Remoting $Using Variable Scope in PowerShell
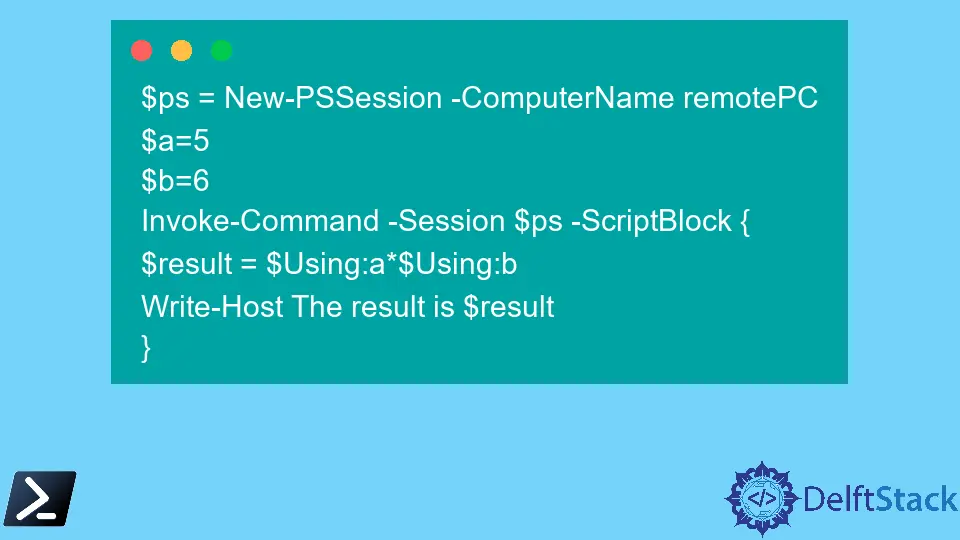
When working with remote commands in PowerShell, sometimes you may want to use local variables in a remote session. But if you use a local variable in remote commands, it will return an error because the variable is not created in the remote session.
By default, PowerShell expects that the variables in remote commands are defined in the remote session in which the command runs. Using
is a special scope modifier that allows you to use local variables in remote commands.
The Using
scope modifier identifies variables defined in the local session as a local variable in the remote command. It is introduced in PowerShell 3.0.
This tutorial will teach you to use local variables in remote commands with Using
in PowerShell.
Use Using
Scope Modifier in PowerShell
The syntax for the Using
scope modifier is:
$Using:VariableName
The Using
scope modifier is supported in remotely executed commands, starting with Invoke-Command
using the ComputerName
, HostName
, SSHConnection
or Session
parameters.
The following script shows the $test
variable is defined in the local computer and used in the remote session.
$test = "C:\New\complex"
Invoke-Command -ComputerName remotePC -ScriptBlock {
Get-ChildItem $Using:test
}
The first command stores the path of the directory C:\New\complex
in the $test
variable in the local computer. The second command runs the Get-ChildItem
command with the local variable $test
in the remote computer.
As a result, it prints the items present in the directory C:\New\complex
in the remote computer.
Output:
Directory: C:\New\complex
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 20-12-2021 19:31 112125 architecture of aqps.png
-a---- 20-12-2021 21:32 33148 formula.png
-a---- 20-12-2021 21:30 55621 modules.png
-a---- 20-12-2021 16:35 190485 output paper.png
You can also use the Using
scope modifier in a PSSession
.
In the following example, two variables, $a
and $b
, are created in the local session and used in the remote session. The $result
variable is defined in the remote session, which stores the multiplication of values in $a
and $b
.
$ps = New-PSSession -ComputerName remotePC
$a = 5
$b = 6
Invoke-Command -Session $ps -ScriptBlock {
$result = $Using:a * $Using:b
Write-Host The result is $result
}
Output:
The result is 30
We hope this tutorial helped you understand how to use the Using
scope modifier for local variables in remote commands. For more information, read about_Remote_Variables
.