PowerShell Equivalent of Linux mkdir Command
- Understanding the mkdir Command
- Basic Directory Creation with New-Item
- Creating Multiple Directories
- Creating Parent Directories
- Conclusion
- FAQ
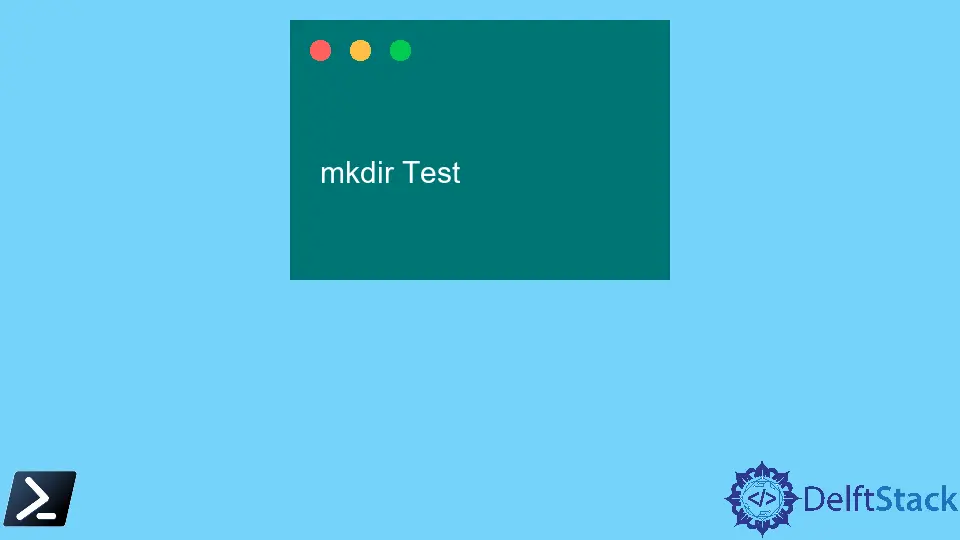
Creating directories is a fundamental task in both Linux and Windows environments. If you’re transitioning from Linux to PowerShell, you might be wondering how to replicate the functionality of the mkdir
command.
In this tutorial, we’ll explore the PowerShell equivalent of the Linux mkdir
command, providing you with clear examples and explanations. Whether you’re managing files on a local machine or working with a remote server, understanding how to create directories in PowerShell will streamline your workflow. Let’s dive in and learn how to harness the power of PowerShell for directory management.
Understanding the mkdir Command
In Linux, the mkdir
command is used to create new directories. It is straightforward and can be used with various options to customize its behavior. For instance, you can create multiple directories at once or create parent directories if they don’t exist. PowerShell has a similar command that allows you to achieve the same functionality, albeit with a different syntax.
In PowerShell, the command to create a directory is New-Item
. You can use this command to create new folders just like you would with mkdir
in Linux. Let’s explore how to use this command effectively.
Basic Directory Creation with New-Item
To create a new directory in PowerShell, you can use the New-Item
cmdlet. This command is quite versatile and can create files, folders, and other types of items. Here’s a simple example of how to create a directory.
New-Item -Path "C:\example\newfolder" -ItemType Directory
Output:
Directory: C:\example
Mode LastWriteTime Length Name
---- ------------- ------ ----
d----- 10/10/2023 10:00 AM newfolder
In this example, we used New-Item
with the -Path
parameter to specify the location of the new folder. The -ItemType Directory
parameter tells PowerShell that we want to create a directory. If the specified path already exists, PowerShell will throw an error, so make sure to choose a unique name or check for existing directories before executing the command.
Creating Multiple Directories
Just like the Linux mkdir
command, PowerShell allows you to create multiple directories in one go. This can be particularly useful when you need to set up a directory structure quickly. Here’s how you can do it using New-Item
in PowerShell.
$folders = @("C:\example\folder1", "C:\example\folder2", "C:\example\folder3")
foreach ($folder in $folders) {
New-Item -Path $folder -ItemType Directory
}
Output:
Directory: C:\example
Mode LastWriteTime Length Name
---- ------------- ------ ----
d----- 10/10/2023 10:05 AM folder1
d----- 10/10/2023 10:05 AM folder2
d----- 10/10/2023 10:05 AM folder3
In this example, we created an array of folder paths that we want to create. The foreach
loop iterates over each folder path and executes the New-Item
command for each one. This method is efficient and saves time, especially when setting up complex directory structures.
Creating Parent Directories
One of the powerful features of the Linux mkdir
command is the ability to create parent directories if they do not exist using the -p
option. In PowerShell, you can achieve a similar result by using the -Force
parameter with New-Item
. This allows you to create a directory structure without worrying about whether the parent directories already exist.
New-Item -Path "C:\example\parent\child" -ItemType Directory -Force
Output:
Directory: C:\example\parent
Mode LastWriteTime Length Name
---- ------------- ------ ----
d----- 10/10/2023 10:10 AM child
In this command, we specified a path that includes both a parent and child directory. The -Force
parameter ensures that PowerShell creates the entire directory structure, even if the parent directory does not exist. This is particularly useful for organizing files and directories in a structured manner.
Conclusion
Understanding how to create directories in PowerShell is essential for anyone working in a Windows environment, especially if you are coming from a Linux background. The New-Item
cmdlet provides a robust way to manage directories, allowing for basic creation, multiple directory setups, and even parent directory creation with ease. By mastering these commands, you’ll enhance your productivity and streamline your workflow when managing files and directories.
FAQ
- What is the PowerShell equivalent of the Linux mkdir command?
The PowerShell equivalent is theNew-Item
cmdlet with the-ItemType Directory
parameter.
-
Can I create multiple directories at once in PowerShell?
Yes, you can use a loop to create multiple directories in PowerShell. -
How do I create parent directories if they do not exist?
You can use the-Force
parameter withNew-Item
to create parent directories automatically. -
Is there a way to check if a directory already exists before creating it?
Yes, you can use theTest-Path
cmdlet to check if a directory exists before attempting to create it. -
Can I create directories on a remote server using PowerShell?
Yes, you can use PowerShell remoting to create directories on a remote server.