How to Take a Screenshot Using PowerShell
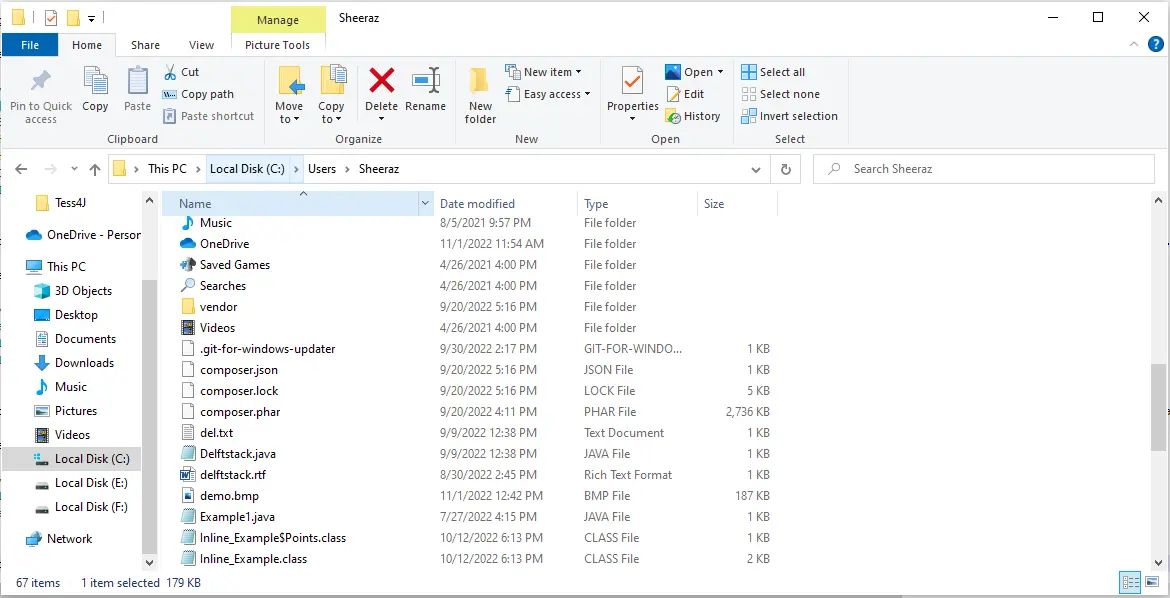
This tutorial demonstrates how to take a screenshot using PowerShell.
Take a Screenshot Using PowerShell
Taking a screenshot in PowerShell is a step-by-step process. This tutorial demonstrates taking a screenshot and then saving it as an image file.
Follow the steps below:
-
The first step is to take a screenshot and set its dimensions. Run the following commands to take a screenshot with the given dimensions:
[void][reflection.assembly]::loadwithpartialname("system.windows.forms") $DemoScreenshot = [System.Windows.Forms.SystemInformation]::VirtualScreen $WIDTH = $DemoScreenshot.Width $HEIGHT = $DemoScreenshot.Height $LEFTDIM = $DemoScreenshot.Left $TOPDIM = $DemoScreenshot.Top
The above code will take the screenshot using
reflection.assembly
andsystem.windows.forms
with the given dimensions width, height, left, and right. -
The next step is to create an image of the taken screenshot; we will save the screenshot as a
BMP
file. Run the following commands to create an image of the screenshot:$BitmapScreenshot = New-Object System.Drawing.Bitmap $WIDTH, $HEIGHT $FinalScreenshot = [System.Drawing.Graphics]::FromImage($BitmapScreenshot) $FinalScreenshot.CopyFromScreen($LEFTDIM, $TOPDIM, 0, 0, $BitmapScreenshot.Size)
The code above creates a new object as a bitmap image and then assigns the above-taken screenshot. Once the image is created, the next step is to save it in the given path.
-
The next thing to do is to save the screenshot to the given path. Run the following commands:
$FinalFile = 'c:\Users\Sheeraz\demo.bmp' $BitmapScreenshot.Save($FinalFile)
The code above will save the screenshot taken to the given path. See the output:
Output for the saved file, demo.bmp
:
The output of the screenshot:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook