The Or Statement in PowerShell
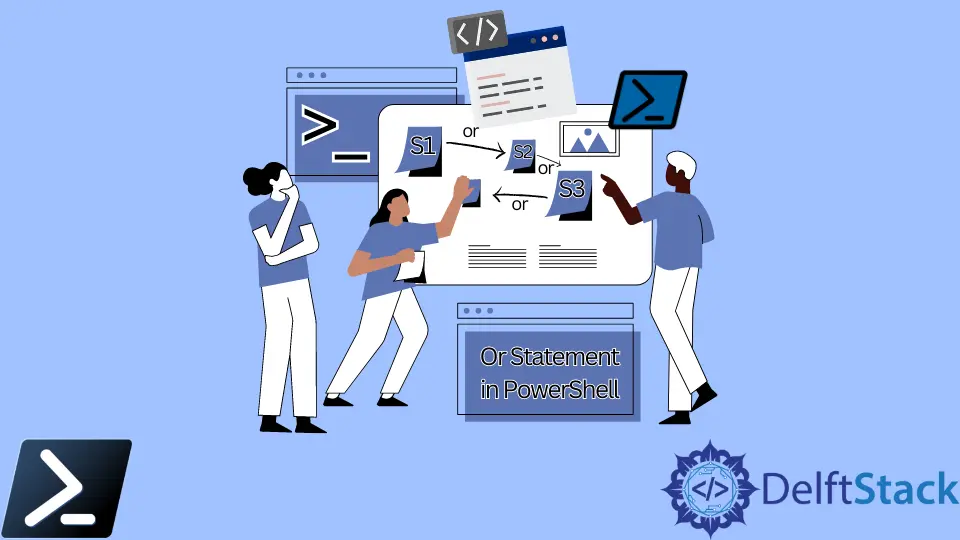
The logical operators connect expressions and statements in the PowerShell. It allows you to use a single expression for multiple conditions. The supported logical operators in the PowerShell are -and
, -or
, -xor
, -not
, and !
.
For example, the below statement uses the -and
operator and the -or
operator to connect three conditions. The statement is true only when the value of $a
is less than $b
, and either $a is equal to 10 or $b is greater than 10.
($a -lt $b) -and (($a -eq 10)) -or ($b -gt 10)
-lt
, -gt
, and -eq
are comparison operators in the PowerShell, which indicate less than
, greater than
, and equals
respectively. The comparison operator allows you to compare values in the PowerShell. The PowerShell also includes other comparison operators such as -ne
, -ge
, -le
, -like
, -notlike
, -match
, -notmatch
, -replace
, -contains
, etc.
This tutorial will teach you to use -or
operator in the PowerShell.
Use the -or
Operator for the Statement in the PowerShell
The -or
operator, also known as logical OR
in the PowerShell, returns the Boolean value True
when either condition is true.
Consider, we have two variables $a=10
and $b=20
. The following statement returns True
because one condition is True
: the value of $a
is less than the value of $b
.
($a -eq $b) -or ($a -lt $b)
Output:
True
When none of the conditions are true, it returns the False
.
($a -eq $b) -or ($a -gt $b)
Output:
False