How to Escape Special Characters in PowerShell
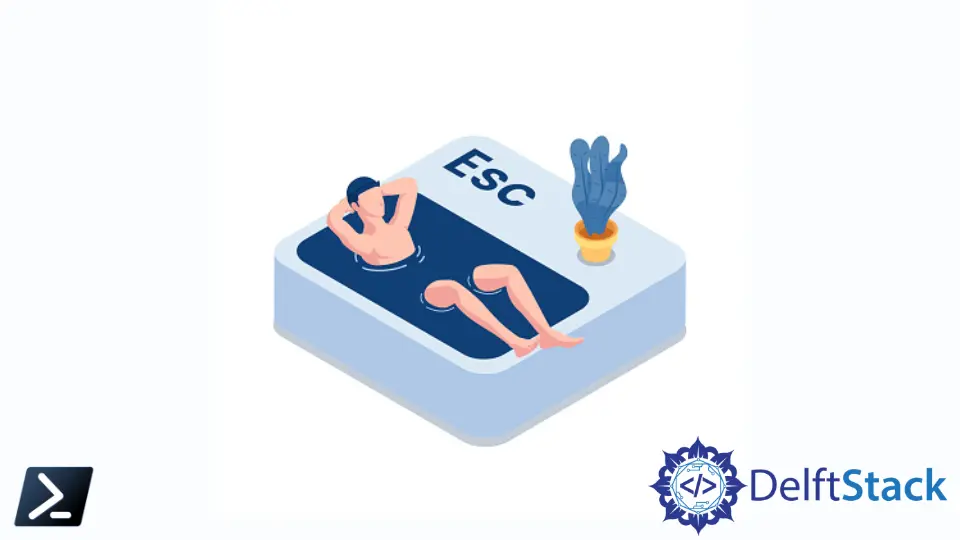
When working on PowerShell, you might need to escape special characters like *\~;(%?.:@/
. This tutorial will teach you to remove and escape such characters in PowerShell using the -Replace
operator.
Use the -Replace
Operator to Escape Special Characters in PowerShell
The -Replace
operator replaces texts or characters in PowerShell. You can use it to remove texts or characters from the string.
The -Replace
operator requires two arguments: the string to find and the string to replace from the given input.
This is our string variable containing special characters as follows.
$new = "p*o$#we%r"
To remove all special characters from the string, you can replace them with an empty string. The backslash \
escape character matches the character that is not recognized.
For example, \*
matches an asterisk *
.
$new = $new -replace "\*", ""
$new = $new -replace "\$", ""
$new = $new -replace "\#", ""
$new = $new -replace "%", ""
$new
Output:
power
The [RegEx]::Escape("input")
returns the escape codes that will help you to escape special characters in a string.
[regex]::Escape("p*o$#we%r")
Output:
p\*o\$\#we%r