How to Use the Get-Clipboard Output in PowerShell
- Understanding Get-Clipboard in PowerShell
- Storing Clipboard Data in a Variable
- Manipulating Clipboard Data
- Exporting Clipboard Data to a File
- Conclusion
- FAQ
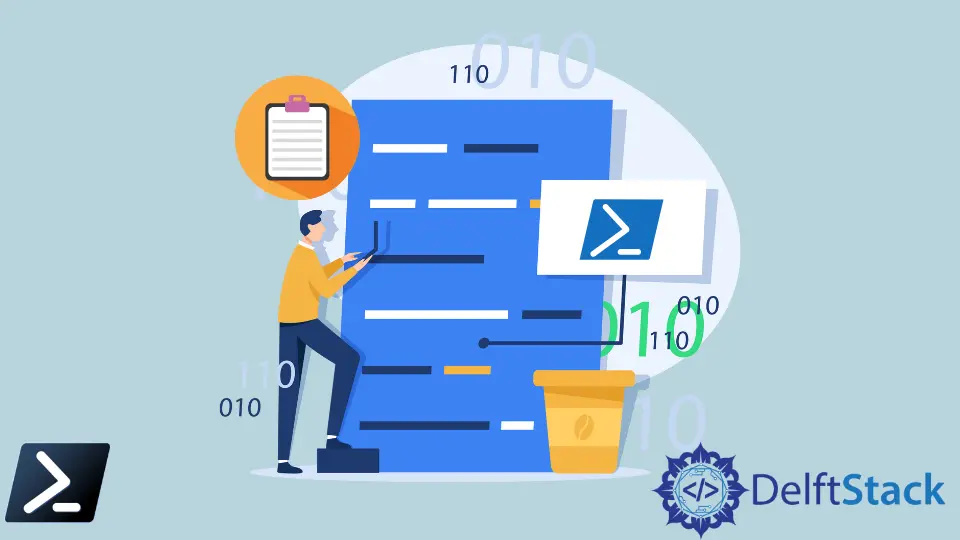
Using the clipboard in PowerShell can significantly enhance your productivity, especially when handling data. If you’ve ever used the familiar Ctrl+C keyboard shortcut to copy content, you might be wondering how to access that data programmatically. PowerShell provides a simple yet powerful command called Get-Clipboard
that allows you to retrieve whatever is currently stored in your clipboard.
This article will guide you through the steps to utilize this command effectively, enabling you to manipulate and use clipboard data seamlessly. Whether you’re a seasoned developer or a casual user, you will find practical applications for this feature in your daily tasks.
Understanding Get-Clipboard in PowerShell
The Get-Clipboard
command in PowerShell is designed to retrieve the contents of the clipboard. This command can be especially useful when you need to extract text or data that you’ve copied from various sources, such as web pages, documents, or even other scripts. By leveraging Get-Clipboard
, you can automate tasks that involve data entry, reporting, or even data transformation.
To use Get-Clipboard
, simply open your PowerShell terminal and type the command. Here’s how it works:
Get-Clipboard
Output:
This is the content of my clipboard.
When you execute this command, PowerShell returns the current clipboard contents. This can be text, images, or other formats, but for most practical applications, you’ll be dealing with text. Once you have the text, you can manipulate it further, save it to files, or even pass it to other commands for processing.
Storing Clipboard Data in a Variable
One of the most effective ways to use the output from Get-Clipboard
is by storing it in a variable. This allows you to reuse the clipboard data without needing to call Get-Clipboard
multiple times. Here’s how you can do it:
$clipboardData = Get-Clipboard
Output:
This is the content of my clipboard.
In this example, we assign the clipboard contents to the variable $clipboardData
. You can now use this variable in subsequent commands, making it easier to manipulate or analyze the data. For instance, if you want to count the number of words in the clipboard content, you can do the following:
$wordCount = ($clipboardData -split '\s+').Count
Write-Output "Word Count: $wordCount"
Output:
Word Count: 7
This command splits the clipboard text into an array of words and counts the number of elements in that array. The result is then displayed in the console. By storing clipboard data in a variable, you streamline your workflow and reduce redundancy in your code.
Manipulating Clipboard Data
Once you have the clipboard data in a variable, you can manipulate it in various ways. For example, you might want to modify the text before pasting it somewhere else. Here’s how you can convert all text to uppercase:
$uppercaseData = $clipboardData.ToUpper()
Set-Clipboard -Value $uppercaseData
Output:
THIS IS THE CONTENT OF MY CLIPBOARD.
In this code snippet, we first convert the clipboard text to uppercase using the ToUpper()
method. We then use the Set-Clipboard
command to update the clipboard with the modified text. This can be particularly useful if you need to format data consistently before pasting it into applications or documents.
Additionally, you can perform more complex transformations, such as replacing specific words or characters. For example, if you want to replace every instance of “clipboard” with “buffer,” you can do this:
$modifiedData = $clipboardData -replace 'clipboard', 'buffer'
Set-Clipboard -Value $modifiedData
Output:
This is the content of my buffer.
This command uses the -replace
operator to substitute text within the clipboard data. Such manipulations can save you time and effort, particularly when dealing with large volumes of text.
Exporting Clipboard Data to a File
Another practical application of Get-Clipboard
is exporting the clipboard contents to a file. This is especially useful for saving temporary data or logs. Here’s how you can write the clipboard data to a text file:
$clipboardData | Out-File -FilePath "C:\path\to\your\file.txt"
Output:
The clipboard content has been saved to the file.
In this example, we pipe the clipboard data to the Out-File
cmdlet, specifying the path where we want to save the file. This is a straightforward way to create a text file containing your clipboard contents. You can also specify additional parameters, such as -Encoding
to control the file format.
If you want to append data to an existing file instead of overwriting it, you can use the -Append
flag:
$clipboardData | Out-File -FilePath "C:\path\to\your\file.txt" -Append
Output:
The clipboard content has been appended to the file.
This method is particularly useful for logging purposes, where you may want to keep a running record of clipboard contents over time.
Conclusion
Using the Get-Clipboard
command in PowerShell opens up a world of possibilities for managing and manipulating clipboard data. Whether you’re storing clipboard content in variables, transforming it, or exporting it to files, PowerShell provides the tools you need to enhance your workflow. By mastering these techniques, you can automate repetitive tasks, streamline your data handling processes, and ultimately become more efficient in your daily activities. So, the next time you copy something to your clipboard, remember that PowerShell can help you make the most of that data.
FAQ
-
What is the purpose of the Get-Clipboard command in PowerShell?
Get-Clipboard retrieves the current contents of the clipboard, allowing you to use that data in your scripts. -
Can I use Get-Clipboard to access non-text data?
Yes, Get-Clipboard can access various formats, including images and files, but text is the most common use case.
-
How can I manipulate clipboard data in PowerShell?
You can store clipboard data in a variable and use string manipulation methods to modify it before using it elsewhere. -
Is it possible to export clipboard data to a file using PowerShell?
Absolutely! You can use the Out-File cmdlet to save clipboard contents to a specified file. -
Can I automate clipboard operations in PowerShell?
Yes, PowerShell scripts can automate clipboard operations, making it easier to handle repetitive tasks.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn