How to Extract a Column From a CSV File and Store It in a Variable in PowerShell
- Understanding CSV Files and PowerShell
- Extracting a Column Using PowerShell
- Working with Multiple Columns
- Filtering Data While Extracting
- Conclusion
- FAQ
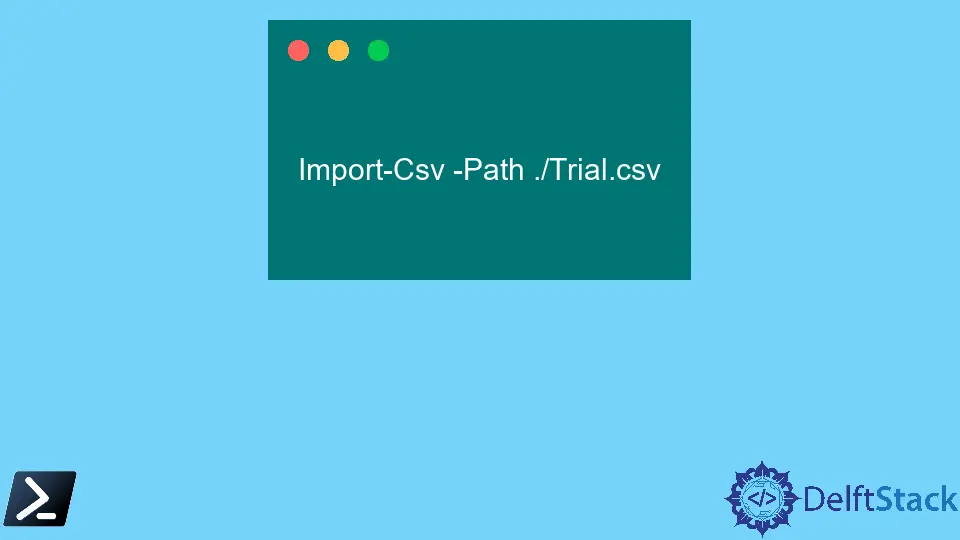
Extracting data from CSV files is a common task for system administrators and data analysts alike. Whether you need to manipulate data for reporting or simply want to automate a process, PowerShell offers a straightforward way to handle this.
In this article, we will explore how to extract a specific column from a CSV file and store it in a variable using PowerShell. By utilizing the Import-Csv cmdlet, we can convert a comma-separated value file into a table of custom objects, making it easy to work with specific columns. Let’s dive into the methods and see how you can streamline your workflow with PowerShell.
Understanding CSV Files and PowerShell
CSV (Comma-Separated Values) files are widely used for storing tabular data. Each line in a CSV file represents a row, with columns separated by commas. PowerShell, a powerful scripting language, makes it easy to interact with these files. The Import-Csv cmdlet is particularly useful as it reads a CSV file and converts it into a collection of custom objects. This allows you to access individual columns easily.
To get started, ensure you have a CSV file ready. For our example, let’s assume you have a file named data.csv
containing the following columns: Name, Age, and Email.
Extracting a Column Using PowerShell
To extract a specific column from a CSV file, you can use the Import-Csv cmdlet followed by a property selection. Let’s say we want to extract the “Email” column from our CSV file. Here’s how you can do it:
$data = Import-Csv -Path "data.csv"
$emailColumn = $data.Email
Output:
user1@example.com
user2@example.com
user3@example.com
In this code snippet, we first import the CSV file using the Import-Csv cmdlet and store the resulting custom objects in the variable $data
. Next, we extract the “Email” column by accessing the property directly. The result is stored in the variable $emailColumn
, which now contains all the email addresses from the CSV file. This method is efficient and allows you to easily manipulate or use the extracted data in further operations.
Working with Multiple Columns
Sometimes, you may want to extract more than one column from a CSV file. PowerShell allows you to do this seamlessly. Suppose we want to extract both the “Name” and “Email” columns. Here’s how we can achieve that:
$data = Import-Csv -Path "data.csv"
$selectedColumns = $data | Select-Object Name, Email
Output:
Name Email
---- -----
user1 user1@example.com
user2 user2@example.com
user3 user3@example.com
In this example, we use the Select-Object
cmdlet to specify the columns we want to extract. The result is stored in the $selectedColumns
variable, which now contains both the “Name” and “Email” columns. This approach is particularly useful when you need to work with multiple data points simultaneously. You can further manipulate this data, export it to another file, or use it in reports.
Filtering Data While Extracting
Another powerful feature of PowerShell is the ability to filter data while extracting columns. For instance, if you only want to extract emails for users older than 30, you can combine filtering with column extraction. Here’s how you can do that:
$data = Import-Csv -Path "data.csv"
$filteredEmails = $data | Where-Object { $_.Age -gt 30 } | Select-Object -ExpandProperty Email
Output:
user2@example.com
In this code, we first import the CSV file and store it in $data
. Then we use the Where-Object
cmdlet to filter the data, selecting only those users whose age is greater than 30. Finally, we use Select-Object -ExpandProperty
to extract just the “Email” property from the filtered results. The resulting email addresses are stored in the $filteredEmails
variable. This method is particularly effective for data analysis and reporting, allowing you to focus on specific subsets of your data.
Conclusion
Extracting columns from a CSV file in PowerShell is a straightforward process that can significantly enhance your data management capabilities. By using the Import-Csv cmdlet, you can easily import data, select specific columns, and even filter results based on conditions. Whether you are managing user data or analyzing trends, these techniques will help you streamline your workflows. With PowerShell, you can efficiently manipulate data and automate repetitive tasks, saving you time and effort in your daily operations.
FAQ
-
How do I import a CSV file in PowerShell?
You can import a CSV file using the Import-Csv cmdlet followed by the path to your file. -
Can I extract multiple columns at once?
Yes, you can use the Select-Object cmdlet to specify multiple columns to extract from the imported data. -
What if my CSV file has a different delimiter?
If your CSV file uses a different delimiter, you can specify it using the-Delimiter
parameter in the Import-Csv cmdlet. -
How do I filter data while extracting columns?
You can use the Where-Object cmdlet to filter data based on specific conditions before extracting the desired columns. -
Can I export the extracted data to a new CSV file?
Yes, you can use the Export-Csv cmdlet to save the extracted data to a new CSV file.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn