How to Run a Git Command in PowerShell
- Download and Install Git on the Computer
- Add Git Binaries to Your System Path Variable
- Run a Git Command in PowerShell
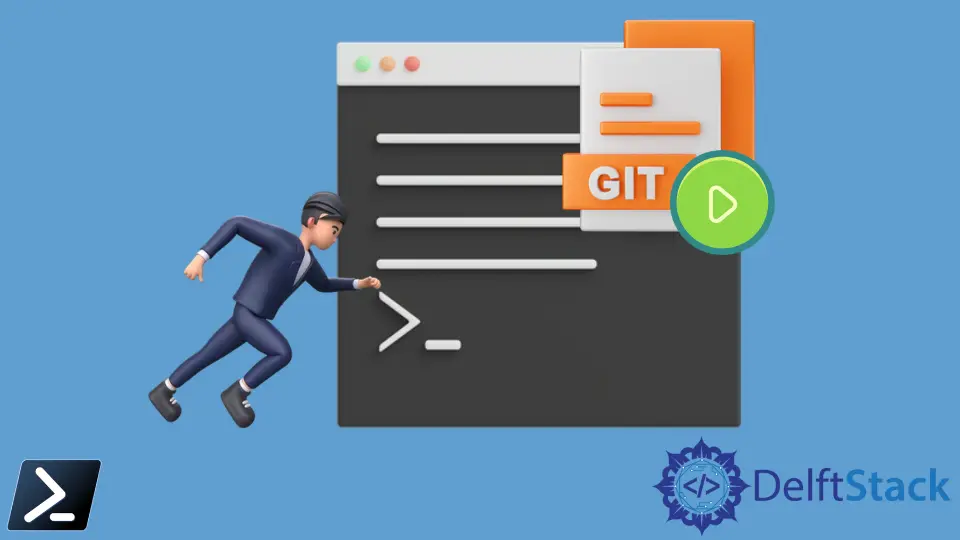
Git is a version control system used for tracking changes in the source code. Multiple developers work together on a single project using Git to track what changes are made.
You can use Git by running Git-specific commands or using the graphical user interface (GUI) such as GitHub Desktop, Sourcetree, GitKraken, etc. We can run Git commands from the command prompt or PowerShell.
This tutorial will teach you to run a Git command with PowerShell.
Download and Install Git on the Computer
First, you have to install Git to run its commands on your computer. So, go to this link and download the latest version of Git.
After the setup file is downloaded, open the file to begin the installation of Git on the computer. You can leave the default options when installing Git.
Add Git Binaries to Your System Path Variable
After installing Git, you must add the Git\bin
path to your system path variable.
Go to Advanced system settings
and click Environment Variables
.
Select Path
and click Edit
to edit environment variables.
Then click New
and add the complete path of the Git\bin
folder. If you have used the default settings for installation, it should be C:\Program Files\Git\bin
.
After configuring all the settings above, click OK
in all dialog boxes to save the changes.
Run a Git Command in PowerShell
Now, open the PowerShell and type git --version
to check whether the Git installation is successful.
git --version
Output:
git version 2.35.1.windows.2
As you can see, git 2.35.1
is installed on our computer.
Let’s run a Git command to create a new empty repository. The following command initializes the .git
repository on the current working directory.
git init
Output:
Initialized empty Git repository in C:/Users/rhntm/.git/
We hope this article helped you with running Git commands in PowerShell.