How to Measure Runtime of a Script Using PowerShell
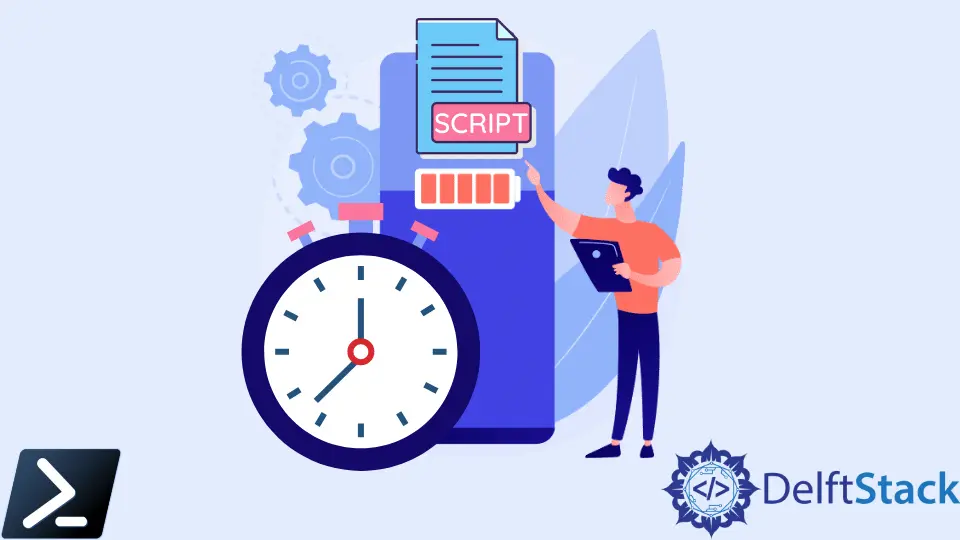
It is expected to check for the runtime of a script after creating it. It helps the developer gauge the script with measurable values if any revisions that will make the script slower or faster.
In Windows PowerShell, there is a way to measure this, and in this article, we will learn how to do that exactly.
Use Measure-Command
Cmdlet to Measure Runtime of a Script in PowerShell
Using the Measure-Command
cmdlet in PowerShell is easy to measure the runtime or execution time. This command can also tell you how long a custom function or an entire script takes to execute.
The Measure-Command
is a cmdlet that is easy to use and provides intuitive, actionable output.
The Measure-Command
cmdlet requires two parameters: -Expression
and -InputObject
. The -InputObject
parameter represents one or more expressions in any way.
It’s simple but provides valuable metrics to give you a glimpse into the performance of your code.
Let’s use Measure-Command
in a performance-tuning example. Say we have an array defined as $arr01 = @()
.
We’ll use integers created with a range to keep it simple. We’ll add these numbers to the array using +=
.
$arr01 = @()
0..10000 | ForEach-Object { $arr01 += $_ }
The snippet above executes, but for how long? Let’s find out with Measure-Command
.
First, we can wrap the code inside a script block representing an expression and use that as the Expression
parameter for Measure-Command
.
Example Code:
Measure-Command -Expression { 0..10000 | ForEach-Object { $arr01 += $_ } }
Output:
Days : 0
Hours : 0
Minutes : 0
Seconds : 0
Milliseconds : 76
Ticks : 762558
TotalDays : 8.82590277777778E-07
TotalHours : 2.11821666666667E-05
TotalMinutes : 0.00127093
TotalSeconds : 0.0762558
TotalMilliseconds : 76.2558
Notice that Measure-Command
returns time broken down into various categories. We don’t need to perform any mathematical operations to convert seconds, minutes, or hours.
It’s all part of the object Measure-Command
returns.
If we need to reference total seconds, we will reference the TotalSeconds
property. We could assign the output of Measure-Command
to a variable and reference all properties.
$slow_performance = Measure-Command -Expression { 0..10000 | ForEach-Object { $arr01 += $_ } }
Let us now use the quicker way of adding elements to an array by using an ArrayList
and see how fast that is.
Example Code:
$arr01 = [System.Collections.ArrayList]@()
$fast_performance = Measure-Command -Expression { 0..10000 | ForEach-Object { $arr01 += $_ } }
$fast_performance
Output:
Days : 0
Hours : 0
Minutes : 0
Seconds : 7
Milliseconds : 346
Ticks : 73465163
TotalDays : 8.50291238425926E-05
TotalHours : 0.00204069897222222
TotalMinutes : 0.122441938333333
TotalSeconds : 7.3465163
TotalMilliseconds : 7346.5163
We can also check the runtime of a PowerShell file (.ps1
) using the Measure-Command
.
Example Code:
Measure-Command { .\checkdrives.ps1 | Out-Default }
Output:
Days : 0
Hours : 0
Minutes : 0
Seconds : 0
Milliseconds : 30
Ticks : 308166
TotalDays : 3.56673611111111E-07
TotalHours : 8.56016666666667E-06
TotalMinutes : 0.00051361
TotalSeconds : 0.0308166
TotalMilliseconds : 30.8166
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn