How to Delete Folder in PowerShell
-
Use the
Remove-item
Cmdlet to Delete a Folder in PowerShell - Use Command Prompt to Delete a Folder in PowerShell
-
Use the
FileSystem
Object Method to Delete a Folder in PowerShell - Using .Net Class to Delete a Folder in PowerShell
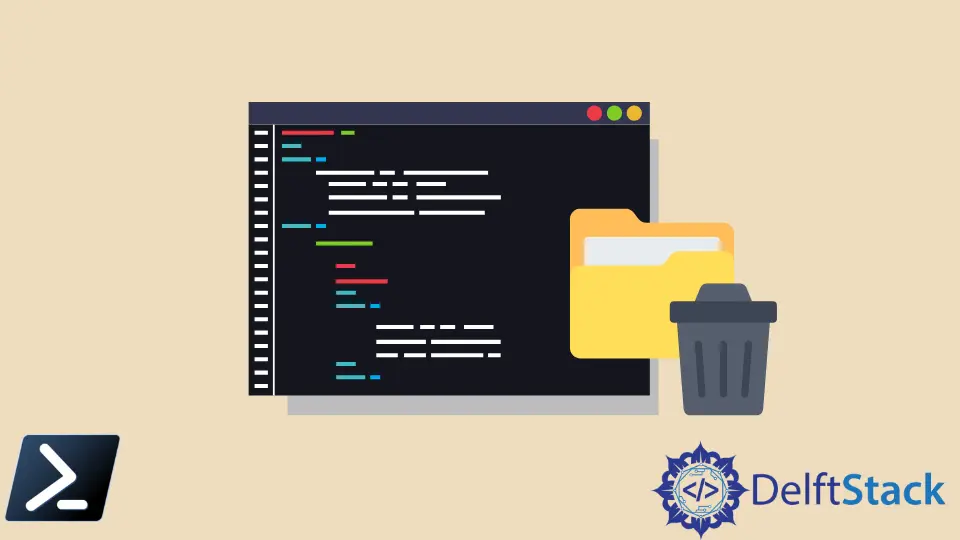
The folder delete action in PowerShell removes a folder from a given location, whether it is a local or shared path.
This article focuses on the different methods to delete a folder using PowerShell.
Use the Remove-item
Cmdlet to Delete a Folder in PowerShell
One or more objects can be removed with the Remove-Item
cmdlet.
We can use two different syntaxes for deletion. However, it should be noted that these two can be used separately but not combined.
- Syntax 1
Remove-Item
[-Path] <String[]>
[-Filter <String>]
[-Include <String[]>]
[-Exclude <String[]>]
[-Recurse]
[-Force]
[-Credential <PSCredential>]
[-WhatIf]
[-Confirm]
[-Stream <String[]>]
[<CommonParameters>]
- Syntax 2
Remove-Item
-LiteralPath <String[]>
[-Filter <String>]
[-Include <String[]>]
[-Exclude <String[]>]
[-Recurse]
[-Force]
[-Credential <PSCredential>]
[-WhatIf]
[-Confirm]
[-Stream <String[]>]
[<CommonParameters>]
Both -Path
and -Literalpath
cannot be used in the same syntax.
Remove-item
Parameters
The name and the description of the most common parameters used are given below.
Name | Description |
---|---|
-Path |
The location of the things to be removed is specified. There are no restrictions on the use of wildcard characters. |
-LiteralPath |
Path specified to one or more locations |
-Confirm |
Confirmation is prompted before running cmdlet |
-Exclude |
Items that the cmdlet excludes during the operation |
-Credential |
Any PowerShell-installed providers do not support this parameter. Use Invoke-Command to impersonate some other user or escalate your privileges when using this cmdlet. |
-Filter |
filter to validate path parameter |
-Force |
Force to remove objects that can’t be modified otherwise, such as private or read-only files/aliases or variables. |
-Include |
Items that cmdlet includes during the operation |
-Recurse |
This cmdlet discards the objects in the given locations, as well as all of the locations’ child items. |
-Stream |
The Stream argument is a dynamic parameter added to Remove-Item by the FileSystem provider. This setting is only applicable to drives with a file system. |
-WhatIf |
Shows what happens if the cmdlet is executed. |
Let’s look at some examples along with different syntax uses,
Example 1:
Here we will delete the test1
folder using the command mentioned below, and in the end, you can see that the folder will be deleted.
Remove-Item 'D:\temp\Test1'
Example 2:
We’ll recursively delete the folder test2. PowerShell checks to see if the directory is empty in the previous example. It will just remove the folder in this scenario.
Remove-Item 'D:\temp\test2' -Recurse
Example 3: Using -LiteralPath
in -RemoveItem
The -LiteralPath
is used along with the -Force
and -Recurse
parameters. So it forcefully deletes the items along with the folder in the given path without any confirmation prompts.
Remove-Item -LiteralPath "foldertodelete" -Force -Recurse
Example 4: Remove-item
as the pipeline
We first use the Get-ChildItem to retrieve the folders and files, and then we use the Remove-Item
to pipeline the results from the previous command.
Get-ChildItem C:\Temp\TestFolder\ | Remove-Item -Recurse -Force -Verbose
The only problem here is that it only deletes the contents of the folder but not the folder itself; therefore, other code snippets must be added to delete the folder.
Use Command Prompt to Delete a Folder in PowerShell
Most command-line users will use the rmdir
command to remove or delete folders. The syntax is rmdir
along with the folder path, as shown below.
rmdir C:\Temp\TestFolder
Use the FileSystem
Object Method to Delete a Folder in PowerShell
Different options for removing a folder are available in PowerShell. One of these is the File System Object Method. There are two phases to this procedure. In the first step, we’ll construct a file system object; next, in the second step, we’ll use the DeleteFolder()
function to destroy the associated object’s folder.
So to do this, first create a test.ps1
file and then add the following commands given below.
$object = New-Object -ComObject Scripting.FileSystemObject
$object.DeleteFolder("C:\Temp\TestFolder")
Finally, execute the test.ps1
file, and it will delete our intended folder.
Using .Net Class to Delete a Folder in PowerShell
In PowerShell, the System.IO.Directory
class and the Delete()
method are used by the.NET framework to delete a folder. If the supplied folder is not empty, this operation will throw an exception:
> [System.IO.Directory]::Delete("C:\Temp\TestFolder")
To remove this non-empty folder, use the $true
option in the Delete()
function:
System.IO.Directory]::Delete("C:\Temp\TestFolder", $true)
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.
Related Article - PowerShell Directory
- How to Get the Parent's Parent Directory in PowerShell
- PowerShell Equivalent of Linux mkdir Command
- How to Query Active Directory Users in PowerShell
- How to Filter Files and Folders Using PowerShell
- How to Get Only Directories Using Get Childitem in PowerShell
- How to Create Directory Using Windows PowerShell