PowerShell Sudo
-
Use
choco
to Install and Usesudo
on Windows PowerShell -
Use
sudo
EquivalentRunas
in Windows PowerShell
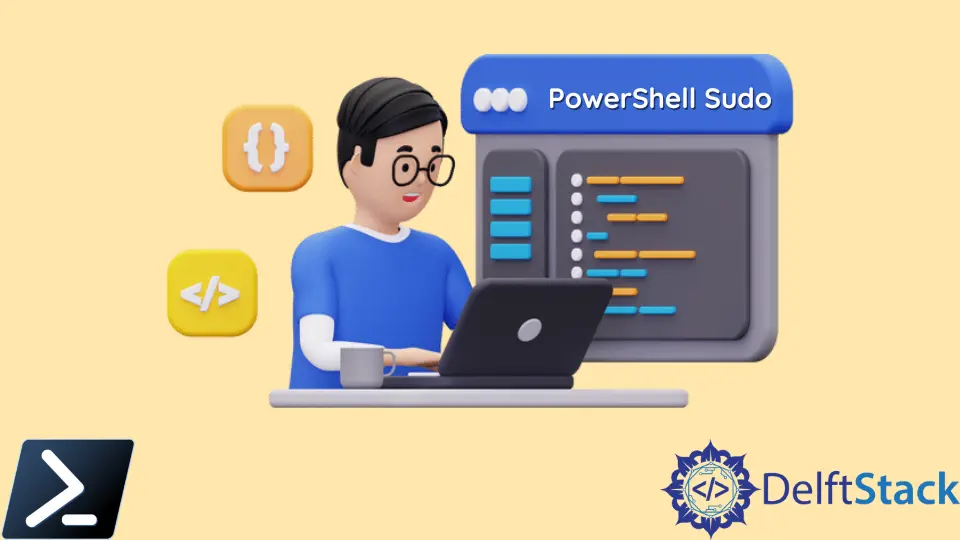
The sudo
command is one of the commonly used commands by Linux system administrators. It provides the root privileges to execute any command.
As a result, a normal user can completely control the system. But, PowerShell does not have sudo
to run commands as an administrator.
If the shell is opened in the normal user privilege, you must restart PowerShell using Run as administrator
. In Linux, you can use the sudo
command to execute commands as a root user.
This tutorial will teach you to use the sudo
command in Windows PowerShell.
Use choco
to Install and Use sudo
on Windows PowerShell
Chocolatey
is a free package manager for Windows to download and install softwares. Before using chocolatey in PowerShell, you will need to install it first.
You can run the command below to install the chocolatey
package. You will need to run PowerShell as an administrator to install any packages.
Set-ExecutionPolicy Bypass -Scope Process -Force; iex ((New-Object System.Net.WebClient).DownloadString('https://community.chocolatey.org/install.ps1'))
After installing the chocolatey
package, run this command to install the sudo
package.
choco install sudo
Now, you can use sudo
in Windows PowerShell like in Linux.
You do not have to click Run as Administrator
to open PowerShell and run commands that require administrative privileges.
For example, you can use sudo
with choco
to install a package without an elevated shell.
sudo choco install nano
Use sudo
Equivalent Runas
in Windows PowerShell
Runas
is a command-line tool in Windows that allows users to run programs with different user accounts. It is mostly used to run as an administrator account.
For example, this command will open Notepad as an administrator.
runas /user:administrator notepad
Similarly, you can run different commands using the Runas
command with an admin privilege. We hope this article helped you understand using sudo
in Windows PowerShell.