How to Read JSON Files in PowerShell
-
Use
Get-Content
to Read JSON as a String in PowerShell -
Use
ConvertFrom-Json
to Read JSON as a Custom Object in PowerShell -
Use
Invoke-WebRequest
to Read JSON From Web in PowerShell
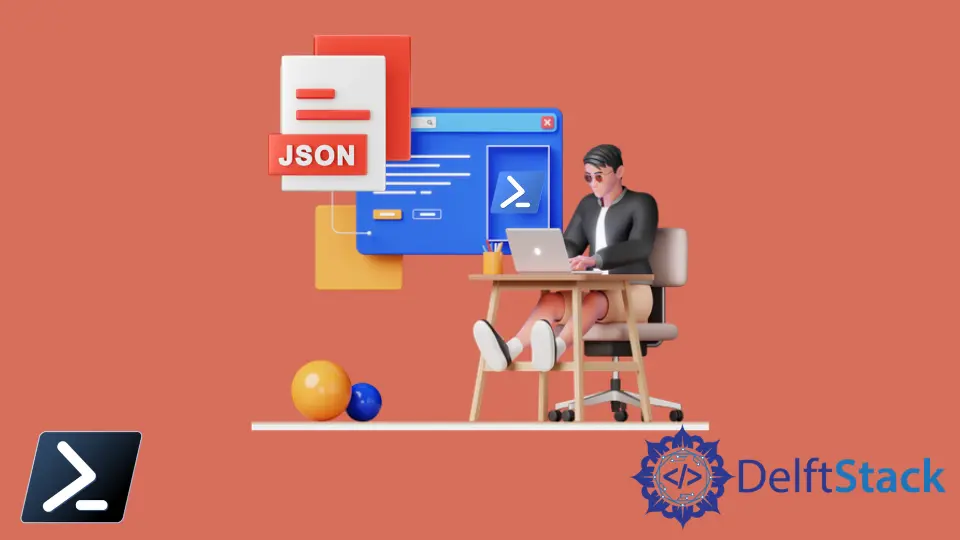
This tutorial demonstrates how to read JSON files in PowerShell.
Use Get-Content
to Read JSON as a String in PowerShell
The Get-Content
command is a PowerShell command that reads an item’s content. This command takes a Path
parameter that specifies the item’s location; the content is usually text data.
The JSON-formatted string is also text data; this command can read a JSON file and output it as a string to the shell.
The following command reads a JSON file as a string.
Get-Content "transactions.json"
Output:
[
{
"Date": "19/5/2022",
"ProductId": "0001",
"ProductName": "Fizzy Drink",
"Cost": "2.32",
"Currency": "USD"
},
{
"Date": "16/5/2022",
"ProductId": "0002",
"ProductName": "Dark Chocolate",
"Cost": "6.52",
"Currency": "USD"
},
{
"Date": "10/5/2022",
"ProductId": "0003",
"ProductName": "Snacks",
"Cost": "4.2",
"Currency": "USD"
}
]
Use ConvertFrom-Json
to Read JSON as a Custom Object in PowerShell
The ConvertFrom-Json
is a PowerShell command that converts a JSON-formatted string into a PowerShell custom object or PSCustomObject
. This object includes all the properties corresponding to the JSON fields.
The following command combines the Get-Content with the ConvertFrom-Json
to read a JSON as PSCustomObject
.
Get-Content "transactions.json" | ConvertFrom-Json
Output:
Date : 19/5/2022
ProductId : 0001
ProductName : Fizzy Drink
Cost : 2.32
Currency : USD
Date : 16/5/2022
ProductId : 0002
ProductName : Dark Chocolate
Cost : 6.52
Currency : USD
Date : 10/5/2022
ProductId : 0003
ProductName : Snacks
Cost : 4.2
Currency : USD
Use Invoke-WebRequest
to Read JSON From Web in PowerShell
JSON is a data interchange format commonly used on the web for client and server communications. A client sends an HTTP/HTTPS request to a server, and as a result, the server returns the requested data as JSON.
The Invoke-WebRequest
sends an HTTP request to a specified URI. The server receives the request and responds with a JSON accordingly.
The following command sends a web request to a server and parses the JSON with ConvertFrom-Json
.
Invoke-WebRequest -Uri https://my-json-server.typicode.com/typicode/demo/posts | ConvertFrom-Json
Output:
id title
-- -----
1 Post 1
2 Post 2
3 Post 3