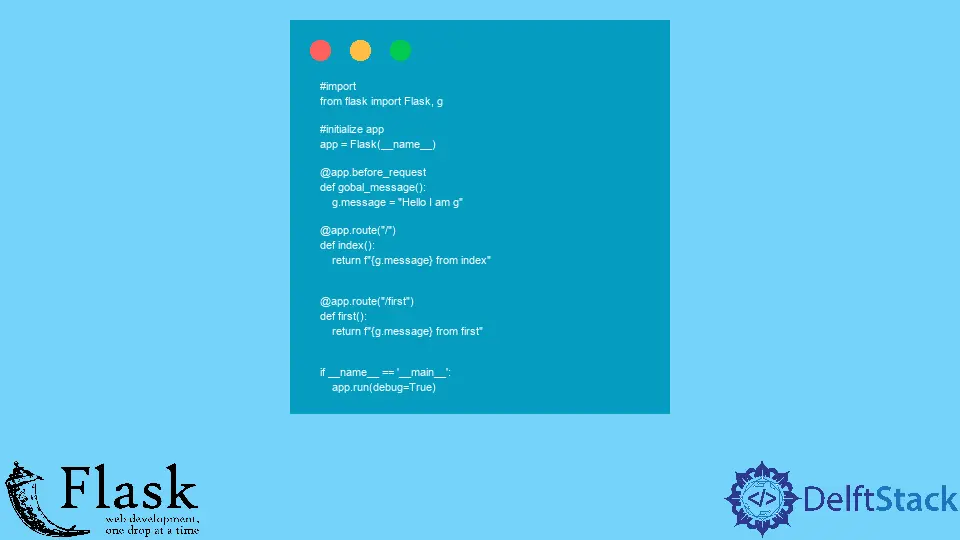
In this guide, we will learn about g
in Flask. What is a g
object?
What is this object used for, and when is the g
object used? So, without delay, let’s learn.
the g
Object in Flask
Flask provides us with an object named g
. This object stores common data while running an application during a request from the client or a CLI (Command Line Interface) command.
The meaning of g
is global
, and it is a simple namespace that is globally used, which means its lifetime is the same as the application it is used in.
The object g
is used to hold data that can be used whenever it is called upon within the context of the application since it remains constant throughout.
Usually, flask.global
is used to import this object, but by simply using the flask
module, we can import the object g
as well, as it is a shortcut.
Here’s an example of the g
object being called on the index and first pages.
# import
from flask import Flask, g
# initialize app
app = Flask(__name__)
@app.before_request
def gobal_message():
g.message = "Hello I am g"
@app.route("/")
def index():
return f"{g.message} from index"
@app.route("/first")
def first():
return f"{g.message} from first"
if __name__ == "__main__":
app.run(debug=True)
Output in index "/"
page:
Hello I am g from index
Output in first "/first"
page:
Hello I am g from first
As seen in the above example, g.message
is initialized only once, and we can use it as many times as we want within the scope of the application.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn