Ternary Operator in PowerShell
- Understanding the Ternary Operator Syntax
- Using the Ternary Operator for Conditional Outputs
- Combining the Ternary Operator with Other PowerShell Features
- Conclusion
- FAQ
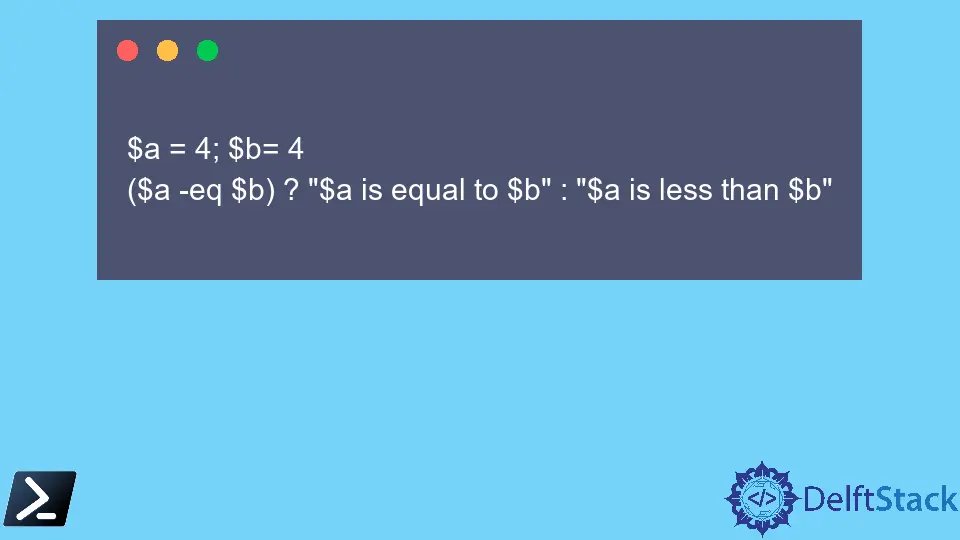
If you’re diving into PowerShell scripting, you may have heard of the ternary operator. This compact and efficient tool allows you to evaluate conditions and return values based on those evaluations, all in a single line of code. Think of it as a shorthand way to write an if-else statement.
In this tutorial, we’ll explore how the ternary operator works in PowerShell, its syntax, and practical examples to help you grasp its functionality. Whether you’re a seasoned scripter or a beginner, understanding the ternary operator can enhance your coding efficiency and streamline your scripts. Let’s get started!
Understanding the Ternary Operator Syntax
The ternary operator in PowerShell follows a straightforward syntax:
<condition> ? <true_value> : <false_value>
Here’s how it works: you define a condition that evaluates to either true or false. If the condition is true, the operator returns the true_value; if false, it returns the false_value. This compact structure not only saves space but also makes your code easier to read at a glance.
Example of Ternary Operator in Action
Let’s look at a simple example to illustrate how the ternary operator can be utilized in PowerShell.
$age = 18
$status = $age -ge 18 ? "Adult" : "Minor"
$status
Output:
Adult
In this example, we check if the variable $age
is greater than or equal to 18. If true, the variable $status
is assigned the value “Adult”; otherwise, it gets “Minor”. The ternary operator condenses the logic into a single line, making the code cleaner and easier to maintain.
Using the Ternary Operator for Conditional Outputs
One of the most practical applications of the ternary operator is in generating conditional outputs. This can be particularly useful in scripts that require user feedback based on certain conditions.
Example of Conditional Outputs
Consider a scenario where you want to display a message based on whether a user is logged in or not.
$isLoggedIn = $true
$message = $isLoggedIn ? "Welcome back!" : "Please log in."
$message
Output:
Welcome back!
In this case, we check the $isLoggedIn
variable. If it’s true, the message “Welcome back!” is displayed. If false, the message prompts the user to log in. This concise approach not only enhances readability but also keeps the code straightforward.
Combining the Ternary Operator with Other PowerShell Features
The ternary operator can also be combined with other PowerShell features, such as arrays and functions, to create more complex logic in your scripts.
Example of Combining Features
Let’s say you have an array of numbers, and you want to label them as “Even” or “Odd”. The ternary operator can help streamline this process.
$numbers = 1..5
$labels = $numbers | ForEach-Object { $_ % 2 -eq 0 ? "Even" : "Odd" }
$labels
Output:
Odd
Even
Odd
Even
Odd
In this example, we use a range of numbers from 1 to 5. The ForEach-Object
cmdlet iterates through each number, applying the ternary operator to determine if the number is even or odd. The results are stored in the $labels
array, showcasing how the ternary operator can efficiently handle multiple evaluations within a single pipeline.
Conclusion
The ternary operator in PowerShell is a powerful tool that can significantly enhance your scripting capabilities. By allowing you to condense conditional logic into a single line, it improves both the readability and efficiency of your code. Whether you’re working with simple conditions or integrating it into more complex scripts, mastering the ternary operator can lead to cleaner and more maintainable code. As you continue to explore PowerShell, keep this handy operator in mind to streamline your scripting processes.
FAQ
-
What is a ternary operator?
A ternary operator is a shorthand way to write an if-else statement, allowing for concise conditional evaluations. -
Can the ternary operator be used in all programming languages?
While many programming languages support a form of the ternary operator, the syntax may vary. PowerShell has its own specific syntax. -
Is the ternary operator faster than traditional if-else statements?
The performance difference is usually negligible, but the ternary operator can make your code cleaner and easier to read. -
When should I use the ternary operator?
Use the ternary operator for simple conditions where you want to return one of two values based on a true/false evaluation. -
Can I nest ternary operators in PowerShell?
Yes, you can nest ternary operators, but be cautious as it can make your code harder to read.