How to Install MSI File in PowerShell
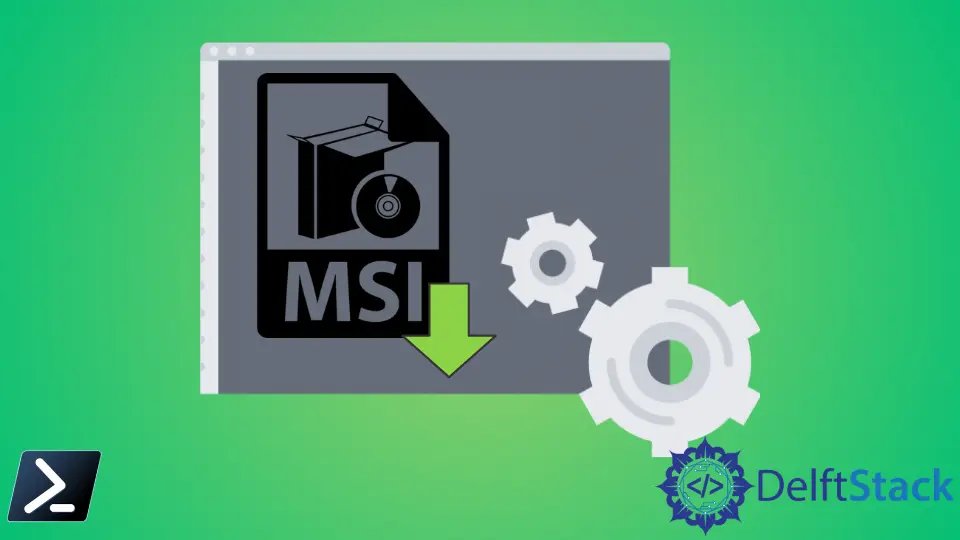
PowerShell is a Microsoft tool that can be used for scripting purposes in Windows, Linux, and Mac users. There are different versions of PowerShell for different operators.
For Windows users, with Windows Operating Systems above Windows XP, PowerShell comes installed in default. However, other users can download the application from the Microsoft site for PowerShell if required.
Advanced scripting purposes can be achieved by using PowerShell tools by professionals. These can be used as automated tools and for Configuration Management of CI\CD-based systems.
Install MSI File in PowerShell
This article will discuss installing other applications with the PowerShell application scripting platform. PowerShell supports different operating system purposes, including installing .exe
and other similar applications by invoking files and functions as a cross-platform application.
MSI
is a Microsoft Installation package file format by Windows, derived from Microsoft Installer. Similar to the .exe
file format, .msi
is a supporting file type for installation storage and uninstallation of programs.
Similar to .exe
files, .msi
files can also be executed through PowerShell. Users can easily install a .msi
file with a few different methods inside the PowerShell script.
Invoke the .msi
File by Calling the Path and the File in PowerShell
The simplest way to achieve this requirement would be by invoking the file directly through the PowerShell script. Following is the code that would be used for the mentioned purpose.
Start-Process FirefoxSetup14.0.1.msi
Following is the screenshot of the execution and the output as the .msi
file for Firefox is used for installation.
There are other methods, such as msiexec
and install-package
, etc. Those methods can be used in the installation or .msi
execution process.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.