How to Perform awk Commands in PowerShell
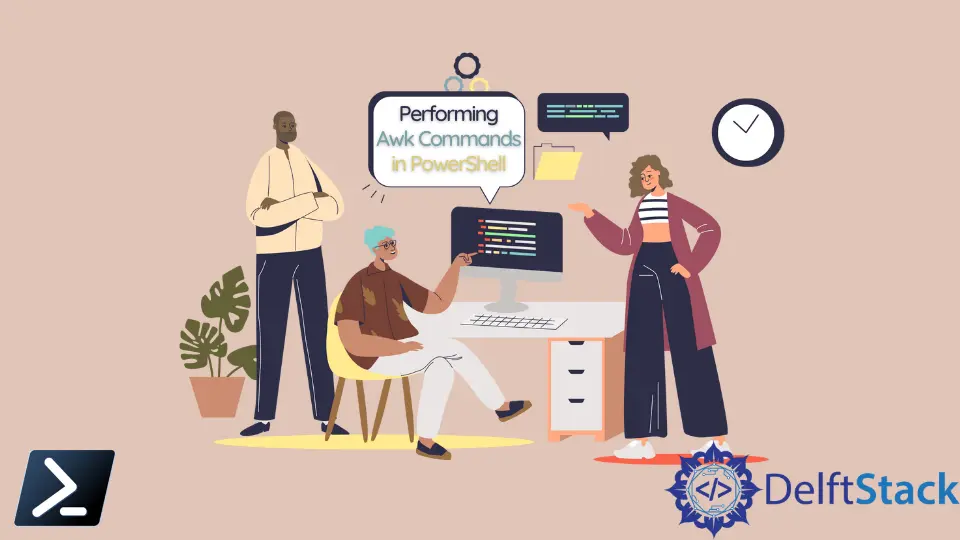
awk
is a scripting language for data manipulation and report generation. awk
is a command-line programming language that supports variables, text functions, numeric functions, and logical operators without compiling.
Unfortunately, awk
is not fully integrated into PowerShell since it uses the Windows operating system. This article will discuss how we can use similar commands and functions to the awk
commands using PowerShell.
Perform awk
Commands in PowerShell
The awk
utility allows programmers to create brief but powerful programs in the form of statements that specify text patterns, which may be strange to users unfamiliar with Linux commands or Linux in general. These text patterns are searched in each line, and action is taken when a match is found.
awk
is mainly used for pattern scanning and processing. It explores one or more files to see if they contain lines that match the specified patterns and then performs the associated actions.
The following code snippet is an example of a command that uses awk
.
awk '/"Box11"/ { print $0 }' test.txt|awk '{ SUM += $4} END { print SUM} '
To perform similar operations like the awk
commands, we would use the Get-Content
command to get the contents of a file and perform a couple of string operations in Windows PowerShell. If we will take the example above, this is what it would look like in PowerShell.
Example Code:
Get-Content c:\temp\test.txt |
Where-Object { $_ -match '"Box11"' } |
ForEach-Object { ($_ -split "\s+")[3] } |
Measure-Object -Sum |
Select-Object -ExpandProperty Sum
- The
Get-Content
command will get the contents of the text file. - The
Where-Object
command will filter the lines with theBox11
string. - Then, once filtered out, the script will loop to split each line string in its 4th element. Remember that PowerShell starts at index
0
; thus, we use index3
instead of4
, unlike in ourawk
command. - The
Measure-Object
will perform a calculation on the property, where it isSum
in this case. - Lastly, the
Select-Object
will output theSum
expanded property.
If we notice, our PowerShell version of the awk
command is slightly longer. Good thing that in PowerShell, we can create shortcuts by using aliases and a couple of PowerShell operators.
Example Code:
gc c:\temp\test.txt | ? { $_ -match '"Box11"' } | % { (-split $_)[3] } |
measure -Sum | select -Exp Sum
Alternatively, if you have WSL (Windows Subsystem for Linux) enabled, we can run awk
commands as it is. A feature of the Windows operating system called the Windows Subsystem for Linux (WSL) enables you to run a Linux file system, complete with Linux command-line tools and GUI applications, directly on Windows.
So we must call the wsl
command and perform awk
commands natively.
Example Code:
wsl -e awk '/"Box11"/{sum += $4} END{print sum}' test.txt
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn