How to Post Request in PowerShell
- Understanding POST Requests
- Using Invoke-RestMethod for POST Requests
- Using Invoke-WebRequest for More Control
- Sending JSON Data with POST Requests
- Conclusion
- FAQ
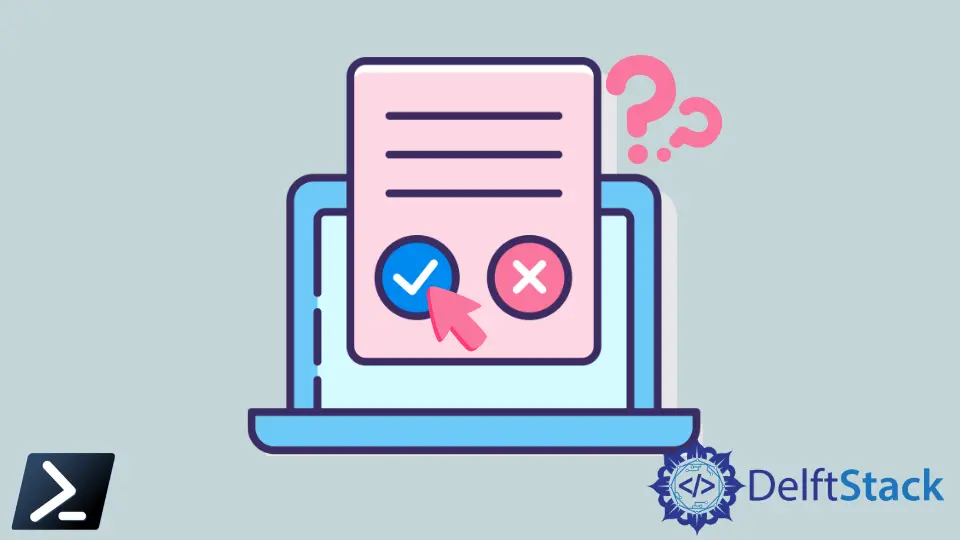
When it comes to interacting with web services, PowerShell is a powerful tool that can simplify the process of sending HTTP requests. One of the most common types of requests is the POST request, which allows you to send data to a server.
In this tutorial, we will delve into how to use POST in PowerShell to send parameters to a URI. Whether you’re automating tasks, integrating with APIs, or simply need to send data, mastering POST requests in PowerShell can significantly enhance your scripting capabilities. Let’s explore the various methods available to achieve this and provide practical examples that you can easily follow.
Understanding POST Requests
Before diving into the specifics of how to make POST requests in PowerShell, it’s essential to understand what a POST request is. Unlike GET requests that retrieve data from a server, POST requests are designed to send data to a server. This is particularly useful when you need to submit forms, upload files, or send data in JSON format.
POST requests can include various types of data, such as form fields, JSON objects, or XML. PowerShell provides several cmdlets and methods to facilitate these requests, making it easy for users to interact with web APIs.
Using Invoke-RestMethod for POST Requests
One of the most straightforward ways to send a POST request in PowerShell is by using the Invoke-RestMethod
cmdlet. This cmdlet allows you to easily send HTTP requests and handle the responses. Here’s how you can use it to send data to a specified URI.
$uri = "https://example.com/api/data"
$body = @{
name = "John Doe"
email = "john.doe@example.com"
}
$response = Invoke-RestMethod -Uri $uri -Method Post -Body $body -ContentType "application/json"
$response
Output:
{"success":true,"message":"Data received"}
In this example, we define a URI to which we want to send our data. The $body
variable is a hashtable containing the data we want to post, in this case, a name and email. The Invoke-RestMethod
cmdlet is then called with the specified URI, method, body, and content type. The server’s response is stored in the $response
variable, which we can output to see the result.
Using Invoke-RestMethod
is particularly advantageous because it automatically converts the response into a PowerShell object, making it easy to work with the returned data. This method is suitable for most API interactions, especially when dealing with JSON data.
Using Invoke-WebRequest for More Control
If you need more control over your HTTP requests, Invoke-WebRequest
is another powerful cmdlet in PowerShell. This cmdlet provides more options for handling the request and response, making it ideal for more complex scenarios.
$uri = "https://example.com/api/data"
$body = @{
name = "Jane Doe"
email = "jane.doe@example.com"
}
$response = Invoke-WebRequest -Uri $uri -Method Post -Body $body -ContentType "application/json"
$response.Content
Output:
{"success":true,"message":"Data received"}
In this example, we again define our URI and body. The key difference is that we are using Invoke-WebRequest
, which allows for more extensive customization of the request. After sending the POST request, we can access the content of the response directly through the $response.Content
property. This method is particularly useful when you need to handle raw response data or when working with APIs that return HTML or XML.
Sending JSON Data with POST Requests
In many modern applications, APIs expect data to be sent in JSON format. PowerShell makes it easy to convert your data into JSON before sending it in a POST request. Here’s how to accomplish that.
$uri = "https://example.com/api/data"
$body = @{
name = "Alice Smith"
email = "alice.smith@example.com"
}
$jsonBody = $body | ConvertTo-Json
$response = Invoke-RestMethod -Uri $uri -Method Post -Body $jsonBody -ContentType "application/json"
$response
Output:
{"success":true,"message":"Data received"}
In this code snippet, we first define our URI and data as a hashtable. We then use the ConvertTo-Json
cmdlet to convert our hashtable into a JSON string, which is necessary for the API to understand the data format. The Invoke-RestMethod
cmdlet is then used to send the POST request, just as before. This method is particularly useful when working with APIs that require JSON-formatted data.
Conclusion
In this tutorial, we explored how to send POST requests in PowerShell using the Invoke-RestMethod
and Invoke-WebRequest
cmdlets. We learned how to send both hashtable and JSON data to a specified URI, making it easier to interact with web APIs. Understanding these methods will enhance your ability to automate tasks and integrate with various online services. As you continue to work with PowerShell, mastering HTTP requests will undoubtedly open up new possibilities for your scripting endeavors.
FAQ
-
What is a POST request?
A POST request is a type of HTTP request used to send data to a server, often for submitting forms or uploading files. -
How do I send JSON data in PowerShell?
You can send JSON data in PowerShell by converting your data to a JSON string using the ConvertTo-Json cmdlet and then using Invoke-RestMethod or Invoke-WebRequest to send the request. -
What is the difference between Invoke-RestMethod and Invoke-WebRequest?
Invoke-RestMethod is simpler and automatically converts the response to a PowerShell object, while Invoke-WebRequest provides more control over the request and response handling. -
Can I send files using POST requests in PowerShell?
Yes, you can send files using POST requests by including the file data in the body of the request, typically using the -InFile parameter with Invoke-WebRequest. -
How can I troubleshoot POST requests in PowerShell?
You can troubleshoot POST requests by checking the response status code, examining error messages, and using tools like Fiddler to monitor HTTP traffic.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.