How to Redirect a PowerShell Output to a File During Execution
-
Use the
Out-File
Cmdlet to Redirect a PowerShell Output to a File During Execution -
Use the
Set-Content
to Redirect a PowerShell Output to a File During Execution -
Use the
Redirection Operators
to Redirect a PowerShell Output to a File During Execution -
Use the
Tee-Object
to Redirect a PowerShell Output to a File During Execution
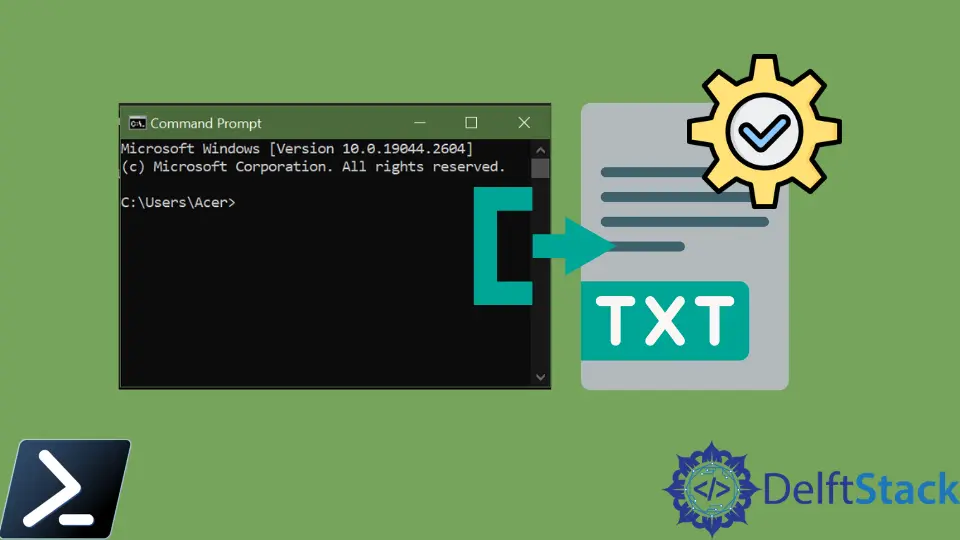
One of the fundamental features of PowerShell is that it formats the output automatically. You run a command or script, and PowerShell returns the result to the console.
But sometimes, there are situations when you need to write the output to a file rather than the console.
This tutorial will introduce different methods to redirect the output of a PowerShell to a file during its execution.
Use the Out-File
Cmdlet to Redirect a PowerShell Output to a File During Execution
The Out-File
cmdlet sends output to a file. It uses PowerShell’s formatting system to write to the file.
The outputs of the command or script are sent down the pipeline to the Out-File
cmdlet. The content in the file is saved in the same way as displayed on the console.
Get-Command gcc | Out-File -FilePath C:\new\test.txt
Output:
The output of Get-Command gcc
is sent to a file test.txt
.
You can use the -Append
parameter to add the output to the end of a file without replacing the existing content.
Get-Command git | Out-File -FilePath C:\new\test.txt -Append
Output:
The output of Get-Command git
is added to the end of a test.txt
file.
Use the Set-Content
to Redirect a PowerShell Output to a File During Execution
The Set-Content
is a string-processing cmdlet that writes new content or replaces the content in a file.
It converts the objects of command or script you send in the pipeline to strings and writes to a specified file.
./myscript.ps1 | Set-Content -Path C:\New\hello.txt
Output:
The output of myscript.ps1
is sent to a file hello.txt
.
The Set-Content
replaces the existing content in the file. You can use the Add-Content
cmdlet to append content to a specified file.
Get-Date | Add-Content -Path C:\New\test.txt
Output:
The output of Get-Date
is added to the end of a test.txt
file.
Use the Redirection Operators
to Redirect a PowerShell Output to a File During Execution
There are two redirection operators in PowerShell that you can use to redirect the output to a file. One is >
, equivalent to the Out-File
, and another is >>
, equivalent to the Out-File -Append
.
The >
writes new content or replaces existing content in a file.
Get-Date > C:\New\new.txt
Whereas >>
appends content to a specified file.
Get-Date >> C:\New\new.txt
Use the Tee-Object
to Redirect a PowerShell Output to a File During Execution
The Tee-Object
cmdlet (like the letter T
) sends a command or script output in two directions. It stores the output in a file or variable and sends it down the pipeline.
If Tee-Object
is the final command in the pipeline, the console also displays the output.
Get-Process | Tee-Object -FilePath C:\New\process.txt
Output:
As you can see, the output is saved in a file and displayed on the console.
You can use -Append
with this cmdlet to append the output to a specified file.
Get-Process | Tee-Object -FilePath C:\New\process.txt -Append