How to Send Email Message to Multiple Recipients in PowerShell
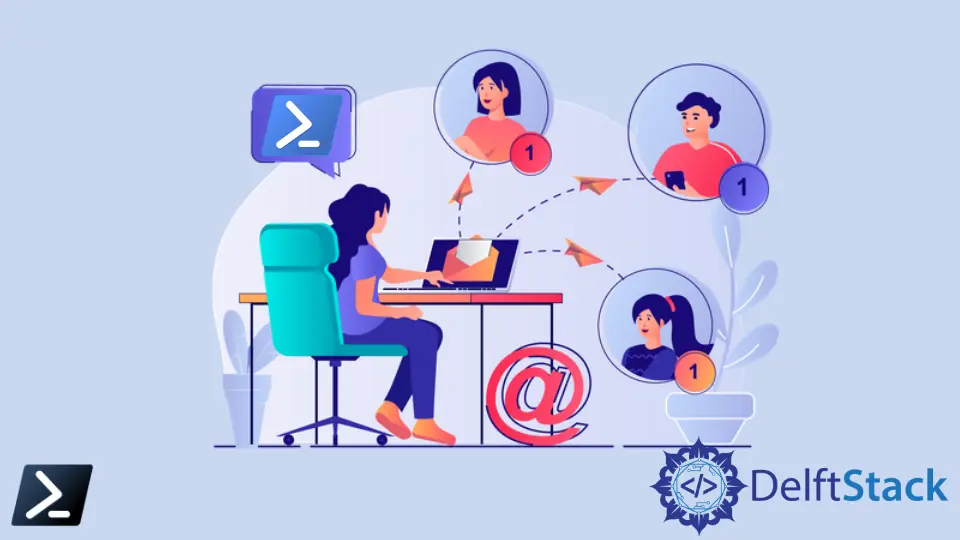
Sending email messages automatically to a set of users is one common requirement and a confusing point to developers of different program languages. Through PowerShell, this requirement can be enabled.
This article will discuss how to send an email message to multiple recipients through PowerShell.
Sending an Email Message to an Array of Recipients in PowerShell
A common issue to some users is that the email is sent only to one recipient, the first recipient of the list given. There are ways to automate sending emails to all the required recipients.
The recipient list has to be passed as a string array
. If you give the list, it will not be properly sent to all the required users. The code below is for adding several recipients.
[string[]]$recieverslist = "Asenika <asenika@gmail.com>", "Mark <mark@kln.au.lk>"
The command above will create a list of recipients to which you can send the email with attachments. You have to give the recipient the $recieverslist
in the following sample.
Send-MailMessage -From "test@123" `
-To "$recieverslist" `
-Subject "test" `
-Body " test test test" `
With the above command followed by correct server details, encryption, etc., you can send the email to the full list of receivers in the above $recieverslist
. Therefore, users can avoid the issue of multiple recipients.
Another way of doing this is by giving the list a parameter
and splitting it.
PS C:\Users\Agni> $receiverslist = "asenika@gmail.com;MArk@domain.org"
>> $username = "asenika@gmail.com"
>> $password = "password"
>>
>> $password = ConvertTo-SecureString -String $password -AsPlainText -Force
>> $credentials = New-Object System.Management.Automation.PSCredential $username, $password
>> $emailParameter = @{
>> To = $receiverslist.Split(';')
>> From = "Asenika <asenika@gmail.com>"
>> Subject = "tesing"
>> Body = "testing"
>> SmtpServer = "smtp.gmail.com"
>> Port = 587 #465
>> Credential = $credentials
>> UseSsl = $true
>> }
Here, the output would be an email sent to the specified users.
You can read more about PowerShell and its commands here.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.