How to Jump Around to Certain Spots in PowerShell Script
-
Use a
while
Statement to Jump Around to Certain Spots in Script in PowerShell -
Use a
for
Statement to Jump Around to Certain Spots in Script in PowerShell -
Use
function
to Jump Around to Certain Spots in Script in PowerShell
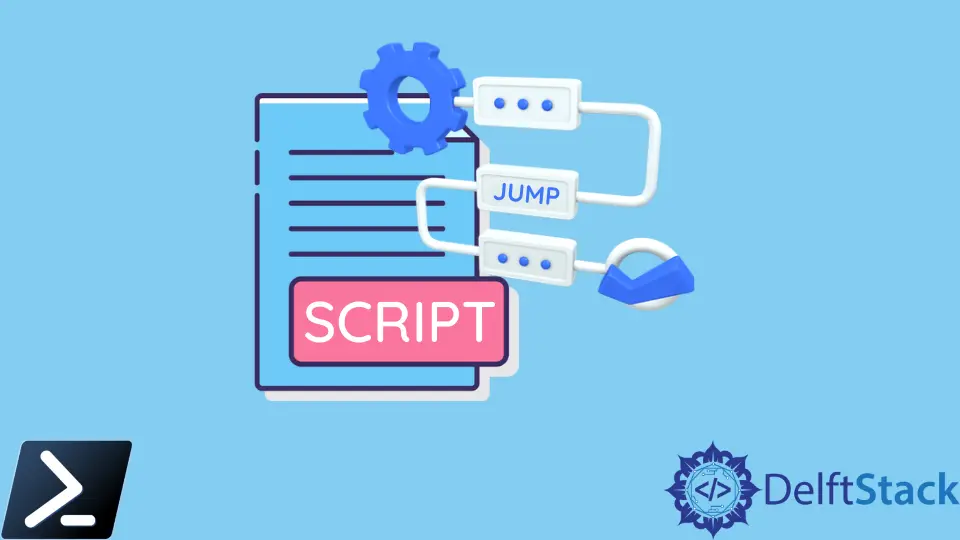
The GOTO
command jumps to a labeled line in a batch program. It directs command processing to a line specified in a label and provides the functionality of repeating lines of code.
PowerShell does not have the GOTO
command. Instead, you can use the loop statements for iteration or repeating lines of code.
This tutorial will teach you to jump around to certain spots in the PowerShell script.
Use a while
Statement to Jump Around to Certain Spots in Script in PowerShell
The while
statement runs commands in a block as long as a condition is true
. It means it will repeat lines of code when the condition is true
.
Example Code:
$a = 1
while ($a -lt 10) {
$a
$a++
}
The first command assigns the value 1
to the variable $a
. Inside the while block, the value of $a
is printed.
The condition $a is not less than 10
is true
, and the value of $a
is incremented by 1 each iteration. When the value of $a
reaches 10
, the conditional statement becomes false
, and the loop is terminated.
As a result, it prints the number from 1 to 9.
Output:
1
2
3
4
5
6
7
8
9
You can create a while
loop in your script that executes when the condition is true
. If the condition is false
, it will be skipped and make a script jump to the next spot.
Use a for
Statement to Jump Around to Certain Spots in Script in PowerShell
The for
statement or for
loop runs commands in a block based on the condition. The commands in the loop continue to run until the condition becomes false
.
In the following example, the condition $b is less than 6
evaluates to true
, and the command in a for
loop runs until the condition becomes false
when it equals 6.
Example Code:
for ($b = 1; $b -lt 6; $b++) {
Write-Host $b
}
Output:
1
2
3
4
5
Use function
to Jump Around to Certain Spots in Script in PowerShell
Another alternative option is to wrap the block of commands in a function. If the value of $continue
equals y
, the condition becomes true
in the following example.
As a result, it runs the first command in the if
statement.
Example Code:
function Choice {
$continue = Read-Host "Do you want to continue?"
if ($continue -eq 'y') {
Write-Host "Welcome to PowerShell tutorials."
}
else {
Write-Host "Exited."
}
}
Choice
Output:
Do you want to continue?: y
Welcome to PowerShell tutorials.
If the value of $continue
is not equal to y
, the condition becomes false
, and the second command gets executed.
Output:
Do you want to continue?: n
Exited.
This way, you can easily make a script jump to a specific spot in PowerShell.