PowerShell Multidimensional Arrays
- What Are Multidimensional Arrays?
- Creating a Multidimensional Array
- Accessing Elements in a Multidimensional Array
- Iterating Through a Multidimensional Array
- Practical Applications of Multidimensional Arrays
- Conclusion
- FAQ
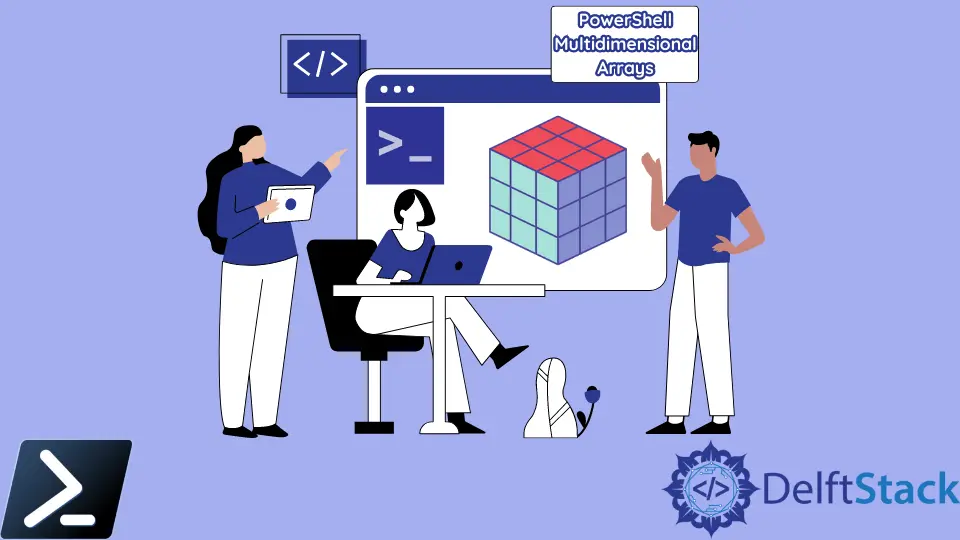
Multidimensional arrays in PowerShell can be a game-changer for managing complex data structures. If you’re looking to store and manipulate data in a more organized way, understanding multidimensional arrays is essential.
This tutorial will introduce you to the concept of multidimensional arrays in PowerShell, guiding you through their creation, manipulation, and practical applications. Whether you’re a beginner or someone looking to enhance your PowerShell skills, this article will provide you with the insights you need to effectively use multidimensional arrays in your scripts. Let’s dive into this powerful feature of PowerShell!
What Are Multidimensional Arrays?
Multidimensional arrays are arrays that allow you to store data in a grid-like structure, organizing information in rows and columns. Unlike single-dimensional arrays, which hold a list of items, multidimensional arrays can hold multiple lists of items, making them ideal for representing tables, matrices, or any structured data.
In PowerShell, you can create a multidimensional array using the [,]
syntax. For example, a two-dimensional array can be visualized as a table with rows and columns. This structure is particularly useful for scenarios such as managing data from a database, organizing configuration settings, or even storing user inputs in a structured manner.
Creating a Multidimensional Array
To create a multidimensional array in PowerShell, you can use the following syntax:
$multiArray = @(
@(1, 2, 3),
@(4, 5, 6),
@(7, 8, 9)
)
Output:
1 2 3
4 5 6
7 8 9
In this example, we created a two-dimensional array with three rows and three columns. Each inner array represents a row in the multidimensional array. You can access elements in the array using their indices, where the first index represents the row and the second index represents the column. For instance, $multiArray[1, 2]
would return the value 6
, which is located in the second row and third column.
This approach allows you to easily manage and retrieve data. You can also expand this concept to create larger arrays or even three-dimensional arrays, depending on your requirements.
Accessing Elements in a Multidimensional Array
Once you have your multidimensional array set up, accessing elements is straightforward. Here’s how you can retrieve and manipulate data:
$firstElement = $multiArray[0, 0]
$secondElement = $multiArray[1, 1]
$multiArray[2, 2] = 10
Output:
1
5
10
In this snippet, we accessed the first element, which is 1
, and the second element, which is 5
. We also modified the value of the last element in the array to 10
. This flexibility is one of the key advantages of using multidimensional arrays. You can easily update, retrieve, or manipulate data as needed.
Iterating Through a Multidimensional Array
To fully leverage the power of multidimensional arrays, you often need to iterate through them. PowerShell provides various ways to loop through arrays, and here’s a common approach using a nested loop:
for ($i = 0; $i -lt $multiArray.GetLength(0); $i++) {
for ($j = 0; $j -lt $multiArray.GetLength(1); $j++) {
Write-Host $multiArray[$i, $j]
}
}
Output:
1
2
3
4
5
6
7
8
9
In this example, we used two nested for
loops to iterate through the rows and columns of the multidimensional array. The GetLength(0)
method gives us the number of rows, while GetLength(1)
provides the number of columns. Inside the loops, we simply print each element to the console. This technique is useful when you need to process or display all the data stored within the array.
Practical Applications of Multidimensional Arrays
Multidimensional arrays can be applied in various scenarios, such as data analysis, configuration management, and more. For instance, if you’re working on a script that processes user data, you could use a multidimensional array to store user attributes like names, ages, and email addresses.
Here’s a quick example:
$userData = @(
@("Alice", 30, "alice@example.com"),
@("Bob", 25, "bob@example.com"),
@("Charlie", 35, "charlie@example.com")
)
for ($i = 0; $i -lt $userData.GetLength(0); $i++) {
Write-Host "Name: $($userData[$i, 0]), Age: $($userData[$i, 1]), Email: $($userData[$i, 2])"
}
Output:
Name: Alice, Age: 30, Email: alice@example.com
Name: Bob, Age: 25, Email: bob@example.com
Name: Charlie, Age: 35, Email: charlie@example.com
In this example, we created a multidimensional array to store user information. The script iterates through the array and displays each user’s details in a readable format. Such applications demonstrate the versatility of multidimensional arrays in organizing and managing data efficiently.
Conclusion
Multidimensional arrays in PowerShell offer a robust way to handle complex data structures. By understanding how to create, access, and manipulate these arrays, you can significantly enhance your scripting capabilities. Whether you’re managing user data, processing configurations, or performing data analysis, multidimensional arrays can help you keep your information organized and easily accessible. Start experimenting with multidimensional arrays today, and watch your PowerShell skills flourish!
FAQ
-
What is a multidimensional array in PowerShell?
A multidimensional array is an array that allows you to store data in a grid-like structure, organizing information in rows and columns. -
How do I create a multidimensional array in PowerShell?
You can create a multidimensional array using the[,]
syntax or by nesting arrays within an array. -
How do I access elements in a multidimensional array?
You can access elements using their indices, where the first index represents the row and the second represents the column.
-
Can I modify elements in a multidimensional array?
Yes, you can easily modify elements by accessing them using their indices and assigning new values. -
What are some practical uses for multidimensional arrays?
Multidimensional arrays can be used for data analysis, configuration management, and storing structured data, such as user attributes.