PowerShell Alias With Parameters
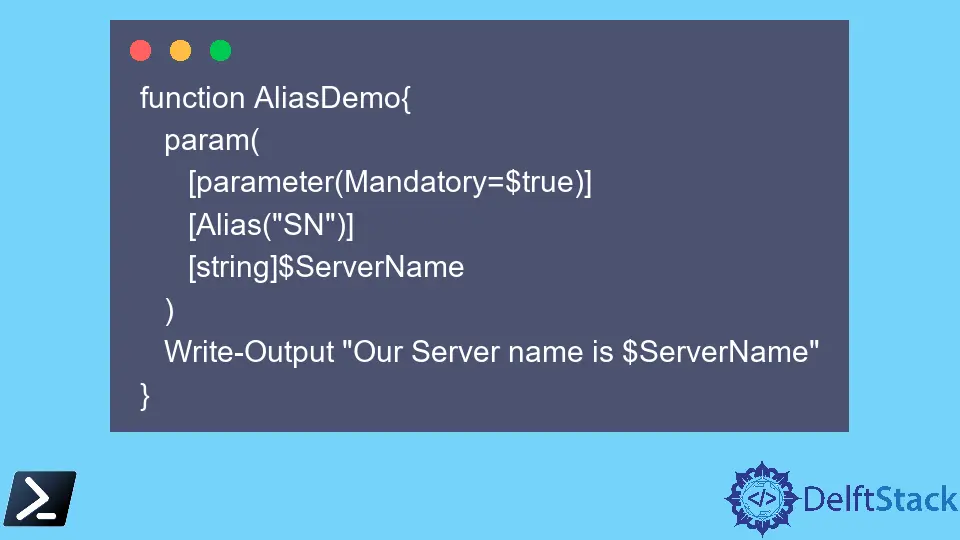
This tutorial demonstrates how to create an Alias
with parameters in PowerShell.
PowerShell Alias With Parameters
The PowerShell Alias
provides a shortcut name for the parameter
where we use the method Alias()
method to use the Alias
with parameters.
For example, if we want an Alias
for parameter authentication, we can use an Auth
alias; similarly, we can use AppID
for the application id, etc.
Let’s create an example using an Alias
with the parameters.
function AliasDemo{
param(
[parameter(Mandatory=$true)]
[Alias("SN")]
[string]$ServerName
)
Write-Output "Our Server name is $ServerName"
}
The code above creates an alias for the parameter ServerName
. Call the AliasDemo
function, and it will show the below output.
cmdlet AliasDemo at command pipeline position 1
Supply values for the following parameters:
ServerName: DelftstackServer
Our Server name is DelftstackServer
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook