How to Import Text File and Format and Export It to CSV in PowerShell
- Importing a Text File with Get-Content
- Formatting the Data
- Exporting to CSV with Export-Csv
- Conclusion
- FAQ
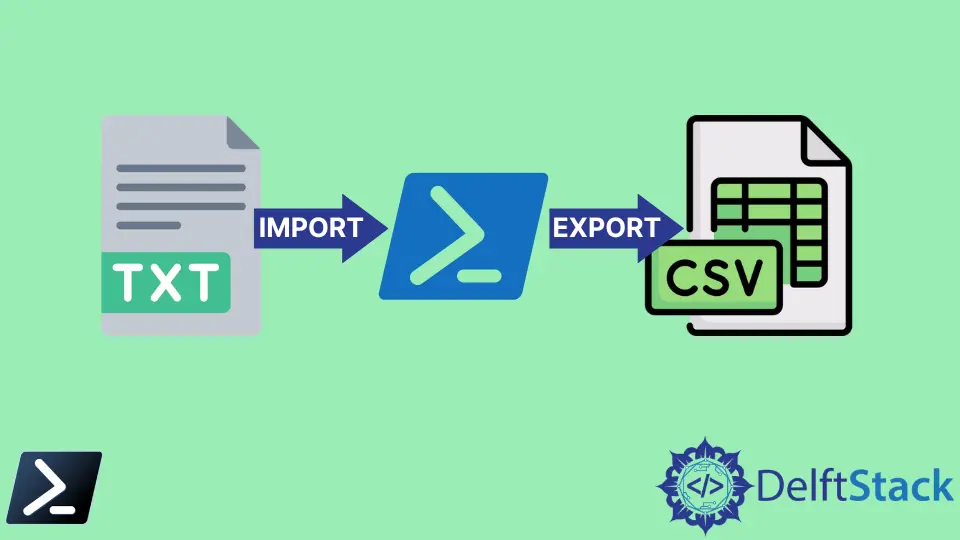
PowerShell is a powerful tool for managing and automating tasks in Windows. One common requirement is to import data from a text file, format it, and then export it to a CSV file. This process can be incredibly useful for data analysis, reporting, or even just organizing information.
In this article, we will walk you through the steps needed to achieve this using PowerShell commands like Get-Content, ConvertFrom-StringData, and Export-Csv. Whether you are a beginner or an experienced user, you’ll find these techniques straightforward and efficient for handling your data transformation tasks.
Importing a Text File with Get-Content
The first step in our process is to import the content of a text file using the Get-Content cmdlet. This command reads the content of a file and allows us to manipulate it as needed. Here’s how you can do it:
$content = Get-Content -Path "C:\path\to\your\file.txt"
Output:
Line 1: Data1, Data2, Data3
Line 2: Data4, Data5, Data6
In this example, we are using the Get-Content cmdlet to read a text file located at “C:\path\to\your\file.txt”. The content of the file is stored in the $content
variable. Each line of the text file will be treated as an element in an array, making it easy to manipulate later. This command is essential for bringing your data into PowerShell, setting the stage for formatting and exporting it.
Formatting the Data
Once we have the data imported, the next step is to format it appropriately. Depending on the structure of your text file, you might need to split the data into separate fields. This can be done using the -split
operator. Here’s an example of how to format our imported data:
$formattedData = $content | ForEach-Object {
$fields = $_ -split ", "
[PSCustomObject]@{
Column1 = $fields[0]
Column2 = $fields[1]
Column3 = $fields[2]
}
}
Output:
Column1 Column2 Column3
-------- -------- --------
Data1 Data2 Data3
Data4 Data5 Data6
In this code snippet, we use a ForEach-Object
loop to iterate through each line of the imported content. The -split
operator divides each line into an array of fields based on the comma and space delimiter. We then create a custom object for each line, mapping the fields to meaningful property names. This structured approach makes it easier to work with the data in a tabular format, preparing it for the final export.
Exporting to CSV with Export-Csv
The final step in our process is to export the formatted data to a CSV file using the Export-Csv cmdlet. This cmdlet allows you to easily save your data in a format that can be opened in spreadsheet applications like Microsoft Excel. Here’s how to do it:
$formattedData | Export-Csv -Path "C:\path\to\your\output.csv" -NoTypeInformation
Output:
"Column1","Column2","Column3"
"Data1","Data2","Data3"
"Data4","Data5","Data6"
In this command, we pipe the $formattedData
to the Export-Csv cmdlet, specifying the path where we want to save our CSV file. The -NoTypeInformation
parameter is used to prevent PowerShell from adding type information to the first line of the CSV file, keeping it clean and easy to read. After executing this command, you will have a neatly formatted CSV file ready for use.
Conclusion
In summary, importing a text file, formatting the data, and exporting it to a CSV file in PowerShell is a straightforward process that can save you time and effort. By leveraging cmdlets like Get-Content, ForEach-Object, and Export-Csv, you can easily transform your data into a usable format. Whether you’re working on data analysis, reporting, or simple organization tasks, these PowerShell techniques will enhance your productivity and streamline your workflows.
FAQ
-
How do I install PowerShell?
You can install PowerShell by downloading it from the official Microsoft website or using a package manager like Chocolatey. -
Can I use PowerShell on Linux?
Yes, PowerShell is cross-platform and can be installed on Linux and macOS. -
What is the difference between CSV and Excel files?
CSV files are plain text files that use commas to separate values, while Excel files are binary files that can contain multiple sheets and complex formatting. -
Can I automate this process with a script?
Absolutely! You can save the PowerShell commands in a script file (.ps1) and run it to automate the import, format, and export process.
- Is PowerShell suitable for large datasets?
Yes, PowerShell can handle large datasets efficiently, but performance may vary based on system resources and the complexity of operations.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn