How to Recursively Set Permissions on Folders Using PowerShell
- Get Permissions on Folders Recursively Using PowerShell
- Set Permissions on Folders Recursively Using PowerShell
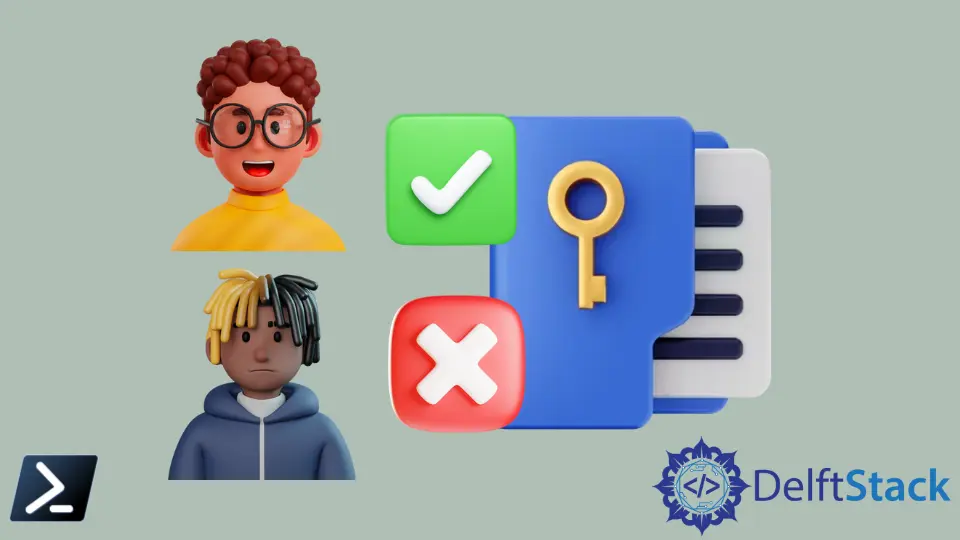
An ACL (access control list) represents users’ permissions and user groups for accessing a file or resource. It is an ordered list of access control entries (ACEs).
Each ACE in an ACL defines the access rights allowed, denied, or audited. The security descriptor for an object can have two types of ACLs: DACL and SACL.
When working with NTFS permissions on Windows, you might need to recursively change the permissions on folders. A GUI would be more time-consuming and complex than a PowerShell script.
This tutorial will teach you to recursively set permissions on folders using PowerShell.
Get Permissions on Folders Recursively Using PowerShell
There are mainly two cmdlets for managing ACL permissions in PowerShell: Get-Acl
and Set-Acl
.
The Get-Acl
cmdlet gets the security descriptor which contains the access control lists (ACLs) of a file or resource.
The Get-Acl
gets the security descriptor of the C:\New
directory.
Get-Acl C:\New
Output:
Directory: C:\
Path Owner Access
---- ----- ------
New DelftStack\rhntm BUILTIN\Administrators Allow FullControl...
The Get-Acl
cmdlet does not return all directories and sub-directories permissions. You will need to use the Get-ChildItem
cmdlet with the -Recurse
parameter to get permissions of folders recursively.
Get-ChildItem "C:\pc" -Recurse | Get-ACL
Set Permissions on Folders Recursively Using PowerShell
The Set-Acl
changes the security descriptor of a file or resource. It applies the security descriptor supplied as the value of the -AclObject
parameter.
The following commands copy the values from the security descriptor of the C:\New
directory to the security descriptor of the C:\pc
directory.
$new = Get-Acl -Path "C:\New"
Set-Acl -Path "C:\pc" -AclObject $new
The first command gets the security descriptor of the C:\New
directory and stores it in the $new
variable. In the second command, Set-Acl
changes the values in the ACL of the C:\New
directory to the values in $new
.
The following script adds the new ACL rule to the existing permissions on the folder recursively.
$acl = Get-Acl -Path "C:\pc"
$ace = New-Object System.Security.Accesscontrol.FileSystemAccessRule ("testuser", "Read", "Allow")
$acl.AddAccessRule($ace)
Set-Acl -Path "C:\pc" -AclObject $acl
The first command gets the existing ACL rules. The second command creates a new FileSystemAccessRule
to apply.
The third command adds the new ACL rule to the existing permissions on the folder. The fourth command uses Set-Acl
to apply the new ACL to the folder.
You can view all users’ permissions using the following command.
(Get-ACL -Path "C:\pc").Access | Format-Table IdentityReference, FileSystemRights, AccessControlType
Output:
IdentityReference FileSystemRights AccessControlType
----------------- ---------------- -----------------
DelftStack\rhntm FullControl Allow
DelftStack\testuser Read, Synchronize Allow
System administrators configure NTFS permissions for many folders and files using scripts to make the process faster and easy. We hope this article helped you understand how to set permissions on folders recursively using PowerShell.
For the detailed NTFS permissions type in PowerShell, read this post.