How to Export Output to a File Using PowerShell
-
Introduction to
Out-File
Command in PowerShell -
Use the
-append
Parameter to Append to a File in PowerShell - Change the Output Data in PowerShell
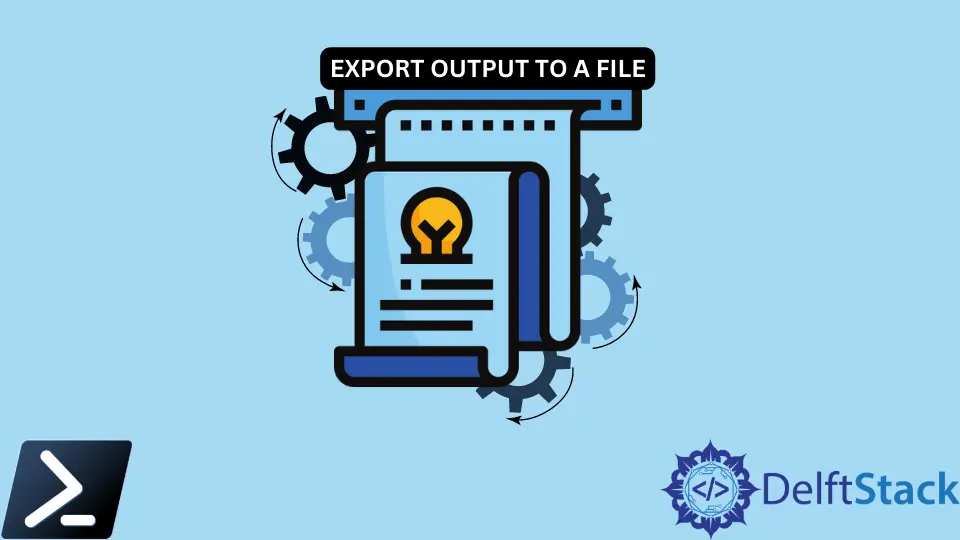
Multiple scripting languages have functions or methods to let you export the data to a file. Even in earlier MS-DOS days, the legacy redirection operator (>
) can conveniently do this.
This article will discuss how to export output data to a file, add data to an existing file, and manipulate output using PowerShell.
Introduction to Out-File
Command in PowerShell
Let us say you have a script that returns a list of all of the Windows services on your computer. When we run Get-Service
, you will receive all of the objects you might expect on the console.
But maybe we would like to save that output to a text file. The Out-File
command is a great way to do that.
We can use Out-File
by piping just about anything via the pipeline operator. Below, we can see that the script sent the output of Get-Service
to the cmdlet, which created a text file called Services.txt
containing the same display on the console.
Example Code:
Get-Service | Out-File -FilePath C:\PS\Services.txt
Get-Content C:\PS\Services.txt
Output:
Status Name DisplayName
Stopped AJRouter AllJoyn Router Service
Stopped ALG Application Layer Gateway Service
<SNIP>
Use the -append
Parameter to Append to a File in PowerShell
By default, the Out-File
command overwrites anything in the text file provided via the -FilePath
parameter. However, we can override this behavior using the -Append
parameter.
Perhaps we are accumulating console output in a file and would like to append text to a file rather than overwriting. The -Append
parameter is your best friend.
If we do not use the Out-File -Append
parameter, the C:\PS\File.txt
contents are overwritten. But, as soon as we add the -Append
parameter, it appends the output to the end.
Example Code:
'firststring' | Out-File -FilePath C:\PS\File.txt
Get-Content -Path C:\PS\File.txt
'secondstring' | Out-File -FilePath C:\PS\File.txt -Append
Get-Content C:\PS\File.txt
Output:
firststring
firststring
secondstring
Change the Output Data in PowerShell
By default, this cmdlet will attempt to replicate what’s shown on the console, but there are some ways to manipulate it. For example, the cmdlet has a -NoNewLine
parameter that removes all newline characters.
Example Code:
Get-Service | Out-File -FilePath C:\PS\Services.txt -NoNewline
Get-Content C:\PS\Services.txt
Output:
Status Name DisplayName
------ ---- -----------
Stopped AJRouter AllJoyn Router Service
Stopped ALG Application La
Or, we can cut off the text on each row at a particular character count with the -Width
parameter.
Example Code:
Get-Service | Out-File -FilePath C:\PS\Services.txt -Width 30
Get-Content C:\PS\Services.txt
Output:
Status Name DisplayName
------ ----- -----------
Stopped AJRouter Al
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn