PowerShell Mandatory Parameter With Default Value
- Understanding Mandatory Parameters
- Setting Default Values for Mandatory Parameters
- Best Practices for Using Mandatory Parameters with Default Values
- Conclusion
- FAQ
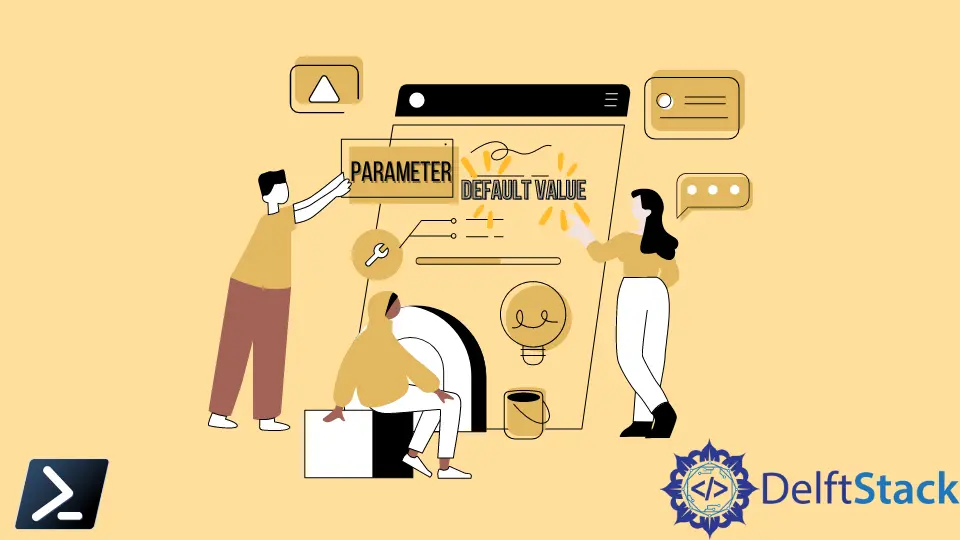
In the world of PowerShell, understanding how to work with parameters is crucial for creating efficient scripts. One interesting feature is the ability to set mandatory parameters with default values. This allows you to enforce user input while still providing a fallback option if no input is given.
In this tutorial, we will explore how to define mandatory parameters in PowerShell and how to assign them default values. This knowledge can significantly streamline your scripting process, making your scripts more robust and user-friendly. Whether you’re a PowerShell novice or an experienced user, this guide will enhance your scripting skills and help you create more effective PowerShell commands.
Understanding Mandatory Parameters
Mandatory parameters are essential components of PowerShell functions and scripts. They require the user to provide a value when the function is called. This ensures that the function has all the necessary information to execute correctly. However, there are scenarios where you might want to provide a default value for these parameters. This way, if the user does not specify a value, the function can still operate using the default.
To define a mandatory parameter with a default value in PowerShell, you can use the Parameter
attribute. This attribute allows you to specify various characteristics of the parameter, including whether it is mandatory and what its default value should be. Here’s a simple example:
function Test-MandatoryParameter {
param (
[Parameter(Mandatory=$true)]
[string]$Name = "DefaultName"
)
"Hello, $Name!"
}
Test-MandatoryParameter
Output:
Hello, DefaultName!
In this example, we define a function called Test-MandatoryParameter
. The Name
parameter is marked as mandatory, but it also has a default value of “DefaultName”. When you call the function without providing a name, it uses the default value, demonstrating how mandatory parameters can work alongside default values.
Setting Default Values for Mandatory Parameters
When defining mandatory parameters in PowerShell, you might wonder how to set default values effectively. This capability can be particularly useful in scenarios where you want to ensure that your scripts still function even if the user forgets to provide a necessary argument.
Here’s how you can set default values for mandatory parameters:
function Greet-User {
param (
[Parameter(Mandatory=$true)]
[string]$FirstName,
[Parameter(Mandatory=$true)]
[string]$LastName = "Doe"
)
"Hello, $FirstName $LastName!"
}
Greet-User -FirstName "John"
Output:
Hello, John Doe!
In this code, the Greet-User
function has two parameters: FirstName
and LastName
. While FirstName
is mandatory, LastName
is also marked as mandatory but has a default value of “Doe”. When the function is called with just the FirstName
, it uses “Doe” as the last name, showcasing how you can provide flexibility in your scripts while still enforcing required inputs.
Best Practices for Using Mandatory Parameters with Default Values
Using mandatory parameters with default values can enhance the usability and robustness of your PowerShell scripts. However, there are best practices to consider to ensure your scripts remain clear and effective.
-
Clarity: Always ensure that the purpose of the parameter is clear. If a parameter has a default value, it should be documented so users know what to expect if they do not provide a value.
-
Validation: Use validation attributes to ensure that the input meets specific criteria. For example, you can use
[ValidateSet()]
to restrict the values that can be passed to a parameter. -
Error Handling: Implement error handling to manage cases where users provide invalid input or none at all. This can help prevent your script from failing unexpectedly.
Here’s an example that incorporates these best practices:
function Set-UserRole {
param (
[Parameter(Mandatory=$true)]
[string]$Username,
[Parameter(Mandatory=$true)]
[ValidateSet("Admin", "User", "Guest")]
[string]$Role = "User"
)
"Setting role of $Username to $Role."
}
Set-UserRole -Username "Alice"
Output:
Setting role of Alice to User.
In this example, the Set-UserRole
function requires a Username
and allows the Role
parameter to default to “User”. The Role
parameter is validated to ensure it can only be one of the specified values, improving the reliability of the script.
Conclusion
Incorporating mandatory parameters with default values in PowerShell can significantly enhance your scripts’ functionality and user experience. By understanding how to define these parameters effectively, you can create scripts that are both flexible and robust. Remember to follow best practices, such as clear documentation and input validation, to ensure your scripts are user-friendly and error-resistant. Whether you’re automating tasks or developing complex scripts, mastering this feature will undoubtedly elevate your PowerShell skills.
FAQ
-
What is a mandatory parameter in PowerShell?
A mandatory parameter is a required input for a function or script that must be provided for it to execute correctly. -
Can a mandatory parameter have a default value?
Yes, a mandatory parameter can have a default value, allowing the script to operate with that value if no input is provided. -
How do I define a mandatory parameter in PowerShell?
You can define a mandatory parameter using theParameter
attribute with theMandatory
property set to$true
. -
What happens if a user does not provide a mandatory parameter value?
If a user does not provide a value for a mandatory parameter, PowerShell will prompt them to enter a value before the script can proceed. -
Are there best practices for using mandatory parameters with default values?
Yes, best practices include ensuring clarity in documentation, implementing validation, and handling errors gracefully.