How to Split a String Into Separate Variables in PowerShell
-
Use the
Split()
Function to Split a String Into Separate Variables in PowerShell -
Use the
-Split
Flag to Split a String Into Separate Variables in PowerShell
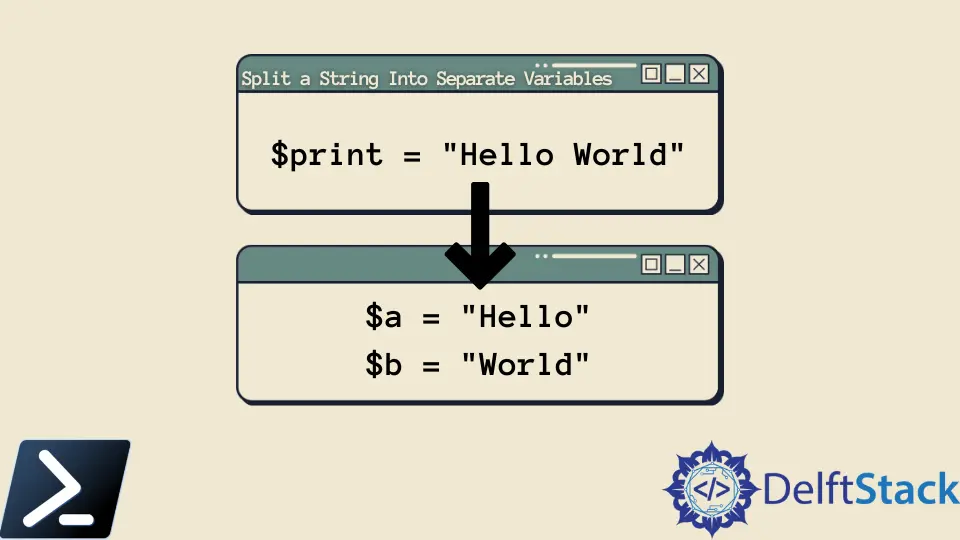
A string is the sequence of characters used to represent texts. It is one of the common data type in PowerShell.
You can define the string in PowerShell using single or double quotes. PowerShell string is created with the System.String
object type. This tutorial will introduce two methods to split a string into separate variables in PowerShell.
Use the Split()
Function to Split a String Into Separate Variables in PowerShell
The Split()
is a built-in function used to split a string in PowerShell. We can store the result of the Split()
function into multiple variables.
We have created a string variable $print
as shown below.
$print = "Hello World"
To split a string of $print
into two separate variables $a
and $b
, we can use:
$a, $b = $print.Split()
It splits the string on characters like spaces, line breaks, and tabs by default.
Check the data in variable $a
.
$a
Output:
Hello
Check the data in variable $b
.
$b
Output:
World
A delimiter is a character that marks the beginning or end of a data. You can specify a delimiter with single ' '
or double quotes " "
. It allows you to split the string on the desired character. The specified character will be removed from the resulting string.
For example, if you want to split on the letter l
, you can use:
$a, $b = $print.Split("l")
$a
Output:
He
You can also select the maximum number of strings to split into. For example, if you need to split the string into 3
parts, you can use:
$a, $b = $print.Split("l", 3)
Use the -Split
Flag to Split a String Into Separate Variables in PowerShell
The -Split
flag also splits a string in PowerShell, and the resulting string can be stored into separate variables. It is similar to the above method.
We have a string variable $new
.
$new = "Happy New Year."
To separate a string of $new
into separate variables, you can use the -Split
option like the command below.
$new1, $new2, $new3 = $new -Split " "
$new
Output:
Happy
Check other variables data in your PowerShell console to see the result. You can also try using different delimiters and strings.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell