How to Read CSV File Line by Line in PowerShell
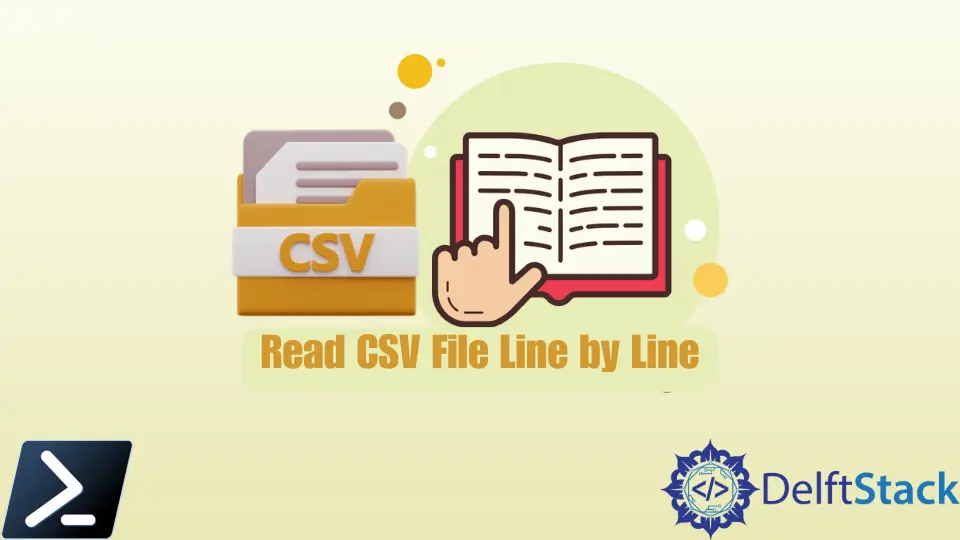
In the previous article we have discussed reading comma-separated files or CSV data and placing it to an array variable using PowerShell. What if we want to read through all the data of the CSV file line per line? This article demonstrates how to loop through PowerShell’s CSV line by line.
Use foreach()
to Read CSV File Line by Line in PowerShell
Let’s use the sample data from our previous CSV file in our last article as an example.
Sample Data (sample.csv
file):
Name,Salary
John,1001
Paul,2001
Mary,1501
Matt,3001
If we recall, the Import-CSV
command in Windows PowerShell will create a table like custom objects and save it in an ArrayList
from the items presented in the CSV file above.
Since PowerShell conveniently transforms our CSV into an object, we can use the foreach
loop to iterate through the whole CSV.
Example Code:
$csv = Import-Csv C:\PS\sample.csv
foreach ($line in $csv) {
$line
}
Now with looping in place, we can conveniently process the CSV file line by line and call their attributes individually per line.
Example Code:
$csv = Import-Csv C:\PS\sample.csv
foreach ($line in $csv) {
$line.Name
$line.Salary
}
Output:
John
1001
Paul
2001
Mary
1501
Matt
3001
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn