How to Check if a String Is Not NULL or EMPTY in PowerShell
- Use a Conditional Statement to Check if a String Variable Is Not Null or Empty in PowerShell
-
Use the
IsNullorEmpty
Method to Check if a String Variable Is Not Null or Empty in PowerShell -
Use the
IsNullOrWhiteSpace
Method to Check if a String Variable Is Not Null or Empty in PowerShell -
Use the
$null
Variable to Check if a String Variable Is Not Null or Empty in PowerShell
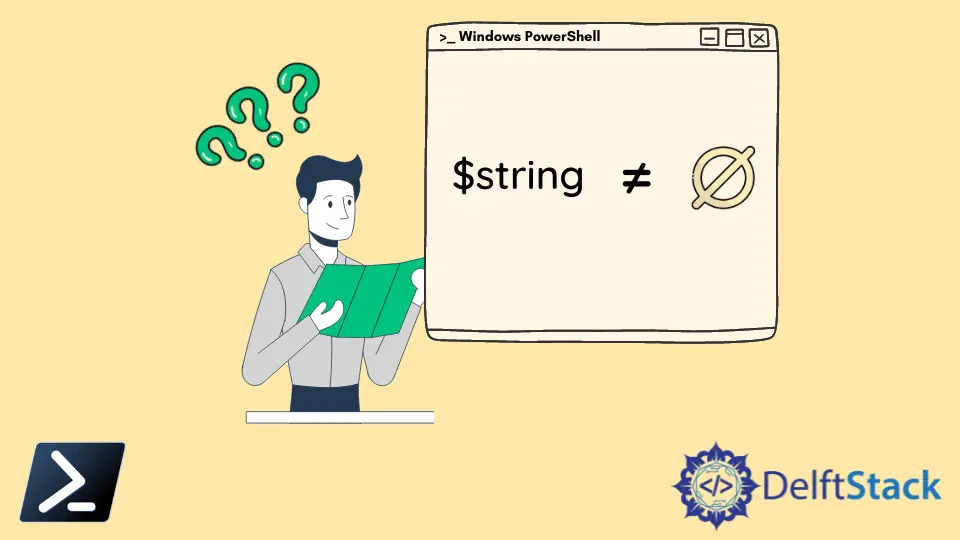
A string is the sequence of characters used to represent texts. You can define the string using single or double quotes in PowerShell.
While working with string variables in PowerShell, sometimes you might need to check whether a string variable is null or empty. This tutorial will introduce different methods to check if a string variable is not null or empty in PowerShell.
Use a Conditional Statement to Check if a String Variable Is Not Null or Empty in PowerShell
We have created a string variable, $string
.
$string = "Hello World"
The following example checks if a $string
variable is null or not in PowerShell. It returns the first statement if the variable is not null or empty and the second statement if the variable is null or empty.
if ($string) {
Write-Host "The variable is not null."
}
else {
Write-Host "The variable is null."
}
Output:
The variable is not null.
Let’s assign an empty string value to a variable and check again. If a variable is not assigned, it also has a null value.
$string = ""
if ($string) {
Write-Host "The variable is not null."
}
else {
Write-Host "The variable is null."
}
Output:
The variable is null.
White space characters are not considered null string values.
Use the IsNullorEmpty
Method to Check if a String Variable Is Not Null or Empty in PowerShell
You can use the .NET class System.String
to check if a string variable is null or empty in PowerShell. The IsNullorEmpty()
method indicates whether the specified string is empty or null.
It returns True
if the string is empty and False
if it is not empty.
[string]::IsNullOrEmpty($new)
Output:
True
Now, let’s assign a string value to a variable.
$new = "asdf"
[string]::IsNullOrEmpty($new)
Output:
False
Use the IsNullOrWhiteSpace
Method to Check if a String Variable Is Not Null or Empty in PowerShell
You can also use the IsNullOrWhiteSpace
method to check if a string variable is not null or empty in PowerShell. This method only works from PowerShell 3.0.
It returns True
if the variable is null or empty or contains white space characters. If not, it prints False
in the output.
[string]::IsNullOrWhiteSpace($str)
Output:
True
Assign a string value to a variable.
$str = "Have a nice day."
[string]::IsNullOrWhiteSpace($str)
Output:
False
Use the $null
Variable to Check if a String Variable Is Not Null or Empty in PowerShell
$null
is one of the automatic variables in PowerShell, which represents NULL. You can use the -eq
parameter to check if a string variable equals $null
.
It returns True
if the variable is equal to $null
and False
if the variable is not equal to $null
.
$str -eq $null
Output:
False
We can use any of the methods above and easily determine whether a string variable is not null or empty in PowerShell.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell