How to Split Directory Path in PowerShell
-
Use
Split-Path
Cmdlet to Split Directory or File Path in PowerShell -
Use the
Split()
Method to Split Directory or File Path in PowerShell
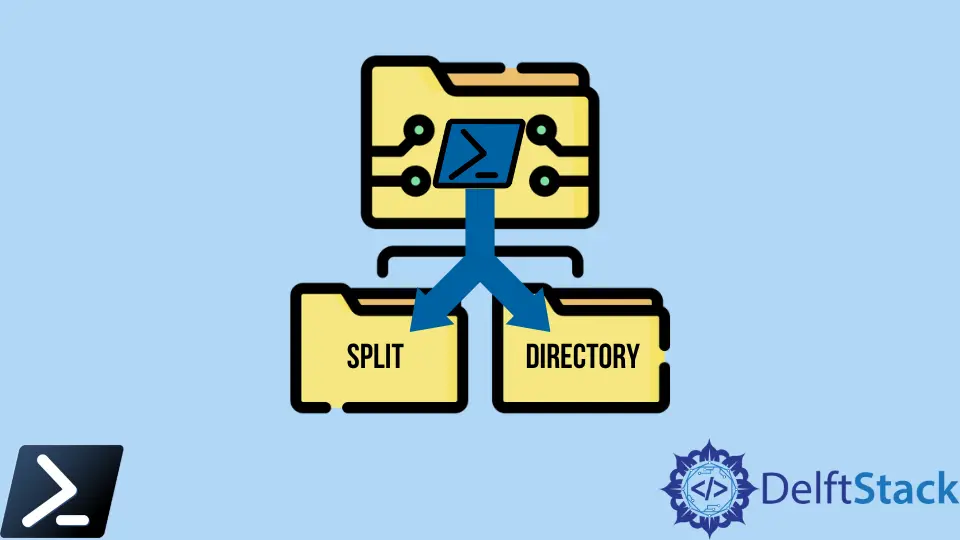
While working with paths in PowerShell, sometimes you may need to split the directory or file path. PowerShell has a handy cmdlet Split-Path
that lets you split a path to a parent path, subfolder, or file name.
This tutorial will teach you to split the directory or file path in PowerShell.
Use Split-Path
Cmdlet to Split Directory or File Path in PowerShell
The Split-Path
cmdlet returns the specific part of a given path in PowerShell. The part of a path can be the parent folder, subfolder, file name, or a file extension only.
By default, the Split-Path
returns the parent folder of the path. The following example will display C:\Windows\System32
, the parent folder of the notepad.exe
.
Command:
Split-Path -Path "C:\Windows\System32\notepad.exe"
Output:
C:\Windows\System32
The -Qualifier
parameter displays the qualifier of the path. The qualifier is the drive of the path, such as C:
, D:
.
Command:
Split-Path -Path "C:\Windows\System32\notepad.exe" -Qualifier
Output:
C:
The -Leaf
parameter prints the last item of the path.
Command:
Split-Path -Path "C:\Windows\System32\notepad.exe" -Leaf
Output:
notepad.exe
To display the base name of the leaf, use the LeafBase
parameter. It returns the file name without an extension.
Command:
Split-Path -Path "C:\Windows\System32\notepad.exe" -LeafBase
Output:
notepad
You can use the -Extension
parameter to get only the leaf extension.
Command:
Split-Path -Path "C:\Windows\System32\notepad.exe" -Extension
Output:
.exe
You can also split paths of the registry path with Split-Path
.
Command:
Split-Path HKCU:\Software\Microsoft
Output:
HKCU:\Software
Use the Split()
Method to Split Directory or File Path in PowerShell
To divide a string into an array, use the Split()
method. You can use this method to split strings of a path into an array.
Then you can use Select-Object
to select specific positions in an array and join them as a path. The following example splits the path C:\Windows\System32\notepad.exe
to C:\Windows
.
Command:
$path = "C:\Windows\System32\notepad.exe".Split("\") | Select-Object -First 2
$path -join "\"
The split method splits a path string on the delimiter \
in the above script. It is then piped to the Select-Object
, selecting only the first two objects from an array.
The outcome of the first command is stored on the variable $path
. The second command joins the resulting objects in $path
with \
and creates a new path.
Output:
C:\Windows
The following example splits the path C:\Windows\System32\notepad.exe
to System32\notepad.exe
.
Command:
$path = "C:\Windows\System32\notepad.exe".Split("\") | Select-Object -Last 2
$path -join "\"
Output:
System32\notepad.exe
Suppose you need the second and last element in the path. Then you can use the -Index
parameter to select specific positions in an array.
The -Index
parameter selects indexes 1
and 3
. The index value in an array starts from 0
.
Command:
$path = "C:\Windows\System32\notepad.exe".Split("\") | Select-Object -Index 1, 3
$path -join "\"
Output:
Windows\notepad.exe
In this article, we learned several examples to split paths in PowerShell. We also showed you how to join the path with a \
delimiter.