How to Perform Keystroke Inside PowerShell
-
Use the
SendKeys
Method to Perform Keystrokes Inside PowerShell -
Use the
SendWait
Method to Perform Keystrokes Inside PowerShell
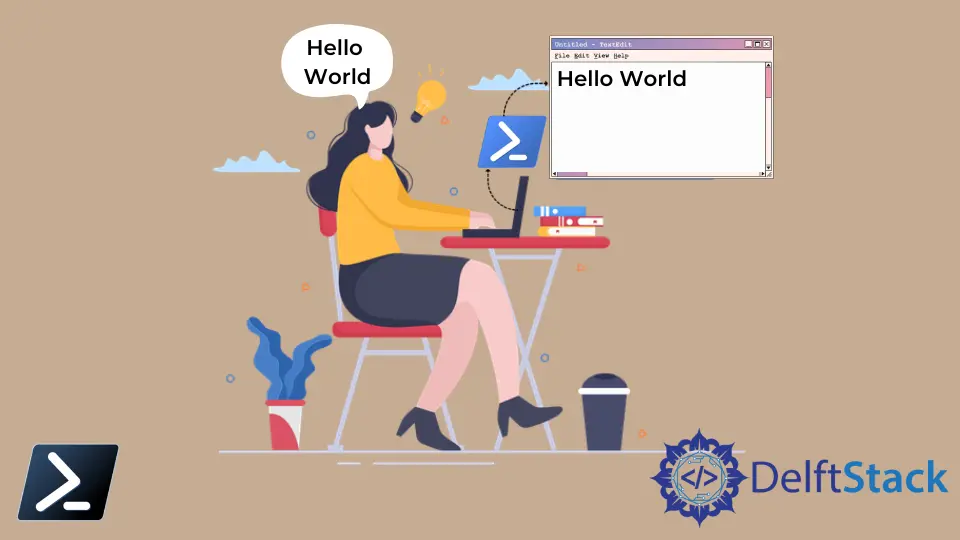
PowerShell is a powerful scripting language that can manage and automate different tasks in the system. It lets you send keystrokes from the console to the running application.
Use the SendKeys
Method to Perform Keystrokes Inside PowerShell
You can use the SendKeys()
method to send keystrokes to the active application.
The AppActivate()
method activates the application Notepad
so you can send keystrokes to it.
Command:
$wshell = New-Object -ComObject wscript.shell;
$wshell.AppActivate('Notepad')
$wshell.SendKeys('Hello World')
If you open the notepad and run the above code, the specified keys will be sent to the Notepad
.
Output:
Use the SendWait
Method to Perform Keystrokes Inside PowerShell
The SendWait()
method sends keystrokes to the active application and waits for the processes started by the keystrokes to be completed. It can be helpful when the other application needs to finish before the first one can proceed.
Command:
$wshell = New-Object -ComObject wscript.shell;
$wshell.AppActivate('Notepad')
[System.Windows.Forms.SendKeys]::SendWait('Hello~World')
The ~
character represents the Enter key. You can find codes to specify such action keys in this link.
Output:
The keystrokes are sent to the specified application after activating its window. We hope this tutorial helps you understand how to perform keystrokes inside PowerShell.