PowerShell ForEach Export-CSV
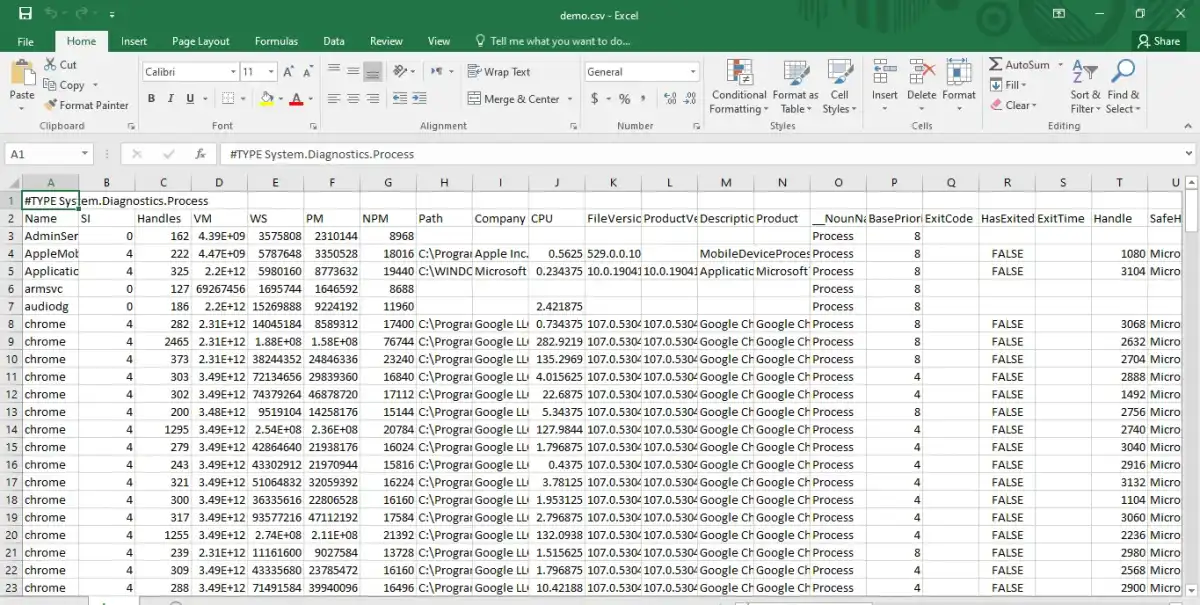
This tutorial demonstrates how to export CSV using the foreach
loop in PowerShell.
PowerShell ForEach Export-CSV
The Export-CSV
is used to export the given data to a CSV file using a PowerShell environment, where the Export-CSV
can be used in the foreach
to access each column of the CSV file to export the particular data.
The Export-CSV
will convert the PowerShell objects to the CSV strings and save them into the CSV file. The syntax for this command is:
<PowerShell Object> | Export-Csv [-Path] <string>
Where the PowerShell Object
is the object which will be exported to the CSV file, and the path
is the path of the CSV file. Let’s first try a simple example of using the Export-CSV
command before using it in the foreach
loop.
Get-Process | Export-Csv -Path "E:\Upwork\Programming article\Milestone 57\PowerShell\demo.csv"
The Get-Process
is used to get all the processes running, and we try to export this object to a CSV file. See the output:
Now let’s use the Export-CSV
in the foreach
loop to export data to particular columns. See the commands:
$Processes = Get-Process
$Processes | ForEach-Object {
$Process = [pscustomobject]@{
'Name' = $_.Name
'Handles' = $_.Handles
'Path' = $_.Path
'Company' = $_.Company
'Description' = $_.Description
'Product' = $_.Product
'SafeHandle' = $_.SafeHandle
}
$Process | Export-Csv "E:\Upwork\Programming article\Milestone 57\PowerShell\demo.csv" -Append -NoTypeInformation -Force
}
The above code will only export the Name
, Handles
, Path
, Company
, Description
, Product
, and SafeHandle
columns from the Get-Process
object to the CSV file. See the output:
Make sure when you run this command for the same file, the file should be closed.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook