How to Sort Object in PowerShell
-
Use the
Sort-Object
Cmdlet to Sort Directory Content in PowerShell -
Use the
Sort-Object
Cmdlet to Sort Array Elements in PowerShell
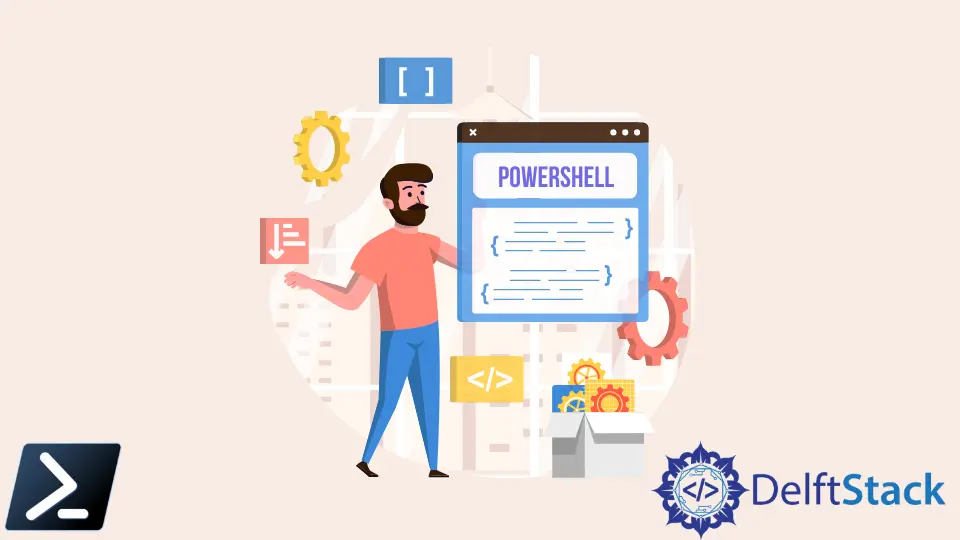
PowerShell has a handy cmdlet Sort-Object
to sort objects based on their property values. It can sort objects in ascending or descending order.
When no sort properties are specified in a command, it tries to use the default sort properties of the first input object. The objects to be sorted are piped to the Sort-Object
.
This tutorial will teach you to sort objects using PowerShell’s Sort-Object
cmdlet.
Use the Sort-Object
Cmdlet to Sort Directory Content in PowerShell
The following example sorts the files and sub-directories in a directory: C:\New
.
Get-ChildItem C:\New | Sort-Object
Output:
In the above command, the Get-ChildItem
cmdlet gets the files and subdirectories in a directory C:\New
. The output objects are piped to the Sort-Object
cmdlet, which sorts the content by the default property Name
.
You can use the -Property
parameter to specify the property names.
The following example sorts the files and sub-directories in the C:\New
directory by the property Length
.
Get-ChildItem C:\New | Sort-Object -Property Length
Output:
The default sorting order is the ascending order. So you must use the -Descending
parameter to sort the objects in descending order.
The following example sorts the content in a directory C:\New
in descending order based on the property LastWriteTime
.
Get-ChildItem C:\New | Sort-Object -Property LastWriteTime -Descending
Output:
Use the Sort-Object
Cmdlet to Sort Array Elements in PowerShell
The following command creates an array variable that contains multiple strings:
$array = "apple", "car", "book", "apple", "tree"
The following example sorts array strings in ascending order alphabetically:
$array | Sort-Object
Output:
apple
apple
book
car
tree
With the -Unique
parameter, you can remove the duplicate elements and get only the unique ones.
$array | Sort-Object -Unique
Output:
apple
book
car
tree
Similarly, you can store multiple numbers in an array and arrange them in ascending or descending order using Sort-Object
:
$num = 1, 2, 5, 7, 89, 54, 35
Next, pipe the array object to the Sort-Object
:
$num | Sort-Object
Output:
1
2
5
7
35
54
89
Now you should know how to sort objects and display them in order in PowerShell. See the documentation for Sort-Object
for more information.